In the following questions, you will write a class called Patient that uses a class called DoctorVisit. You could imagine these classes being used in a hospital computer system. Class Descriptions The DoctorVisit class is provided as a image. A visit is described by: a reason for the visit the payment owed for the visit whether or not the visit requires the patient to return for a follow-up You will write the class called Patient. A patient is described by: an int id
In the following questions, you will write a class called Patient that uses a class called DoctorVisit. You could imagine these classes being used in a hospital computer system.
Class Descriptions
The DoctorVisit class is provided as a image. A visit is described by:
- a reason for the visit
- the payment owed for the visit
- whether or not the visit requires the patient to return for a follow-up
You will write the class called Patient. A patient is described by:
- an int id
- a name
- an ArrayList of DoctorVisit objects, representing the history of their visits
Here is the class header, instance data variables, and one constructor for the Patient class.
public class Patient {
private int id;
private String name;
private ArrayList<DoctorVisit> visitList;
public Patient(int patientID, String name) {
this.id = patientID;
this.name = name;
this.visitList = new ArrayList<DoctorVisit>();
}
1. Write getters and setters for the Patient class. If you decide not to include a setter, add a comment explaining why.
2. Write a toString method. The text representation should include:
- the patient id
- the patient name
- the number of doctor visits this patient has
3. Write a method to return the number of doctor visits the patient has.
Below is the patient tester file:
public class PatientTester {
public static void main(String[] args) {
Patient patientA = new Patient(123, "PatientA");
Patient patientB = new Patient(234, "PatientB");
DoctorVisit visit1 = new DoctorVisit("sore knee", 200, true);
Patient patientC = new Patient(345, "PatientC", visit1);
DoctorVisit visit2 = new DoctorVisit("flu", 100, false);
DoctorVisit visit3 = new DoctorVisit("earache", 100, false);
DoctorVisit visit4 = new DoctorVisit("hip pain", 500, true);
DoctorVisit visit5 = new DoctorVisit("broken ankle", 1000, true);
DoctorVisit visit6 = new DoctorVisit("store throat", 100, false);
patientA.addDoctorVisit(visit2);
patientA.addDoctorVisit(visit3);
patientC.addDoctorVisit(visit4);
patientC.addDoctorVisit(visit5);
System.out.println("PatientA toString:\n\tExpected=" + "ID: 123 Name: PatientA Visits: 2");
System.out.println("\tActual =" + patientA);
System.out.println("PatientB toString:\n\tExpected=" + "ID: 234 Name: PatientB Visits: 0");
System.out.println("\tActual =" + patientB);
System.out.println("PatientC toString:\n\tExpected=" + "ID: 345 Name: PatientC Visits: 3");
System.out.println("\tActual =" + patientC);
System.out.println("PatientA number of visits:\n\tExpected=2\n\tActual =" + patientA.getNumberOfVisits());
System.out.println("PatientB number of visits:\n\tExpected=0\n\tActual =" + patientB.getNumberOfVisits());
System.out.println("PatientC number of visits:\n\tExpected=3\n\tActual =" + patientC.getNumberOfVisits());
System.out.println("\nPatientA total payments owed: \n\tExpected=200.0\n\tActual =" + patientA.getTotalPaymentsOwed());
System.out.println("PatientB total payments owed: \n\tExpected=0.0\n\tActual =" + patientB.getTotalPaymentsOwed());
System.out.println("PatientC total payments owed: \n\tExpected=1700.0\n\tActual =" + patientC.getTotalPaymentsOwed());
System.out.println("\nPatientA has a follow up:\n\tExpected=false\n\tActual =" + patientA.hasAFollowUp());
System.out.println("PatientB has a follow up:\n\tExpected=false\n\tActual =" + patientB.hasAFollowUp());
System.out.println("PatientC has a follow up:\n\tExpected=true\n\tActual =" + patientC.hasAFollowUp());
System.out.println("\nPatientA wants to add a visit. Success?\n\tExpected=true\n\tActual =" + patientA.addDoctorVisit(visit6));
System.out.println("PatientA number of visits:\n\tExpected=3\n\tActual =" + patientA.getNumberOfVisits());
System.out.println("\nPatientC wants to add a visit. Success?\n\tExpected=false\n\tActual =" + patientC.addDoctorVisit(visit6));
System.out.println("PatientC number of visits:\n\tExpected=3\n\tActual =" + patientC.getNumberOfVisits());
System.out.println("Number of patients:\n\tExpected=3\n\tActual =???" ); // YOU CONCATENATE THE METHOD CALL HERE TO CHECK!
}
}
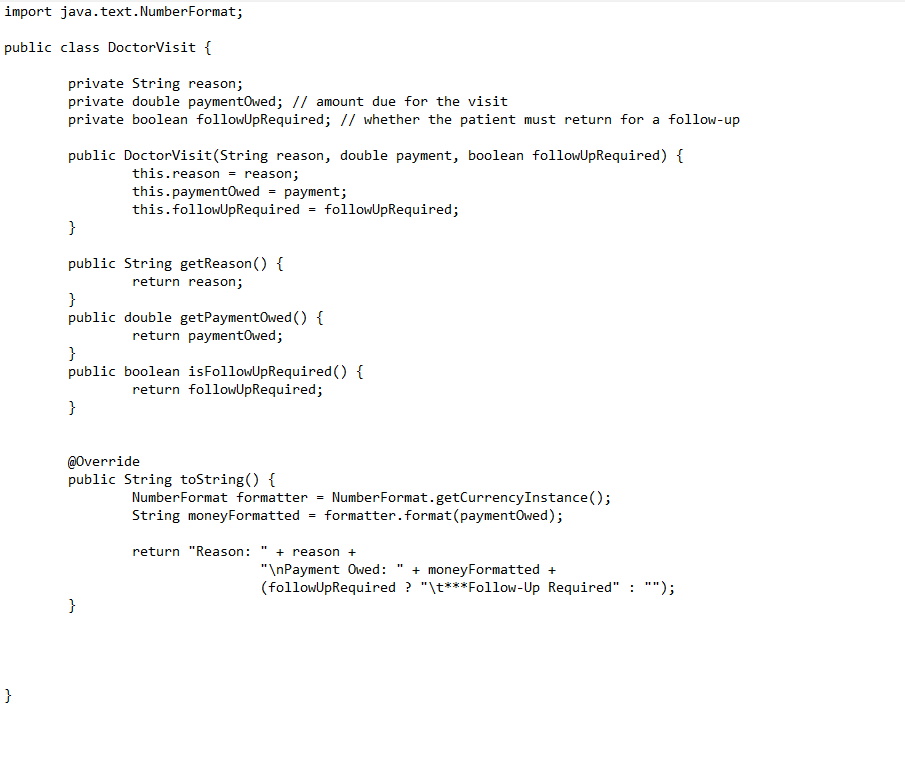

Step by step
Solved in 2 steps

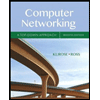
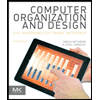
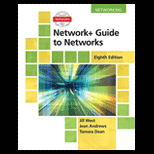
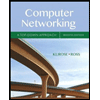
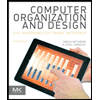
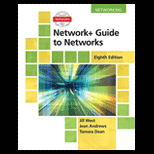
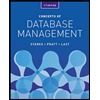
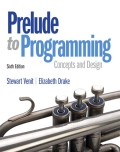
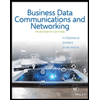