In Java Write a Fraction class that implements these methods: • add ─ This method receives a Fraction parameter and adds the parameter fraction to the calling object fraction. • multiply ─ This method receives a Fraction parameter and multiplies the parameter fraction by the calling object fraction. • print ─ This method prints the fraction using fraction notation (1/4, 21/14, etc.) • printAsDouble ─ This method prints the fraction as a double (0.25, 1.5, etc.) • Separate accessor methods for each instance variable (numerator , denominator ) in the Fraction class Provide a driver class (FractionDemo) that demonstrates this Fraction class. The driver class should contain this main method : public static void main(String[] args) { Scanner stdIn = new Scanner(System.in); Fraction c, d, x; // Fraction objects System.out.println("Enter numerator; then denominator."); c = new Fraction(stdIn.nextInt(), stdIn.nextInt()); c.print(); System.out.println("Enter numerator; then denominator."); d = new Fraction(stdIn.nextInt(), stdIn.nextInt()); d.print(); x = new Fraction(); // create a fraction for number 0 System.out.println("Sum:"); x.add(c).add(d); x.print(); x.printAsDouble(); x = new Fraction(1, 1); // create a fraction for number 1 System.out.println("Product:"); x.multiply(c).multiply(d); x.print(); x.printAsDouble(); System.out.println("Enter numerator; then denominator."); x = new Fraction(stdIn.nextInt(), stdIn.nextInt()); x.printAsDouble(); } // end main Please study this driver carefully. Note that this driver does not call the accessor methods--that’s OK. Accessor methods are often implemented regardless of whether there is an immediate need; they are handy methods in general, and providing them means that future code can use them when needed. Sample session: Enter numerator; then denominator. 5 8 5/8 Enter numerator; then denominator. 4 10 4/10 Sum: 82/80 1.025 Product: 20/80 0.25 Enter numerator; then denominator. 6 0 infinity
In Java
Write a Fraction class that implements these methods:
• add ─ This method receives a Fraction parameter and adds the parameter fraction to the
calling object fraction.
• multiply ─ This method receives a Fraction parameter and multiplies the parameter
fraction by the calling object fraction.
• print ─ This method prints the fraction using fraction notation (1/4, 21/14, etc.)
• printAsDouble ─ This method prints the fraction as a double (0.25, 1.5, etc.)
• Separate accessor methods for each instance variable (numerator , denominator ) in the
Fraction class
Provide a driver class (FractionDemo) that demonstrates this Fraction class. The driver class should
contain this main method :
public static void main(String[] args)
{
Scanner stdIn = new Scanner(System.in);
Fraction c, d, x; // Fraction objects
System.out.println("Enter numerator; then denominator.");
c = new Fraction(stdIn.nextInt(), stdIn.nextInt());
c.print();
System.out.println("Enter numerator; then denominator.");
d = new Fraction(stdIn.nextInt(), stdIn.nextInt());
d.print();
x = new Fraction(); // create a fraction for number 0
System.out.println("Sum:");
x.add(c).add(d);
x.print();
x.printAsDouble();
x = new Fraction(1, 1); // create a fraction for number 1
System.out.println("Product:");
x.multiply(c).multiply(d);
x.print();
x.printAsDouble();
System.out.println("Enter numerator; then denominator.");
x = new Fraction(stdIn.nextInt(), stdIn.nextInt());
x.printAsDouble();
} // end main
Please study this driver carefully. Note that this driver does not call the accessor methods--that’s OK.
Accessor methods are often implemented regardless of whether there is an immediate need; they are
handy methods in general, and providing them means that future code can use them when needed.
Sample session:
Enter numerator; then denominator.
5
8
5/8
Enter numerator; then denominator.
4
10
4/10
Sum:
82/80
1.025
Product:
20/80
0.25
Enter numerator; then denominator.
6
0
infinity

Step by step
Solved in 4 steps

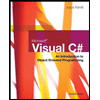
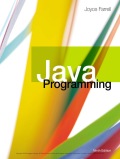
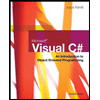
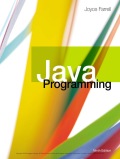