In Python - I got the menu set up and repeating for multiple users. I'm not sure how to do the accumulators so that I can tally up total users and get the averages. Thanks Problem #1: How much should I study outside of class? Your fellow students need help. This is their first year in college and they need to determine how many hours they need to study to get good grades. Study Hours per Week per Class Grade 15 A 12 B 9 C 6 D 0 F Project Specifications: The menu driven program has the following options: Determine Hours to Study The user enters their full name and the number of credits they are taking. The user will then enter the grade they want, assume the same grade for all classes. The program determines the number of hours they have to study, assume they will study the same number of hours for each class. The program displays the user’s name in proper case, number of credits, expected total number of weekly study hours, and desired grade The information from 2.4 is also appended to a file named txt. Determine Grade The user enters their full name and the number of credits they are taking. The user will then enter the number of study hours they have to study per week. Hint: Make sure it’s reasonable, you can’t study more than the number of hours within a week. The program determines the grade they should earn, assume they will receive the same grade in each class All data should be proper case and displayed as proper case The information from 3.5 is also appended to a file named txt Display Total At the end of the program, the program displays the total number of students who used the program, the average credits taken, and the average study hours. In the following format – Total Students: 3 Average Credits: 9 Average Study Hours: 20 Terminate - program is terminated def welcome(): print('Welcome to the Study Time Calculator\n'+ 'By Casie West\n\n'+ 'This program calculates the number of hours needed\n'+ 'to study to get the letter grade desired.\n\n'+ 'At the end of the program it tallies the number of students\n'+ 'and shows the average course credits and study hours calculated.\n\n') def menuOption(): option = input("Select one of the following options: \n"\ "A. How many hours do you need to study? \n"\ "B. What grade will you earn if you study this many hours? \n"\ "C. Total number of students and average hours: \n"\ "D. Goodbye. \n") option = validate(option) return option def validate(selection): while selection.isdigit() or (selection.upper() != "A" and selection.upper() != "B" \ and selection.upper() != "C" and selection.upper() != "D"): print('Not a valid menu option.') selection = input("Select one of the following options: \n"\ "A. How many hours do you need to study? \n"\ "B. What grade will you earn if you study this many hours? \n"\ "C. Total number of students and average hours: \n"\ "D. Goodbye. \n") return selection.upper() def Main(): welcome() cont = True while cont: choice = menuOption() if choice == 'A': studyHours() elif choice == 'B': grade() elif choice == 'C': total() elif choice == 'D': closeProgram() cont = False def studyHours(): hoursFile = open('StudentsHoursGrades.txt', 'w') student = input('Please enter your first and last name: \n') #Validation that something was entered while student == "": print('Please print a valid name. \n') student = input('Please enter your first and last name: \n') creditHours = input('How many credit hours are you taking this semester? \n') #Validate user entered a number while not creditHours.isdigit() or int(creditHours)<0 or int(creditHours) >=19: print('You entered', creditHours,'Please enter a the number of credit hours you are taking.') creditHours = input('How many credit hours are you taking this semester? \n') grade = input('Please enter what letter grade you want to earn: \n') while not((grade >= 'a' and grade <='d') or (grade == 'f') or (grade >='A' and grade <= 'D') or (grade =='F')): print('Please enter a valid letter grade of A, B, C, D, or F.') grade = input('Please enter what letter grade you want to earn: \n') if grade.upper() == 'A': studyRate = 15 elif grade.upper() == 'B': studyRate = 12 elif grade.upper() == 'C': studyRate = 9 elif grade.upper() == 'D': studyRate = 6 elif grade.upper() == 'F': studyRate = 0 classHours = int(creditHours)/3 hours = int(classHours) * studyRate hoursFile.write(student + '\n') hoursFile.write(str(creditHours) + '\n') hoursFile.write(str(hours) + '\n') hoursFile.write(grade + '\n') hoursFile.close() print('Name: ',student) print('Credits: ',creditHours) print('Study Hours: ',hours) print('Letter Grade: ',grade) def grade(): hoursFile = open('StudentsHoursGrades.txt', 'a') def closeProgram(): print('\nThank you for using the study time calculator.'\ 'Have a great day!') Main()
In Python - I got the menu set up and repeating for multiple users. I'm not sure how to do the accumulators so that I can tally up total users and get the averages. Thanks
Problem #1: How much should I study outside of class?
Your fellow students need help. This is their first year in college and they need to determine how many hours they need to study to get good grades.
Study Hours per Week per Class Grade
15 A
12 B
9 C
6 D
0 F
Project Specifications:
- The menu driven
program has the following options: - Determine Hours to Study
- The user enters their full name and the number of credits they are taking.
- The user will then enter the grade they want, assume the same grade for all classes.
- The program determines the number of hours they have to study, assume they will study the same number of hours for each class.
- The program displays the user’s name in proper case, number of credits, expected total number of weekly study hours, and desired grade
- The information from 2.4 is also appended to a file named txt.
- Determine Grade
- The user enters their full name and the number of credits they are taking.
- The user will then enter the number of study hours they have to study per week. Hint: Make sure it’s reasonable, you can’t study more than the number of hours within a week.
- The program determines the grade they should earn, assume they will receive the same grade in each class
- All data should be proper case and displayed as proper case
- The information from 3.5 is also appended to a file named txt
- Display Total
-
- At the end of the program, the program displays the total number of students who used the program, the average credits taken, and the average study hours. In the following format –
Total Students: 3
Average Credits: 9
Average Study Hours: 20
-
- Terminate - program is terminated
def welcome():
print('Welcome to the Study Time Calculator\n'+
'By Casie West\n\n'+
'This program calculates the number of hours needed\n'+
'to study to get the letter grade desired.\n\n'+
'At the end of the program it tallies the number of students\n'+
'and shows the average course credits and study hours calculated.\n\n')
def menuOption():
option = input("Select one of the following options: \n"\
"A. How many hours do you need to study? \n"\
"B. What grade will you earn if you study this many hours? \n"\
"C. Total number of students and average hours: \n"\
"D. Goodbye. \n")
option = validate(option)
return option
def validate(selection):
while selection.isdigit() or (selection.upper() != "A" and selection.upper() != "B" \
and selection.upper() != "C" and selection.upper() != "D"):
print('Not a valid menu option.')
selection = input("Select one of the following options: \n"\
"A. How many hours do you need to study? \n"\
"B. What grade will you earn if you study this many hours? \n"\
"C. Total number of students and average hours: \n"\
"D. Goodbye. \n")
return selection.upper()
def Main():
welcome()
cont = True
while cont:
choice = menuOption()
if choice == 'A':
studyHours()
elif choice == 'B':
grade()
elif choice == 'C':
total()
elif choice == 'D':
closeProgram()
cont = False
def studyHours():
hoursFile = open('StudentsHoursGrades.txt', 'w')
student = input('Please enter your first and last name: \n')
#Validation that something was entered
while student == "":
print('Please print a valid name. \n')
student = input('Please enter your first and last name: \n')
creditHours = input('How many credit hours are you taking this semester? \n')
#Validate user entered a number
while not creditHours.isdigit() or int(creditHours)<0 or int(creditHours) >=19:
print('You entered', creditHours,'Please enter a the number of credit hours you are taking.')
creditHours = input('How many credit hours are you taking this semester? \n')
grade = input('Please enter what letter grade you want to earn: \n')
while not((grade >= 'a' and grade <='d') or (grade == 'f') or (grade >='A' and grade <= 'D') or (grade =='F')):
print('Please enter a valid letter grade of A, B, C, D, or F.')
grade = input('Please enter what letter grade you want to earn: \n')
if grade.upper() == 'A':
studyRate = 15
elif grade.upper() == 'B':
studyRate = 12
elif grade.upper() == 'C':
studyRate = 9
elif grade.upper() == 'D':
studyRate = 6
elif grade.upper() == 'F':
studyRate = 0
classHours = int(creditHours)/3
hours = int(classHours) * studyRate
hoursFile.write(student + '\n')
hoursFile.write(str(creditHours) + '\n')
hoursFile.write(str(hours) + '\n')
hoursFile.write(grade + '\n')
hoursFile.close()
print('Name: ',student)
print('Credits: ',creditHours)
print('Study Hours: ',hours)
print('Letter Grade: ',grade)
def grade():
hoursFile = open('StudentsHoursGrades.txt', 'a')
def closeProgram():
print('\nThank you for using the study time calculator.'\
'Have a great day!')
Main()

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 12 images

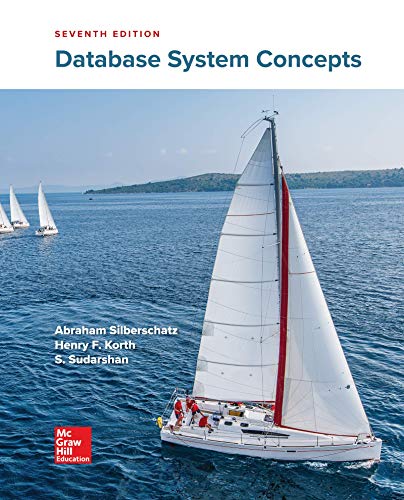
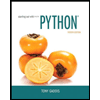
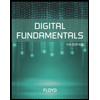
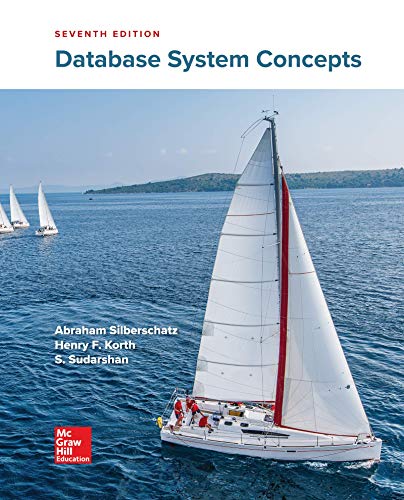
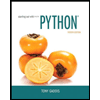
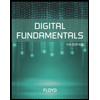
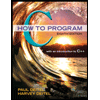
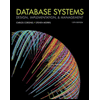
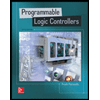