In this assignment you will be responsible for writing several string validation and manipulation functions. The assumption will be that you are writing functions that will take input from a form and manipulate or validate the information entered before it is processed into a database. A database is a collection of information but the information in the database must always be entered in a specific format. Your responsibility will be to take data entered into a program and be sure it is written correctly before it is entered into the database. Each function will have to have a specific name and heading. Each function will also need to include a docstring, the correct logic, and the proper return. Each function will be given a specific set of preconditions and postconditions/returns. A precondition is something you may assume to be true when the function is executed. A post condition is something you need to be sure is completed when the function is executed. Be sure to test each one of your functions against a variety of inputs to be sure it works correctly. only work with the tools of strings and do not use any additional libraries or regular expression. the goal is to assess your abilitiy to use strings and their methods as well as selection and iterative structure. Function 1 - formatData(information: str) → str This function will format the information passed by parameter to be in all uppercase letters and remove all white space before the first character and after the last character. ● Precondition: information is a string ● Postconditon: returns a string with no leading or trailing white space and in all uppercase. Function 2 - getFirstName(fullName: str) → str This function is going to return someone's first name when past a full name by parameter ● Preconditions: fullName parameter is a sting with at least 2 names each separated by a single space. ● Postcondition: returns a string containing only the first name which is all characters from the start of full name to the first space. Function 3 - getLastName(fullName: str) → str This function will return someone’s last name when past a full name by parameter ● Precondition: fullName is a string with at least 2 names each separated by a single space. A last name is always the set of characters after the last space in fullName. ● Postcondition: returns a string containing only the last name. Function 4 - validateEmail(emailAddress: str) → boolean This function is to validate an email address based on the listed criteria. 1. It must include the @ symbol 2. it must end with .com, .org, or .net ● Precondition: the parameter emailAddress will be of proper length, include a name, domain name and top level domain. ● Postcodition: will return true if it is a valid email address and false otherwise Function 5 - validatePhoneNumber(number: str) → boolean This function is to validate a phone number. A valid phone number must meet the listed criteria 1. Have 10 digits and 12 characters total 2. Be in the format 3 digits, a “-”, 3 digit, a “-” 4 digits (ie 732-124-9834) ● Precondition: number is a string containing any alpha,numeric, and symbol characters ● Postcondition: return true if it is a valid phone number, false otherwise Function 6 - validatePassword(password: str) → boolean This function will validate a password based on the given criteria: 1. password contains at least 10 characters 2. Has at least 1 lowercase letter, 1 upper case letter, and 1 digit 3. Has at least 1 special symbol from the following: ! @ # $ % ^ & * ( ) ? ● Precondition: password is a string possibly containing characters, number, and symbols ● Postcondition: returns true if it is a valid password, false otherwise in python
In this assignment you will be responsible for writing several string validation and manipulation
functions. The assumption will be that you are writing functions that will take input from a form and
manipulate or validate the information entered before it is processed into a
collection of information but the information in the database must always be entered in a specific
format. Your responsibility will be to take data entered into a program and be sure it is written
correctly before it is entered into the database.
Each function will have to have a specific name and heading. Each function will also need to include
a docstring, the correct logic, and the proper return. Each function will be given a specific set of
preconditions and postconditions/returns. A precondition is something you may assume to be true
when the function is executed. A post condition is something you need to be sure is completed when
the function is executed. Be sure to test each one of your functions against a variety of inputs to be
sure it works correctly.
only work with the tools of strings and do not use any additional libraries or
regular expression. the goal is to assess your abilitiy to use
strings and their methods as well as selection and iterative structure.
Function 1 - formatData(information: str) → str
This function will format the information passed by parameter to be in all uppercase letters and
remove all white space before the first character and after the last character.
● Precondition: information is a string
● Postconditon: returns a string with no leading or trailing white space and in all uppercase.
Function 2 - getFirstName(fullName: str) → str
This function is going to return someone's first name when past a full name by parameter
● Preconditions: fullName parameter is a sting with at least 2 names each separated by a single
space.
● Postcondition: returns a string containing only the first name which is all characters from the
start of full name to the first space.
Function 3 - getLastName(fullName: str) → str
This function will return someone’s last name when past a full name by parameter
● Precondition: fullName is a string with at least 2 names each separated by a single space. A
last name is always the set of characters after the last space in fullName.
● Postcondition: returns a string containing only the last name.
Function 4 - validateEmail(emailAddress: str) → boolean
This function is to validate an email address based on the listed criteria.
1. It must include the @ symbol
2. it must end with .com, .org, or .net
● Precondition: the parameter emailAddress will be of proper length, include a name, domain
name and top level domain.
● Postcodition: will return true if it is a valid email address and false otherwise
Function 5 - validatePhoneNumber(number: str) → boolean
This function is to validate a phone number. A valid phone number must meet the listed
criteria
1. Have 10 digits and 12 characters total
2. Be in the format 3 digits, a “-”, 3 digit, a “-” 4 digits (ie 732-124-9834)
● Precondition: number is a string containing any alpha,numeric, and symbol characters
● Postcondition: return true if it is a valid phone number, false otherwise
Function 6 - validatePassword(password: str) → boolean
This function will validate a password based on the given criteria:
1. password contains at least 10 characters
2. Has at least 1 lowercase letter, 1 upper case letter, and 1 digit
3. Has at least 1 special symbol from the following: ! @ # $ % ^ & * ( ) ?
● Precondition: password is a string possibly containing characters, number, and symbols
● Postcondition: returns true if it is a valid password, false otherwise
in python

Step by step
Solved in 3 steps with 1 images

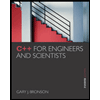
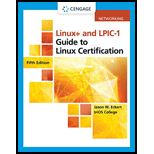
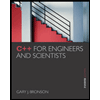
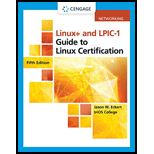