In Python. Write a program to calculate the score from a throw of five dice. Step 0. Review the provided main code. Five integer values are input and inserted into a list. The list is sorted and passed to find_high_score() to determine the highest scoring category. Make no changes to the main code. Stubs are provided for all remaining functions. Step 1. Complete the check_singles() function. Return the sum of all values that match parameter goal. Update the find_high_score() function to use a loop to call check_singles() six times with parameters being 1 - 6. Return the highest score from all function calls. Ex: If input is: 2 4 1 5 4 the output is: High score: 8 Step 2. Complete the check_three_of_kind(), check_four_of_kind(), and check_five_of_kind() functions. Hint: Since the values are in ascending order, same values are stored in consecutive index locations. Return 30 from check_three_of_kind() if the dice contain at least three of the same values. Ex: (2, 3, 3, 3, 6). Return 40 from check_four_of_kind() if the dice contain at least four of the same values. Ex: (4, 4, 4, 4, 5). Return 50 from check_five_of_kind() if the dice contain five identical values. Ex: (5, 5, 5, 5, 5). Update the find_high_score() function to call the three functions and return the highest score from all function calls. Ex: If input is: 2 4 4 5 4 the output is: High score: 30 Step 3. Complete the check_full_house() function to return 35 if the dice contain a full house (a pair and three of a kind). Ex: (1, 1, 3, 3, 3). Note: Five of a kind also satisfies the definition of a full house since (4, 4, 4, 4, 4) includes a pair of 4s and three 4s. Update the find_high_score() function to call check_full_house() and return the highest score from all function calls. Step 4. Complete the check_straight() function to return 45 if the dice contain a straight of (1, 2, 3, 4, 5) or (2, 3, 4, 5, 6). Update the find_high_score() function to call check_straight() and return the highest score from all function calls.
In Python. Write a program to calculate the score from a throw of five dice.
Step 0. Review the provided main code. Five integer values are input and inserted into a list. The list is sorted and passed to find_high_score() to determine the highest scoring category. Make no changes to the main code. Stubs are provided for all remaining functions.
Step 1. Complete the check_singles() function. Return the sum of all values that match parameter goal. Update the find_high_score() function to use a loop to call check_singles() six times with parameters being 1 - 6. Return the highest score from all function calls.
Ex: If input is:
2 4 1 5 4
the output is:
High score: 8
Step 2. Complete the check_three_of_kind(), check_four_of_kind(), and check_five_of_kind() functions. Hint: Since the values are in ascending order, same values are stored in consecutive index locations. Return 30 from check_three_of_kind() if the dice contain at least three of the same values. Ex: (2, 3, 3, 3, 6). Return 40 from check_four_of_kind() if the dice contain at least four of the same values. Ex: (4, 4, 4, 4, 5). Return 50 from check_five_of_kind() if the dice contain five identical values. Ex: (5, 5, 5, 5, 5). Update the find_high_score() function to call the three functions and return the highest score from all function calls.
Ex: If input is:
2 4 4 5 4
the output is:
High score: 30
Step 3. Complete the check_full_house() function to return 35 if the dice contain a full house (a pair and three of a kind). Ex: (1, 1, 3, 3, 3). Note: Five of a kind also satisfies the definition of a full house since (4, 4, 4, 4, 4) includes a pair of 4s and three 4s. Update the find_high_score() function to call check_full_house() and return the highest score from all function calls.
Step 4. Complete the check_straight() function to return 45 if the dice contain a straight of (1, 2, 3, 4, 5) or (2, 3, 4, 5, 6). Update the find_high_score() function to call check_straight() and return the highest score from all function calls.
![# Check for full house (score = 35)
def
check_full_house(dice):
score 0
#Type your code here.
return score
# Check for straight (score= 45)
def check_straight (dice):
score 0
#Type your code here.
return score
if ___name__ == '__main__': #Do not modify
#Fill array with five dice from input
dice [int(val) for val in input().split()]
high_score= 0
#Place dice in ascending order
dice. sort()
#Find high score and output
high score find_high_score(dice)
print(f'High score: { high_score }')](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7a4b6106-33b4-45be-b454-1e2dfd0ce02e%2F64ede9c7-aa4a-4276-a8e0-c99a039b2d16%2Fji3bhgq_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

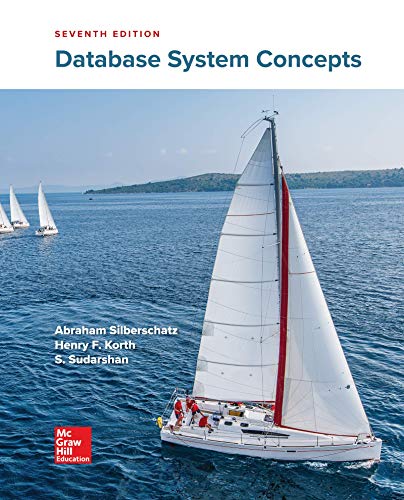
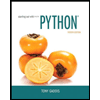
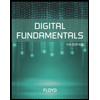
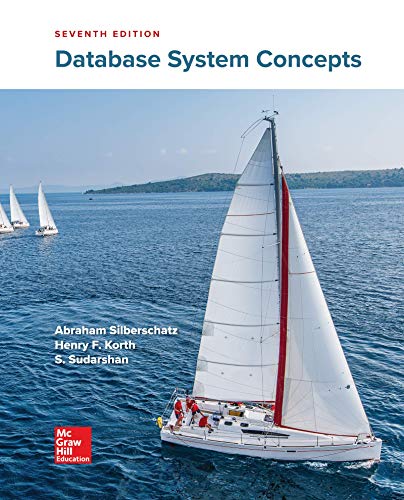
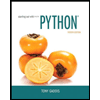
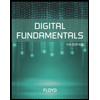
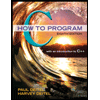
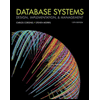
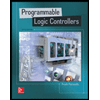