in java I need help with making a preorder traversal method in a binary search tree, It uses recursion and the code is down below private void preorder(BSTNode current, ArrayList preorderKeys) { if (current == null) { return; } preorderKeys.add(current.getKey()); preorderKeys.add((E) current.getLeftChild()); preorderKeys.add((E) current.getRightChild()); } its supposed to look something like this preorder(node): if node is null: return visit node preorder(node.left) preorder(node.right)
in java I need help with making a preorder traversal method in a binary search tree, It uses recursion and the code is down below private void preorder(BSTNode current, ArrayList preorderKeys) { if (current == null) { return; } preorderKeys.add(current.getKey()); preorderKeys.add((E) current.getLeftChild()); preorderKeys.add((E) current.getRightChild()); } its supposed to look something like this preorder(node): if node is null: return visit node preorder(node.left) preorder(node.right)
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
in java
I need help with making a preorder traversal method in a binary search tree, It uses recursion and the code is down below
private void preorder(BSTNode<E> current, ArrayList<E> preorderKeys) {
if (current == null) {
return;
}
preorderKeys.add(current.getKey());
preorderKeys.add((E) current.getLeftChild());
preorderKeys.add((E) current.getRightChild());
}
its supposed to look something like this
preorder(node):
if node is null: return
visit node
preorder(node.left)
preorder(node.right)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-engineering and related others by exploring similar questions and additional content below.Recommended textbooks for you
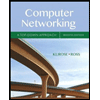
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
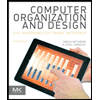
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
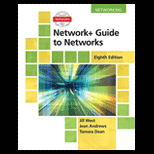
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
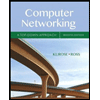
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
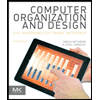
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
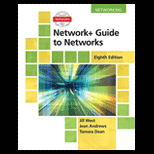
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
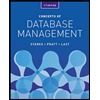
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
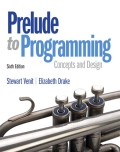
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
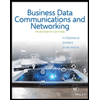
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY