In the starting code, there is a dictionary variable dictMenu. This dictionary has keys that are strings. Each string represents a food category on the menu (e.g., "Fruits", "Drinks", ."). The keys of dictMenuhave values that are dictionaries. These associated dictionaries have key:value pairs that represent the item's name as a string and its associated price as a float. See diagram below giving a visual representation of dictMenu. dictMenu Key Value Key Value Value Key "Fruits" dictionary "Apple" 1.99 "Water" 1.00 "Drinks" dictionary "Banana" 0.59 "Juice" 3.50 "Desserts" dictionary "Grapes" 2.99 "Coffee" 1.50 "Main Items" dictionary "Pear" 1.10 "Tea" 1.24 "Soda" 1.50 Task 1 is to print out all the items in the dictMenu in the format shown below (all floats should be formatted with 2 decimal places). This task is not very complicated (only a few lines of code), but you need to think carefully about how the data is organized. Note that it is OK if your order is different than what is shown below: ------ Task 1 keys from main dictMenu. Format their output as shown. (Note - it is OK if your program prints out in a different order) ----Fruits---- $1.99 $0.59 $1.10 $2.99 $2.15 ----Drinks-- Apple Banana Kiwi Grapes Реar value:key pair of the dictionaries associated with each dictMenu's key. Format their output as shown. (Note - it is OK if your program prints out in a different order) $1.00 Water $3.50 Juice $1.50 Coffee $1.50 $1.25 ----Dessert---- $2.99 $2.50 Soda Теа к Ice Cream Pie $2.75 ----Main Dishes---- $4.25 $2.88 Cake Masala Dosa Jianbing $5.15 Falafel $8.50 Pizza
In the starting code, there is a dictionary variable dictMenu. This dictionary has keys that are strings. Each string represents a food category on the menu (e.g., "Fruits", "Drinks", ."). The keys of dictMenuhave values that are dictionaries. These associated dictionaries have key:value pairs that represent the item's name as a string and its associated price as a float. See diagram below giving a visual representation of dictMenu. dictMenu Key Value Key Value Value Key "Fruits" dictionary "Apple" 1.99 "Water" 1.00 "Drinks" dictionary "Banana" 0.59 "Juice" 3.50 "Desserts" dictionary "Grapes" 2.99 "Coffee" 1.50 "Main Items" dictionary "Pear" 1.10 "Tea" 1.24 "Soda" 1.50 Task 1 is to print out all the items in the dictMenu in the format shown below (all floats should be formatted with 2 decimal places). This task is not very complicated (only a few lines of code), but you need to think carefully about how the data is organized. Note that it is OK if your order is different than what is shown below: ------ Task 1 keys from main dictMenu. Format their output as shown. (Note - it is OK if your program prints out in a different order) ----Fruits---- $1.99 $0.59 $1.10 $2.99 $2.15 ----Drinks-- Apple Banana Kiwi Grapes Реar value:key pair of the dictionaries associated with each dictMenu's key. Format their output as shown. (Note - it is OK if your program prints out in a different order) $1.00 Water $3.50 Juice $1.50 Coffee $1.50 $1.25 ----Dessert---- $2.99 $2.50 Soda Теа к Ice Cream Pie $2.75 ----Main Dishes---- $4.25 $2.88 Cake Masala Dosa Jianbing $5.15 Falafel $8.50 Pizza
Chapter2: Using Data
Section: Chapter Questions
Problem 1CP
Related questions
Question
python code easy way please

Transcribed Image Text:dictMenu = {'Fruits': {'Apple': 1.99, 'Banana': 0.59, 'Kiwi': 1.1, 'Grapes': 2.99, 'Pear': 2.15}, 'Drinks':
{'Water': 1.e, 'Juice': 3.5, 'Coffee': 1.5, 'Soda': 1.5, 'Tea': 1.25}, 'Dessert': {'Ice Cream': 2.99, 'Pie':
2.5, 'Cake': 2.75}, 'Main Dishes': {'Masala Dosa': 4.25, 'Jianbing': 2.88, 'Falafel': 5.15, 'Pizza': 8.5}}
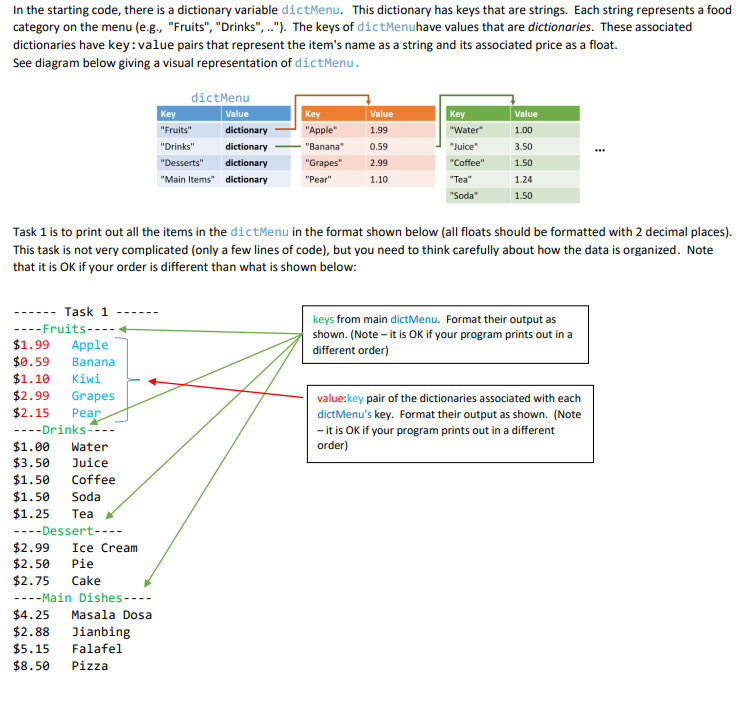
Transcribed Image Text:In the starting code, there is a dictionary variable dictMenu. This dictionary has keys that are strings. Each string represents a food
category on the menu (e.g., "Fruits", "Drinks", ."). The keys of dictMenuhave values that are dictionaries. These associated
dictionaries have key:value pairs that represent the item's name as a string and its associated price as a float.
See diagram below giving a visual representation of dictMenu.
dictMenu
Key
Key
Value
Value
Key
Value
"Fruits"
dictionary
"Apple"
1.99
"Water"
1.00
"Drinks"
dictionary
"Banana"
0.59
"Juice"
3.50
"Desserts"
dictionary
"Grapes"
2.99
"Coffee"
1.50
"Main Items" dictionary
"Pear"
1.10
"Tea"
1.24
"Soda"
1.50
Task 1 is to print out all the items in the dictMenu in the format shown below (all floats should be formatted with 2 decimal places).
This task is not very complicated (only a few lines of code), but you need to think carefully about how the data is organized. Note
that it is OK if your order is different than what is shown below:
--- Task 1
keys from main dictMenu. Format their output as
----Fruits----+
shown. (Note – it is OK if your program prints out in a
$1.99
$0.59
Apple
different order)
Banana
$1.10
Kiwi
$2.99
Grapes
value:key pair of the dictionaries associated with each
$2.15
Рear
dictMenu's key. Format their output as shown. (Note
- it is OK if your program prints out in a different
order)
----Drinks----
$1.00
$3.50
Water
Juice
$1.50
$1.50
Coffee
Soda
$1.25
Tea
----Dessert----
$2.99
$2.50
Ice Cream
Pie
$2.75
Cake
----Main Dishes----
$4.25
Masala Dosa
$2.88
Jianbing
Falafel
$5.15
$8.50
Pizza
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
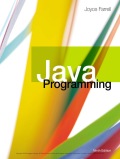
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
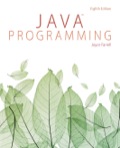
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
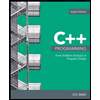
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
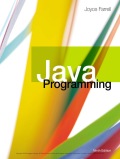
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
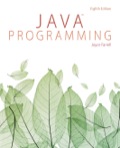
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
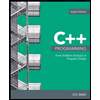
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning