In this lab, you complete a partially written C++ program for an airline that offers a 25 percent discount to passengers who are 6 years old or younger and the same discount to passengers who are 65 years old or older. The program should request a passenger’s name and age and then print whether the passenger is eligible or not eligible for a discount. Instructions Ensure the file named Airline.cpp is open in the code editor. Variables have been declared and initialized for you, and the input statements have been written. Read them carefully before you proceed to the next step. Design the logic deciding whether to use AND or OR logic. Write the decision statement to identify when a discount should be offered and when a discount should not be offered. Be sure to include output statements telling whether or not the customer is eligible for a discount. Execute the program by clicking the Run button and enter the following as input, and verify the outputs are correct: Customer Name: Will Moriarty Customer Age : 11 Passenger is not eligible for a discount. Customer Name: James Chung Customer Age : 64 Passenger is not eligible for a discount. Customer Name: Darlene Sanchez Customer Age : 75 Passenger is eligible for a discount. Customer Name: Ray Sanchez Customer Age : 60 Passenger is not eligible for a discount. Customer Name: Tommy Sanchez Customer Age : 6 Passenger is eligible for a discount. Customer Name: Amy Patel Customer Age : 8 Passenger is not eligible for a discount. Strictly use the given code // Airline.cpp - This program determines if an airline passenger is // eligible for a 25% discount. #include #include using namespace std; int main() { string passengerFirstName = ""; // Passenger's first name string passengerLastName = ""; // Passenger's last name int passengerAge = 0; // Passenger's age // This is the work done in the housekeeping() function cout << "Enter passenger's first name: "; cin >> passengerFirstName; cout << "Enter passenger's last name: "; cin >> passengerLastName; cout << "Enter passenger's age: "; cin >> passengerAge; // This is the work done in the detailLoop() function // Test to see if this customer is eligible for a 25% discount // This is the work done in the endOfJob() function return 0; }
In this lab, you complete a partially written C++ program for an airline that offers a 25 percent discount to passengers who are 6 years old or younger and the same discount to passengers who are 65 years old or older. The program should request a passenger’s name and age and then print whether the passenger is eligible or not eligible for a discount.
Instructions
-
Ensure the file named Airline.cpp is open in the code editor.
-
Variables have been declared and initialized for you, and the input statements have been written. Read them carefully before you proceed to the next step.
-
Design the logic deciding whether to use AND or OR logic. Write the decision statement to identify when a discount should be offered and when a discount should not be offered.
-
Be sure to include output statements telling whether or not the customer is eligible for a discount.
Execute the program by clicking the Run button and enter the following as input, and verify the outputs are correct:
Customer Name: Will Moriarty Customer Age : 11 Passenger is not eligible for a discount.
Customer Name: James Chung Customer Age : 64 Passenger is not eligible for a discount.
Customer Name: Darlene Sanchez Customer Age : 75 Passenger is eligible for a discount.
Customer Name: Ray Sanchez Customer Age : 60 Passenger is not eligible for a discount.
Customer Name: Tommy Sanchez Customer Age : 6 Passenger is eligible for a discount.
Customer Name: Amy Patel Customer Age : 8 Passenger is not eligible for a discount.
Strictly use the given code

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

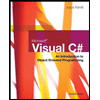
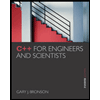
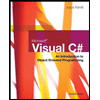
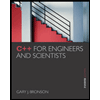