In this assignment you are to use pointers to manipulate dynamically created arrays. An array's name is basically a pointer to the fisrt element of the array. Dynamic arrays are created using pointer syntax, but can subsequently use array syntax, which is the best practice approach. Instructions (main.cpp) Inside main.cpp implement the following functions: int makeArray (const int size); This function dynamically allocates an int array of the specified size. • size-the size of the dynamically allocated array. • returns - a dynamically allocated integer array of size elements. void initializeArray (int * array, const int size, const int initValue); This function initializes an array of size elements with the initValue. array-The array whose elements are to be initialized. size the size of the array. initValue the value with which to initialize each element. int duplicateArray (const int const sourceArray, const int size); This function duplicates an array of size elements. When copying dynamic instances of any kind you must be careful not to perform a shallow copy when a true duplicate is desired. A shallow copy simply assigns the contents of one pointer to another, i.e. ptr1= ptr2; A duplicate is a deep copy and requires memory allocation, i.e. ptr1 = new .... • sourceArray- The array being duplicated. Notice how neither the pointer nor the array are allowed to change (both const). • size-the size of the array being duplicated. • returns - a dynamically allocated array which is a duplicate of the source array. Note: The function mush dynamically create a new array and initialize each element with the corresponding source array's elements. string describeArray (const int const array, const int size); This function returns a formatted description of the array. • array-the constant pointer to a constant int. Neither the elements nor the pointer can be changed. size the size of the array. returns-returns a formatted description of the instance, i.e. [v1, v2...], where v1, v2... are the values of each element. This function uses the seekp() method of the ostringstream class to remove one character from the end of the stream. This removes the last, before the ] is added to complete the formatting. Example The following code: int sourceArray makeArray (5); initializeArray (sourceArray, 5, 5); int targetArray - duplicateArray (sourceArray, 5); cout << "source: " << describeArray (sourceArray, 5) << ", " << sourceArray << endl << "target: " << describeArray (targetArray, 5) << ", " << targetArray; produces the output: source: [5,5,5,5,51, 0x7f9fa4405aa0 target: [5,5,5,5,5), 0x7f9fa4405ac0 Notice how the two arrays, sourceArray and targetArray are two distinct memory locations rather than the same location. This implies a deep copy rather a shallow copy.
In this assignment you are to use pointers to manipulate dynamically created arrays. An array's name is basically a pointer to the fisrt element of the array. Dynamic arrays are created using pointer syntax, but can subsequently use array syntax, which is the best practice approach. Instructions (main.cpp) Inside main.cpp implement the following functions: int makeArray (const int size); This function dynamically allocates an int array of the specified size. • size-the size of the dynamically allocated array. • returns - a dynamically allocated integer array of size elements. void initializeArray (int * array, const int size, const int initValue); This function initializes an array of size elements with the initValue. array-The array whose elements are to be initialized. size the size of the array. initValue the value with which to initialize each element. int duplicateArray (const int const sourceArray, const int size); This function duplicates an array of size elements. When copying dynamic instances of any kind you must be careful not to perform a shallow copy when a true duplicate is desired. A shallow copy simply assigns the contents of one pointer to another, i.e. ptr1= ptr2; A duplicate is a deep copy and requires memory allocation, i.e. ptr1 = new .... • sourceArray- The array being duplicated. Notice how neither the pointer nor the array are allowed to change (both const). • size-the size of the array being duplicated. • returns - a dynamically allocated array which is a duplicate of the source array. Note: The function mush dynamically create a new array and initialize each element with the corresponding source array's elements. string describeArray (const int const array, const int size); This function returns a formatted description of the array. • array-the constant pointer to a constant int. Neither the elements nor the pointer can be changed. size the size of the array. returns-returns a formatted description of the instance, i.e. [v1, v2...], where v1, v2... are the values of each element. This function uses the seekp() method of the ostringstream class to remove one character from the end of the stream. This removes the last, before the ] is added to complete the formatting. Example The following code: int sourceArray makeArray (5); initializeArray (sourceArray, 5, 5); int targetArray - duplicateArray (sourceArray, 5); cout << "source: " << describeArray (sourceArray, 5) << ", " << sourceArray << endl << "target: " << describeArray (targetArray, 5) << ", " << targetArray; produces the output: source: [5,5,5,5,51, 0x7f9fa4405aa0 target: [5,5,5,5,5), 0x7f9fa4405ac0 Notice how the two arrays, sourceArray and targetArray are two distinct memory locations rather than the same location. This implies a deep copy rather a shallow copy.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![In this assignment you are to use pointers to manipulate dynamically created arrays.
An array's name is basically a pointer to the fisrt element of the array. Dynamic arrays are created using pointer syntax, but
can subsequently use array syntax, which is the best practice approach.
Instructions (main.cpp)
Inside main.cpp implement the following functions:
int makeArray (const int size);
This function dynamically allocates an int array of the specified size.
• size the size of the dynamically allocated array.
• returns - a dynamically allocated integer array of size elements.
void initializeArray (int * array, const int size, const int initValue);
This function initializes an array of size elements with the initValue.
array-The array whose elements are to be initialized.
size the size of the array.
initValue the value with which to initialize each element.
int
duplicateArray (const int const sourceArray, const int size);
This function duplicates an array of size elements. When copying dynamic instances of any kind you must be careful not
to perform a shallow copy when a true duplicate is desired.
A shallow copy simply assigns the contents of one pointer to another, i.e. ptr1= ptr2; A duplicate is a deep copy and
requires memory allocation, i.e. ptr1 = new ....
• sourceArray - The array being duplicated. Notice how neither the pointer nor the array are allowed to change (both
const).
• size - the size of the array being duplicated.
• returns - a dynamically allocated array which is a duplicate of the source array.
Note: The function mush dynamically create a new array and initialize each element with the corresponding source array's
elements.
string describeArray (const int const array, const int size);
This function returns a formatted description of the array.
. array- the constant pointer to a constant int. Neither the elements nor the pointer can be changed.
size - the size of the array.
returns-returns a formatted description of the instance, i.e. [v1, v2...], where v1, v2... are the values of each
element.
This function uses the seekp() method of the ostringstream class to remove one character from the end of the
stream. This removes the last, before the ] is added to complete the formatting.
Example
The following code:
int sourceArray makeArray (5);
initializeArray (sourceArray, 5, 5);
int targetArray duplicateArray (sourceArray, 5);
cout << "source: " << describeArray (sourceArray, 5) << ", " << sourceArray << endl
<< "target: " << describeArray (targetArray, 5) << ", " << targetArray;
produces the output:
source: [5,5,5,5,51, 0x7f9fa4405aa0
target: [5,5,5,5,5], 0x7f9fa4405ac0
Notice how the two arrays, sourceArray and targetArray are two distinct memory locations rather than the same
location. This implies a deep copy rather a shallow copy.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe65db211-a834-48fa-9a9d-c4296262ab17%2F3c54001d-a1f2-4113-a7c8-c1b5539b95d1%2Fvksr3gd_processed.png&w=3840&q=75)
Transcribed Image Text:In this assignment you are to use pointers to manipulate dynamically created arrays.
An array's name is basically a pointer to the fisrt element of the array. Dynamic arrays are created using pointer syntax, but
can subsequently use array syntax, which is the best practice approach.
Instructions (main.cpp)
Inside main.cpp implement the following functions:
int makeArray (const int size);
This function dynamically allocates an int array of the specified size.
• size the size of the dynamically allocated array.
• returns - a dynamically allocated integer array of size elements.
void initializeArray (int * array, const int size, const int initValue);
This function initializes an array of size elements with the initValue.
array-The array whose elements are to be initialized.
size the size of the array.
initValue the value with which to initialize each element.
int
duplicateArray (const int const sourceArray, const int size);
This function duplicates an array of size elements. When copying dynamic instances of any kind you must be careful not
to perform a shallow copy when a true duplicate is desired.
A shallow copy simply assigns the contents of one pointer to another, i.e. ptr1= ptr2; A duplicate is a deep copy and
requires memory allocation, i.e. ptr1 = new ....
• sourceArray - The array being duplicated. Notice how neither the pointer nor the array are allowed to change (both
const).
• size - the size of the array being duplicated.
• returns - a dynamically allocated array which is a duplicate of the source array.
Note: The function mush dynamically create a new array and initialize each element with the corresponding source array's
elements.
string describeArray (const int const array, const int size);
This function returns a formatted description of the array.
. array- the constant pointer to a constant int. Neither the elements nor the pointer can be changed.
size - the size of the array.
returns-returns a formatted description of the instance, i.e. [v1, v2...], where v1, v2... are the values of each
element.
This function uses the seekp() method of the ostringstream class to remove one character from the end of the
stream. This removes the last, before the ] is added to complete the formatting.
Example
The following code:
int sourceArray makeArray (5);
initializeArray (sourceArray, 5, 5);
int targetArray duplicateArray (sourceArray, 5);
cout << "source: " << describeArray (sourceArray, 5) << ", " << sourceArray << endl
<< "target: " << describeArray (targetArray, 5) << ", " << targetArray;
produces the output:
source: [5,5,5,5,51, 0x7f9fa4405aa0
target: [5,5,5,5,5], 0x7f9fa4405ac0
Notice how the two arrays, sourceArray and targetArray are two distinct memory locations rather than the same
location. This implies a deep copy rather a shallow copy.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
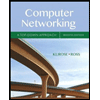
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
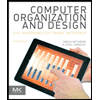
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
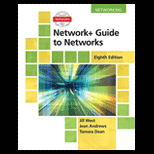
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
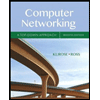
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
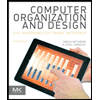
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
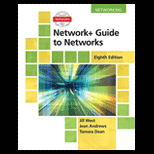
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
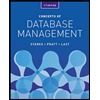
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
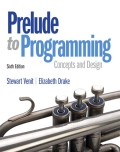
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
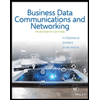
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY