In this lab, you complete a prewritten C++ program that calculates an employee’s productivity bonus and prints the employee’s name and bonus. Bonuses are calculated based on an employee’s productivity score as shown below. A productivity score is calculated by first dividing an employee’s transactions dollar value by the number of transactions and then dividing the result by the number of shifts worked. Productivity Score Bonus <=30 $50 31–69 $75 70–199 $100 >= 200 $200
In this lab, you complete a prewritten C++ program that calculates an employee’s productivity bonus and prints the employee’s name and bonus. Bonuses are calculated based on an employee’s productivity score as shown below. A productivity score is calculated by first dividing an employee’s transactions dollar value by the number of transactions and then dividing the result by the number of shifts worked.
Productivity Score | Bonus |
---|---|
<=30 | $50 |
31–69 | $75 |
70–199 | $100 |
>= 200 | $200 |
Instructions
-
Ensure the file named EmployeeBonus.cpp is open in the code editor.
-
Variables have been declared for you, and the input statements and output statements have been written. Read them over carefully before you proceed to the next step.
-
Design the logic, and write the rest of the program using a nested if statement.
-
Execute the program by clicking the Run button and enter the following as input:
Employee’s first name: Kim Employee's last name: Smith Number of shifts: 25 Number of transactions: 75 Transaction dollar value: 40000.00 - Your output should be:Employee Name: Kim Smith Employee Bonus: $50.0
-
// EmployeeBonus.cpp - This program calculates an employee's yearly bonus.
#include <iostream>#include <string>using namespace std;int main(){// Declare and initialize variables herestring employeeFirstName;string employeeLastName;double numTransactions;double numShifts;double dollarValue;double score;double bonus;const double BONUS_1 = 50.00;const double BONUS_2 = 75.00;const double BONUS_3 = 100.00;const double BONUS_4 = 200.00;// This is the work done in the housekeeping() functioncout << "Enter employee's first name: ";cin >> employeeFirstName;cout << "Enter employee's last name: ";cin >> employeeLastName;cout << "Enter number of shifts: ";cin >> numShifts;cout << "Enter number of transactions: ";cin >> numTransactions;cout << "Enter dollar value of transactions: ";cin >> dollarValue;
// This is the work done in the detailLoop()function// Write your code here// This is the work done in the endOfJob() function// Output.cout << "Employee Name: " << employeeFirstName << " " << employeeLastName << endl;cout << "Employee Bonus: $" << bonus << endl;return 0;}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

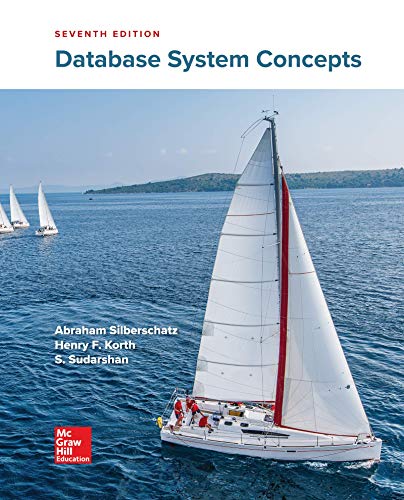
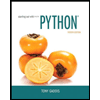
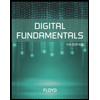
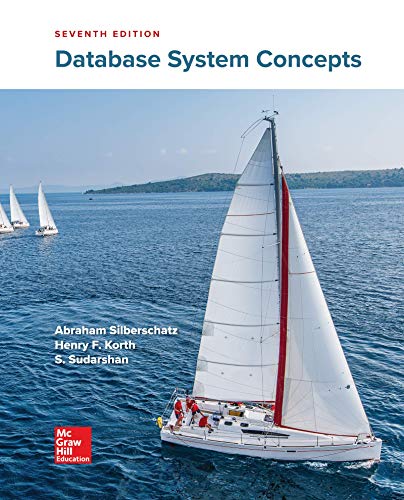
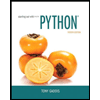
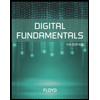
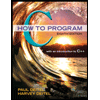
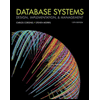
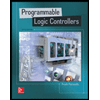