In Python: Write a for loop to print each contact in contact_emails. Sample output with inputs: 'Alf' 'alf1@hmail.com' mike.filt@bmail.com is Mike Filt s.reyn@email.com is Sue Reyn narty042@nmail.com is Nate Arty alf1@hmail.com is Alf My code so far: contact_emails = { 'Sue Reyn' : 's.reyn@email.com', 'Mike Filt': 'mike.filt@bmail.com', 'Nate Arty': 'narty042@nmail.com' } new_contact = input() new_email = input() contact_emails[new_contact] = new_email for new_contact in contact_emails: print('{} is {}'.format(new_email, contact_emails[new_contact])) However it is giving me the wrong output: alf1@hmail.com is s.reyn@email.com alf1@hmail.com is mike.filt@bmail.com alf1@hmail.com is narty042@nmail.com alf1@hmail.com is alf1@hmail.com I dont understand how to separate the contact_names from the contact_emails in the same list.
In Python:
Write a for loop to print each contact in contact_emails.
Sample output with inputs: 'Alf' 'alf1@hmail.com'
mike.filt@bmail.com is Mike Filt
s.reyn@email.com is Sue Reyn
narty042@nmail.com is Nate Arty
alf1@hmail.com is Alf
My code so far:
contact_emails = {
'Sue Reyn' : 's.reyn@email.com',
'Mike Filt': 'mike.filt@bmail.com',
'Nate Arty': 'narty042@nmail.com'
}
new_contact = input()
new_email = input()
contact_emails[new_contact] = new_email
for new_contact in contact_emails:
print('{} is {}'.format(new_email, contact_emails[new_contact]))
However it is giving me the wrong output:
alf1@hmail.com is s.reyn@email.com
alf1@hmail.com is mike.filt@bmail.com
alf1@hmail.com is narty042@nmail.com
alf1@hmail.com is alf1@hmail.com
I dont understand how to separate the contact_names from the contact_emails in the same list.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

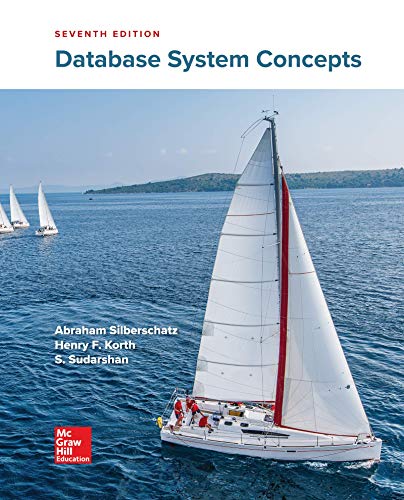
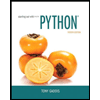
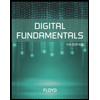
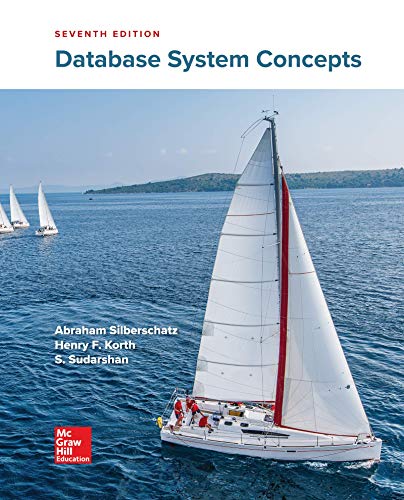
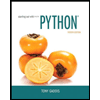
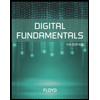
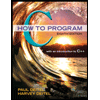
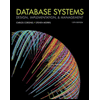
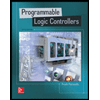