In this lab you will practice using Python. Most of the elements within this lab is the examples that are used within the book. Do not be afraid of Python! It is a great programming language and once mastered you will see some of the similarities between this scripting language and Object Oriented languages. Part 1 Log into your virtual machine. Make sure you are in your Home directory. Note that when you log into your virtual machine, you will be defaulted to your Home directory. Invoke the Python interactive session by typing in python at the shell prompt and press enter(screenshot 1). Type in the following at the screen prompt: >>> 5+2 and press enter. >>> 'Here is a string.' and press enter >>> range(1,11) and press enter. (screenshot 2 for all examples above) Exit out of interactive mode by pressing d Part 2 Create a file called gm.py. To do this, using vi, type in vi gm.py and press enter. Remember, the extension is not necessary in Linux filesystem, but it does help in determining which language you are using. Type in the following code in the file: #!/usr/bin/python print 'Good morning!' Save and exit your program. Make sure you make your file executable by typing in chmod u+x gm.py and press enter. Execute the program by typing in ./gm.py and press enter(screenshot 3). Type in python gm.py and press enter(screenshot 4) Part 3 Create a python program called mypy.py using vi. Once you open the file, type in the following program: untitled #!/usr/bin/python inp = raw_input('Enter the name of a month: ') print 'You entered ' + inp Save and exit your program. Make the file executable by typing in chmod u+x mypy.py and press enter(screenshot 5). Run the program by typing in python mypy.py and press enter. Enter June for the month and press enter (screenshot 6) Part 4 Let's go back to the interactive shell by typing in python and press enter. Type in the following commands at the python prompt: untitled >>> mon = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun'] >>> print mon (screenshot 7) Let's now create a for loop to iterate though our elements. Type in the following code in the python prompt: >>> for nam in mon: ... print nam + '.' (screenshot 8) Now you will sort the names of the month in alphabetical order. to do this, type in the following command at the interactive shell prompt: >>> mon.sort() >>>mon (screenshot 9) Part 5 Now we will Instantiate a dictionary in which the keys are the months in the third quarter of the year and the values are the number of days in the corresponding month. Type in the following code: untitled >>> days = {'July': 31, 'August': 31, 'September': 30} and press enter >>> days (screenshot 10) Type in the following >>> days.keys() (screenshot 11) Type in the following: >>> days.values() (screenshot 12) Exit out of interactive mode by pressing d Part 6 Next thing we will do is create a script to guess numbers. Create a script called number_guess.py in vi and press enter. Type in the script below: #!/usr/bin/python def guessANumber(val): guess = int(input('Enter your guess: ')) if guess > val: print 'Too high.' return 1 elif guess < val: print 'Too low.' return -1 else: print 'Got it!' return 0 while (guessANumber(6) != 0): print 'Guess again.', Save and exit your script (screenshot 13). Make the script executable by typing in chmod u+x number_guess.py and press enter. Run the script by typing in ./number_guess.py and press enter. Give me 3 outputs to show that the script ran correctly.(screenshot 14) Part 7 Create a Perl script below. Using vi, type in the following script. #!/usr/bin/perl -w print "Enter a number: "; $num1 = <>; print "Enter another, different number: "; $num2 = <>; if ($num1 == $num2) { die ("Please enter two different numbers.\n"); } if ($num1 > $num2) { print "The first number is greater than the second number.\n"; } else { print "The first number is less than the second number.\n"; } Save your file and call the file ifelse.pl Make the file executable and run the script. Type in values that are the same (screenshot 15) Type in the first value larger than the second value (screenshot 16) Type in the second value larger than the first value(screenshot 17) Part 8 Create a Perl script below, name the file arrayvar2.pl. Using vi, type in the following script. #!/usr/bin/perl -w @arrayvar2 = ("apple", "bird", 44, "Tike", "metal", "pike"); $num = @arrayvar2; # number of elements in array print "Elements: ", $num, "\n"; # two equivalent print statements print "Elements: $num\n"; print "Last: $#arrayvar2\n"; # index of last element in array Save your file and call the file arrayvar2.pl Make the file executable and run the script. Screenshot your output (screenshot 18)
In this lab you will practice using Python. Most of the elements within this lab is the examples that are used within the book. Do not be afraid of Python! It is a great programming language and once mastered you will see some of the similarities between this scripting language and Object Oriented languages. Part 1 Log into your virtual machine. Make sure you are in your Home directory. Note that when you log into your virtual machine, you will be defaulted to your Home directory. Invoke the Python interactive session by typing in python at the shell prompt and press enter(screenshot 1). Type in the following at the screen prompt: >>> 5+2 and press enter. >>> 'Here is a string.' and press enter >>> range(1,11) and press enter. (screenshot 2 for all examples above) Exit out of interactive mode by pressing d Part 2 Create a file called gm.py. To do this, using vi, type in vi gm.py and press enter. Remember, the extension is not necessary in Linux filesystem, but it does help in determining which language you are using. Type in the following code in the file: #!/usr/bin/python print 'Good morning!' Save and exit your program. Make sure you make your file executable by typing in chmod u+x gm.py and press enter. Execute the program by typing in ./gm.py and press enter(screenshot 3). Type in python gm.py and press enter(screenshot 4) Part 3 Create a python program called mypy.py using vi. Once you open the file, type in the following program: untitled #!/usr/bin/python inp = raw_input('Enter the name of a month: ') print 'You entered ' + inp Save and exit your program. Make the file executable by typing in chmod u+x mypy.py and press enter(screenshot 5). Run the program by typing in python mypy.py and press enter. Enter June for the month and press enter (screenshot 6) Part 4 Let's go back to the interactive shell by typing in python and press enter. Type in the following commands at the python prompt: untitled >>> mon = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun'] >>> print mon (screenshot 7) Let's now create a for loop to iterate though our elements. Type in the following code in the python prompt: >>> for nam in mon: ... print nam + '.' (screenshot 8) Now you will sort the names of the month in alphabetical order. to do this, type in the following command at the interactive shell prompt: >>> mon.sort() >>>mon (screenshot 9) Part 5 Now we will Instantiate a dictionary in which the keys are the months in the third quarter of the year and the values are the number of days in the corresponding month. Type in the following code: untitled >>> days = {'July': 31, 'August': 31, 'September': 30} and press enter >>> days (screenshot 10) Type in the following >>> days.keys() (screenshot 11) Type in the following: >>> days.values() (screenshot 12) Exit out of interactive mode by pressing d Part 6 Next thing we will do is create a script to guess numbers. Create a script called number_guess.py in vi and press enter. Type in the script below: #!/usr/bin/python def guessANumber(val): guess = int(input('Enter your guess: ')) if guess > val: print 'Too high.' return 1 elif guess < val: print 'Too low.' return -1 else: print 'Got it!' return 0 while (guessANumber(6) != 0): print 'Guess again.', Save and exit your script (screenshot 13). Make the script executable by typing in chmod u+x number_guess.py and press enter. Run the script by typing in ./number_guess.py and press enter. Give me 3 outputs to show that the script ran correctly.(screenshot 14) Part 7 Create a Perl script below. Using vi, type in the following script. #!/usr/bin/perl -w print "Enter a number: "; $num1 = <>; print "Enter another, different number: "; $num2 = <>; if ($num1 == $num2) { die ("Please enter two different numbers.\n"); } if ($num1 > $num2) { print "The first number is greater than the second number.\n"; } else { print "The first number is less than the second number.\n"; } Save your file and call the file ifelse.pl Make the file executable and run the script. Type in values that are the same (screenshot 15) Type in the first value larger than the second value (screenshot 16) Type in the second value larger than the first value(screenshot 17) Part 8 Create a Perl script below, name the file arrayvar2.pl. Using vi, type in the following script. #!/usr/bin/perl -w @arrayvar2 = ("apple", "bird", 44, "Tike", "metal", "pike"); $num = @arrayvar2; # number of elements in array print "Elements: ", $num, "\n"; # two equivalent print statements print "Elements: $num\n"; print "Last: $#arrayvar2\n"; # index of last element in array Save your file and call the file arrayvar2.pl Make the file executable and run the script. Screenshot your output (screenshot 18)
A+ Guide To It Technical Support
10th Edition
ISBN:9780357108291
Author:ANDREWS, Jean.
Publisher:ANDREWS, Jean.
Chapter14: Troubleshooting Windows After Startup
Section: Chapter Questions
Problem 7TC
Related questions
Question
In this lab you will practice using Python. Most of the elements within this lab is the examples that are used within the book. Do not be afraid of Python! It is a great programming language and once mastered you will see some of the similarities between this scripting language and Object Oriented languages.
Part 1
Log into your virtual machine. Make sure you are in your Home directory. Note that when you log into your virtual machine, you will be defaulted to your Home directory. Invoke the Python interactive session by typing in python at the shell prompt and press enter(screenshot 1).
Type in the following at the screen prompt:
>>> 5+2 and press enter.
>>> 'Here is a string.' and press enter
>>> range(1,11) and press enter. (screenshot 2 for all examples above)
Exit out of interactive mode by pressing d
Part 2
Create a file called gm.py. To do this, using vi, type in vi gm.py and press enter. Remember, the extension is not necessary in Linux filesystem, but it does help in determining which language you are using. Type in the following code in the file:
#!/usr/bin/python
print 'Good morning!'
Save and exit your program. Make sure you make your file executable by typing in chmod u+x gm.py and press enter. Execute the program by typing in ./gm.py and press enter(screenshot 3).
Type in python gm.py and press enter(screenshot 4)
Part 3
Create a python program called mypy.py using vi. Once you open the file, type in the following program:
untitled
#!/usr/bin/python
inp = raw_input('Enter the name of a month: ')
print 'You entered ' + inp
Save and exit your program. Make the file executable by typing in chmod u+x mypy.py and press enter(screenshot 5).
Run the program by typing in python mypy.py and press enter. Enter June for the month and press enter (screenshot 6)
Part 4
Let's go back to the interactive shell by typing in python and press enter. Type in the following commands at the python prompt:
untitled
>>> mon = ['Jan', 'Feb', 'Mar', 'Apr', 'May', 'Jun']
>>> print mon
(screenshot 7)
Let's now create a for loop to iterate though our elements. Type in the following code in the python prompt:
>>> for nam in mon:
... print nam + '.'
(screenshot 8)
Now you will sort the names of the month in alphabetical order. to do this, type in the following command at the interactive shell prompt:
>>> mon.sort()
>>>mon
(screenshot 9)
Part 5
Now we will Instantiate a dictionary in which the keys are the months in the third quarter of the year and the values are the number of days in the corresponding month. Type in the following code:
untitled
>>> days = {'July': 31, 'August': 31, 'September': 30} and press enter
>>> days (screenshot 10)
Type in the following
>>> days.keys()
(screenshot 11)
Type in the following:
>>> days.values()
(screenshot 12)
Exit out of interactive mode by pressing d
Part 6
Next thing we will do is create a script to guess numbers. Create a script called number_guess.py in vi and press enter. Type in the script below:
#!/usr/bin/python
def guessANumber(val):
guess = int(input('Enter your guess: '))
if guess > val:
print 'Too high.'
return 1
elif guess < val:
print 'Too low.'
return -1
else:
print 'Got it!'
return 0
while (guessANumber(6) != 0):
print 'Guess again.',
Save and exit your script (screenshot 13).
Make the script executable by typing in chmod u+x number_guess.py and press enter.
Run the script by typing in ./number_guess.py and press enter. Give me 3 outputs to show that the script ran correctly.(screenshot 14)
Part 7
Create a Perl script below. Using vi, type in the following script.
#!/usr/bin/perl -w
print "Enter a number: ";
$num1 = <>;
print "Enter another, different number: ";
$num2 = <>;
if ($num1 == $num2) {
die ("Please enter two different numbers.\n");
}
if ($num1 > $num2) {
print "The first number is greater than the second number.\n";
}
else {
print "The first number is less than the second number.\n";
}
Save your file and call the file ifelse.pl
Make the file executable and run the script. Type in values that are the same (screenshot 15)
Type in the first value larger than the second value (screenshot 16)
Type in the second value larger than the first value(screenshot 17)
Part 8
Create a Perl script below, name the file arrayvar2.pl. Using vi, type in the following script.
#!/usr/bin/perl -w
@arrayvar2 = ("apple", "bird", 44, "Tike", "metal", "pike");
$num = @arrayvar2; # number of elements in array
print "Elements: ", $num, "\n"; # two equivalent print statements
print "Elements: $num\n";
print "Last: $#arrayvar2\n"; # index of last element in array
Save your file and call the file arrayvar2.pl
Make the file executable and run the script.
Screenshot your output (screenshot 18)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 11 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
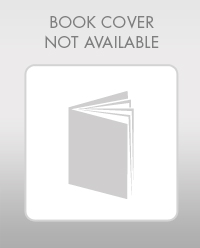
A+ Guide To It Technical Support
Computer Science
ISBN:
9780357108291
Author:
ANDREWS, Jean.
Publisher:
Cengage,
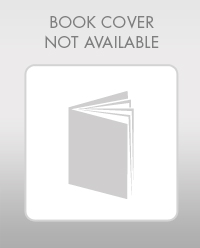
A+ Guide To It Technical Support
Computer Science
ISBN:
9780357108291
Author:
ANDREWS, Jean.
Publisher:
Cengage,