In Java please. Reimplement the TrafficLight class using a simple counter that is advanced in each call to next. If the traffic light was initially green, the counter has values 0 1 2 3 4 5 6 … . If the traffic light was initially red, the counter has values 2 3 4 5 6 7 8 … . Compute the current color and the number of reds, using integer division and remainder. Help me with this code (fill in /* Your code goes here */): /** A simulated traffic light. */ public class TrafficLight { private int steps; /** Constructs a green traffic light. */ public TrafficLight() { /* Your code goes here */ } /** Constructs a traffic light. @param initialColor the initial color "green", "yellow", or "red" */ public TrafficLight(String initialColor) { /* Your code goes here */ } /** Moves this traffic light to the next color. */ public void next() { steps++; } /** Returns the current color of this traffic light. @return the current color */ public String getColor() { /* Your code goes here */ } /** Counts how often this traffic light has been red. @return the number of times this traffic light has been red */ public int getReds() { /* Your code goes here */ } } Second part of code that was GIVEN do not modify public class TrafficLightTester { public static void main(String[] args) { TrafficLight tl1 = new TrafficLight(); System.out.println(tl1.getColor()); System.out.println("Expected: green"); System.out.println(tl1.getReds()); System.out.println("Expected: 0"); tl1.next(); System.out.println(tl1.getColor()); System.out.println("Expected: yellow"); System.out.println(tl1.getReds()); System.out.println("Expected: 0"); tl1.next(); System.out.println(tl1.getColor()); System.out.println("Expected: red"); System.out.println(tl1.getReds()); System.out.println("Expected: 1"); tl1.next(); System.out.println(tl1.getColor()); System.out.println("Expected: green"); System.out.println(tl1.getReds()); System.out.println("Expected: 1"); TrafficLight tl2 = new TrafficLight("red"); System.out.println(tl2.getColor()); System.out.println("Expected: red"); System.out.println(tl2.getReds()); System.out.println("Expected: 1"); tl2.next(); System.out.println(tl2.getColor()); System.out.println("Expected: green"); System.out.println(tl2.getReds()); System.out.println("Expected: 1"); tl2.next(); tl2.next(); System.out.println(tl2.getColor()); System.out.println("Expected: red"); System.out.println(tl2.getReds()); System.out.println("Expected: 2"); }
In Java please.
Reimplement the TrafficLight class using a simple counter that is advanced in each call to next. If the traffic light was initially green, the counter has values 0 1 2 3 4 5 6 … . If the traffic light was initially red, the counter has values 2 3 4 5 6 7 8 … . Compute the current color and the number of reds, using integer division and remainder.
Help me with this code (fill in /* Your code goes here */):
/**
A simulated traffic light.
*/
public class TrafficLight
{
private int steps;
/**
Constructs a green traffic light.
*/
public TrafficLight()
{
/* Your code goes here */
}
/**
Constructs a traffic light.
@param initialColor the initial color "green", "yellow", or "red"
*/
public TrafficLight(String initialColor)
{
/* Your code goes here */
}
/**
Moves this traffic light to the next color.
*/
public void next()
{
steps++;
}
/**
Returns the current color of this traffic light.
@return the current color
*/
public String getColor()
{
/* Your code goes here */
}
/**
Counts how often this traffic light has been red.
@return the number of times this traffic light has been red
*/
public int getReds()
{
/* Your code goes here */
}
}
Second part of code that was GIVEN do not modify
public class TrafficLightTester
{
public static void main(String[] args)
{
TrafficLight tl1 = new TrafficLight();
System.out.println(tl1.getColor());
System.out.println("Expected: green");
System.out.println(tl1.getReds());
System.out.println("Expected: 0");
tl1.next();
System.out.println(tl1.getColor());
System.out.println("Expected: yellow");
System.out.println(tl1.getReds());
System.out.println("Expected: 0");
tl1.next();
System.out.println(tl1.getColor());
System.out.println("Expected: red");
System.out.println(tl1.getReds());
System.out.println("Expected: 1");
tl1.next();
System.out.println(tl1.getColor());
System.out.println("Expected: green");
System.out.println(tl1.getReds());
System.out.println("Expected: 1");
TrafficLight tl2 = new TrafficLight("red");
System.out.println(tl2.getColor());
System.out.println("Expected: red");
System.out.println(tl2.getReds());
System.out.println("Expected: 1");
tl2.next();
System.out.println(tl2.getColor());
System.out.println("Expected: green");
System.out.println(tl2.getReds());
System.out.println("Expected: 1");
tl2.next();
tl2.next();
System.out.println(tl2.getColor());
System.out.println("Expected: red");
System.out.println(tl2.getReds());
System.out.println("Expected: 2");
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

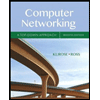
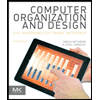
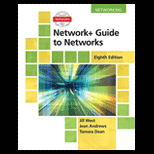
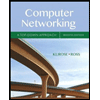
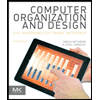
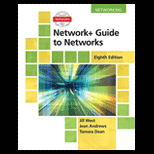
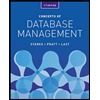
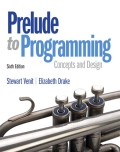
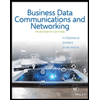