In main, declare an array of size 20 and name it "randomArray." Use the function in step 2 to fill the array. Use the function in step 3 to print the array. Create a function that generates 20 random integers with a range of 1 to 10 and places them into an array. Re-cycle the functions from Lab 10 where appropriate.
In main, declare an array of size 20 and name it "randomArray." Use the function in step 2 to fill the array. Use the function in step 3 to print the array. Create a function that generates 20 random integers with a range of 1 to 10 and places them into an array. Re-cycle the functions from Lab 10 where appropriate.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter8: Arrays And Strings
Section: Chapter Questions
Problem 23PE
Related questions
Question
(C++) PLEASE INCLUDE COMMENTS AND OUTPUT SCREEN
Write a
- In main, declare an array of size 20 and name it "randomArray." Use the function in step 2 to fill the array. Use the function in step 3 to print the array.
- Create a function that generates 20 random integers with a range of 1 to 10 and places them into an array. Re-cycle the functions from Lab 10 where appropriate.
- Make this a function.
- There will be two arguments in the parameter list of this function: an array and the size of the array.
- Within the function and the function prototype name the array: intArray.
- Within the function and the function prototype name the size of the array: size.
- The data type of the function will be void since the array will be sent back through the parameter list.
- Bring in the function that generates and returns a random number that you created from the previous module. Call that function from this within the loop that adds random numbers to the array.
- Display the contents of the array. Re-cycle the function that prints out the contents of an integer array from Lab 10.
- Make this a function.
- There will be two arguments in the parameter list of this function: an array and the size of the array.
- Within the function and the function prototype name the array: intArray.
- Within the function and the function prototype name the size of the array: size.
- The data type of the function will be void since the array will be sent back through the parameter list.
- From main, generate one more random number (also from 1 to 10) from the random number function. Do not put this in an array. This is a stand alone variable that contains one random number.
- Search though the array and count how many times the extra random number occurs. It is possible that the extra random number may occur more than once or not at all.
Output:
- Display the entire array.
- Display the extra random number.
- Depending upon the result of your search, display one of the following:
– How many times the random number occurs.
– That the random number did not occur at all.
Also include:
- Use a sentinel driven outer While Loop to repeat the task
- Ask the User if they wish to generate a new set of random numbers
- Clear the previous list of numbers from the output screen before displaying the new set.
NOTE 1: Other than the prompt to repeat the task, there is no input from the User in this program.
NOTE 2: This program will have 3 functions:
- The function that fills the array with random numbers.
- The function that generates one random number at a time (re-use the one from Lab 10).
- The function that prints out an array of integers (re-use the one from Lab 10).
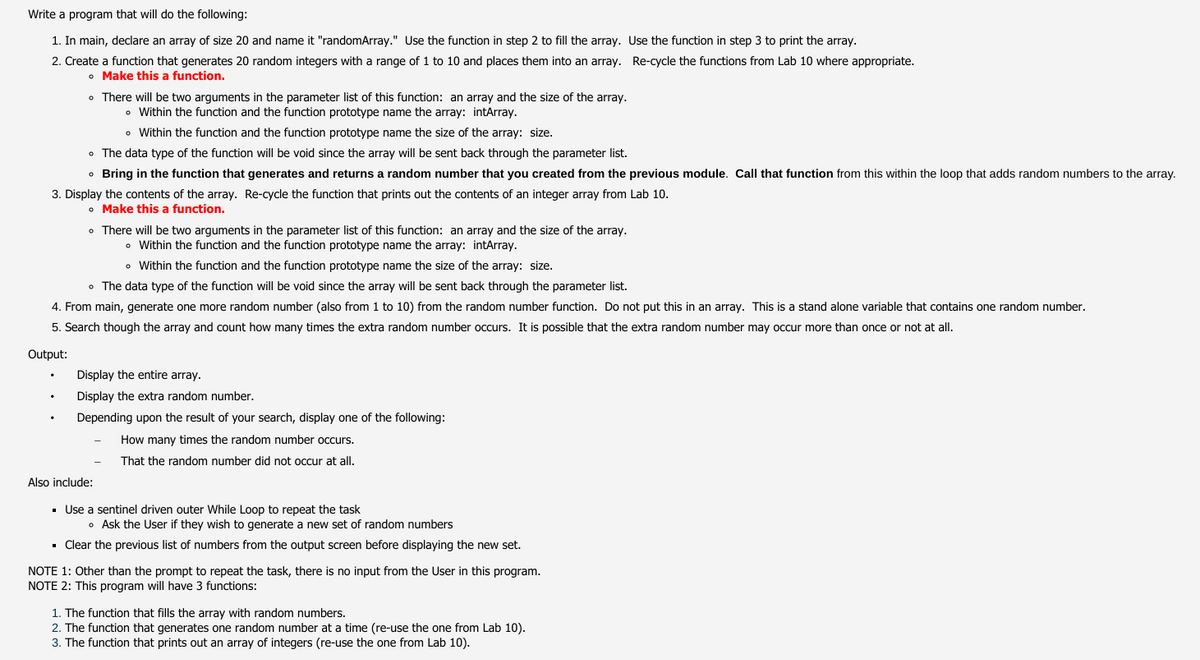
Transcribed Image Text:Write a program that will do the following:
1. In main, declare an array of size 20 and name it "randomArray." Use the function in step 2 to fill the array. Use the function in step 3 to print the array.
2. Create a function that generates 20 random integers with a range of 1 to 10 and places them into an array. Re-cycle the functions from Lab 10 where appropriate.
• Make this a function.
• There will be two arguments in the parameter list of this function: an array and the size of the array.
• Within the function and the function prototype name the array: intArray.
• Within the function and the function prototype name the size of the array: size.
• The data type of the function will be void since the array will be sent back through the parameter list.
• Bring in the function that generates and returns a random number that you created from the previous module. Call that function from this within the loop that adds random numbers to the array.
3. Display the contents of the array. Re-cycle the function that prints out the contents of an integer array from Lab 10.
• Make this a function.
• There will be two arguments in the parameter list of this function: an array and the size of the array.
• Within the function and the function prototype name the array: intArray.
• Within the function and the function prototype name the size of the array: size.
• The data type of the function will be void since the array will be sent back through the parameter list.
4. From main, generate one more random number (also from 1 to 10) from the random number function. Do not put this in an array. This is a stand alone variable that contains one random number.
5. Search though the array and count how many times the extra random number occurs. It is possible that the extra random number may occur more than once or not at all.
Output:
Display the entire array.
Display the extra random number.
Depending upon the result of your search, display one of the following:
How many times the random number occurs.
That the random number did not occur at all.
Also include:
• Use a sentinel driven outer While Loop to repeat the task
• Ask the User if they wish to generate a new set of random numbers
· Clear the previous list of numbers from the output screen before displaying the new set.
NOTE 1: Other than the prompt to repeat the task, there is no input from the User in this program.
NOTE 2: This program will have 3 functions:
1. The function that fills the array with random numbers.
2. The function that generates one random number at a time (re-use the one from Lab 10).
3. The function that prints out an array of integers (re-use the one from Lab 10).
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
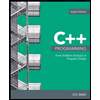
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
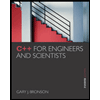
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
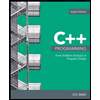
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
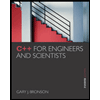
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage