In this project, we convert a positive decimal number to its positive hexadecimal value equivalent and vice versa. The code should prompt the user for a choice of 1 or 2: • If the user enters 1 it means the user wants to convert a decimal number to its hexadecimal value equivalent. If the user enters 2 it means the user wants to convert a hexadecimal number to its decimal value equivalent Since the hexadecimal numbers include characters between A-F we have to keep a hexadecimal number as an array of characters. To read a hexadecimal number, we need to read a string. To keep this code simple, just enter and display capital letters for hexadecimal numbers. Your code needs to include at least two functions. • One function to convert a decimal number to its hexadecimal value equivalent and prints both values. • The other function to convert a hexadecimal number to its decimal value equivalent and prints both values. Input and output samples: Input values are bolded. Sample1: Enter 1 for Decimal to Hexadecimal conversion. Enter 2 for Hexadecimal to Decimal conversion. 1 Enter a positive decimal number. 255 The hexadecimal representation of 0 is Sample 2: Enter 1 for Decimal to Hexadecimal conversion. 2 Enter 2 for Hexadecimal to Decimal conversion. FF Enter a positive hexadecimal number. The hexadecimal representation of FF is 255. FF.
In this project, we convert a positive decimal number to its positive hexadecimal value equivalent and vice versa. The code should prompt the user for a choice of 1 or 2: • If the user enters 1 it means the user wants to convert a decimal number to its hexadecimal value equivalent. If the user enters 2 it means the user wants to convert a hexadecimal number to its decimal value equivalent Since the hexadecimal numbers include characters between A-F we have to keep a hexadecimal number as an array of characters. To read a hexadecimal number, we need to read a string. To keep this code simple, just enter and display capital letters for hexadecimal numbers. Your code needs to include at least two functions. • One function to convert a decimal number to its hexadecimal value equivalent and prints both values. • The other function to convert a hexadecimal number to its decimal value equivalent and prints both values. Input and output samples: Input values are bolded. Sample1: Enter 1 for Decimal to Hexadecimal conversion. Enter 2 for Hexadecimal to Decimal conversion. 1 Enter a positive decimal number. 255 The hexadecimal representation of 0 is Sample 2: Enter 1 for Decimal to Hexadecimal conversion. 2 Enter 2 for Hexadecimal to Decimal conversion. FF Enter a positive hexadecimal number. The hexadecimal representation of FF is 255. FF.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter10: Pointers
Section10.3: Pointer Arithmetic
Problem 4E
Related questions
Question
100%
Hello. Please answer the attached C
*If correctly fulfill and do all of the steps correctly, I will give you a thumbs up. Thanks.
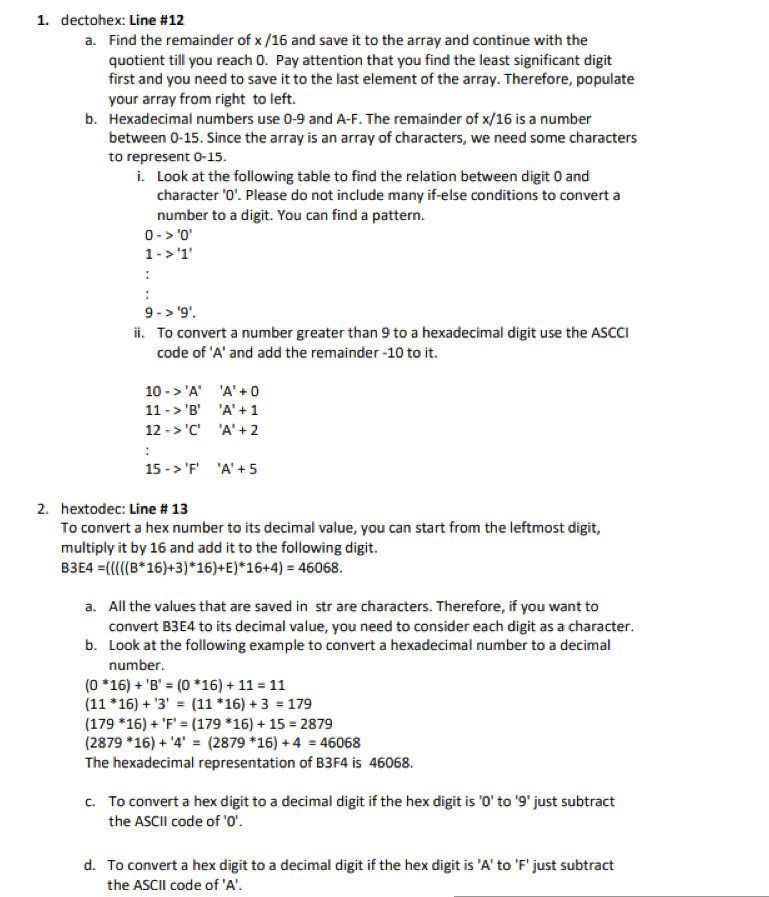
Transcribed Image Text:1. dectohex: Line #12
a. Find the remainder of x /16 and save it to the array and continue with the
quotient till you reach O. Pay attention that you find the least significant digit
first and you need to save it to the last element of the array. Therefore, populate
your array from right to left.
b. Hexadecimal numbers use 0-9 and A-F. The remainder of x/16 is a number
between 0-15. Since the array is an array of characters, we need some characters
to represent 0-15.
i. Look at the following table to find the relation between digit 0 and
character '0'. Please do not include many if-else conditions to convert a
number to a digit. You can find a pattern.
0-> '0'
1-> '1'
:
:
9-> '9'.
ii. To convert a number greater than 9 to a hexadecimal digit use the ASCCI
code of 'A' and add the remainder -10 to it.
10->'A'
11-> 'B'
12-> 'C'
:
'A' +0
'A' +1
'A' +2
15-> 'F' 'A' +5
2. hextodec: Line # 13
To convert a hex number to its decimal value, you can start from the leftmost digit,
multiply it by 16 and add it to the following digit.
B3E4 =(((((B*16)+3)*16)+E)*16+4)=46068.
a. All the values that are saved in str are characters. Therefore, if you want to
convert B3E4 to its decimal value, you need to consider each digit as a character.
b. Look at the following example to convert a hexadecimal number to a decimal
number.
(0 *16) + 'B' = (0*16) + 11 = 11
(11 *16) + '3' = (11*16) + 3 = 179
(179 *16) + 'F' = (179 *16) + 15 = 2879
(2879 *16) + '4' = (2879 *16) +4 = 46068
The hexadecimal representation of B3F4 is 46068.
c. To convert a hex digit to a decimal digit if the hex digit is '0' to '9' just subtract
the ASCII code of '0'.
d. To convert a hex digit to a decimal digit if the hex digit is 'A' to 'F' just subtract
the ASCII code of 'A'.
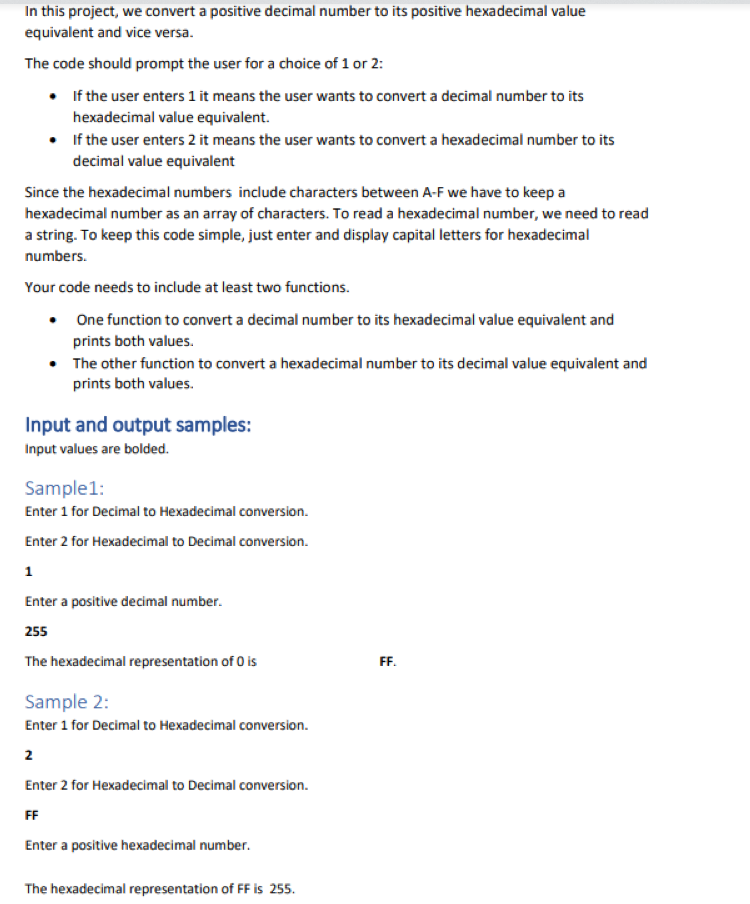
Transcribed Image Text:In this project, we convert a positive decimal number to its positive hexadecimal value
equivalent and vice versa.
The code should prompt the user for a choice of 1 or 2:
• If the user enters 1 it means the user wants to convert a decimal number to its
hexadecimal value equivalent.
• If the user enters 2 it means the user wants to convert a hexadecimal number to its
decimal value equivalent
Since the hexadecimal numbers include characters between A-F we have to keep a
hexadecimal number as an array of characters. To read a hexadecimal number, we need to read
a string. To keep this code simple, just enter and display capital letters for hexadecimal
numbers.
Your code needs to include at least two functions.
One function to convert a decimal number to its hexadecimal value equivalent and
prints both values.
• The other function to convert a hexadecimal number to its decimal value equivalent and
prints both values.
Input and output samples:
Input values are bolded.
Sample1:
Enter 1 for Decimal to Hexadecimal conversion.
Enter 2 for Hexadecimal to Decimal conversion.
1
Enter a positive decimal number.
255
The hexadecimal representation of 0 is
Sample 2:
Enter 1 for Decimal to Hexadecimal conversion.
2
Enter 2 for Hexadecimal to Decimal conversion.
FF
Enter a positive hexadecimal number.
The hexadecimal representation of FF is 255.
FF.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 5 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
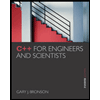
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
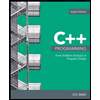
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
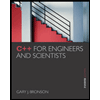
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
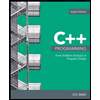
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
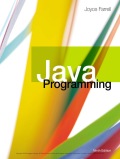
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT