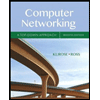
#include <stdio.h>
#include <stdlib.h>
#define SEQ_SIZE 50
#define CACHE_SIZE 3
int page_reference_sequence[SEQ_SIZE];
int read_file(char *filename) // read in the page reference sequence from the file
{
FILE *input;
int i, n;
if ((input = fopen(filename, "r")) == NULL) {
printf("The input file does not exist.\n");
exit(1);
}
for (i = 0; i < SEQ_SIZE; i++) {
if (fscanf(input, "%d", &page_reference_sequence[i]) == EOF) { // end of file
n = i; // total number of page reference
i = SEQ_SIZE; // stop reading from file
}
}
fclose(input);
printf("Input page reference sequence: ");
for (i =0; i < n; i++) {
printf("%d ", page_reference_sequence[i]);
}
printf("(total %d references)\n", n);
return n; // return the total number of page reference
}
void FIFO(int n)
{
// write your own code for the FIFO policy
// based on the input file content, print out a table similar to the Figure 22.2
int i;
printf("FIFO page reference sequence: ");
for (i = 0; i < n; i++) {
printf("%d ", page_reference_sequence[i]);
}
printf("(total %d references)\n", n);
}
void LRU(int n)
{
// write your own code for the LRU policy
// based on the input file content, print out a table similar to the Figure 22.5
int i;
printf("LRU page reference sequence: ");
for (i = 0; i < n; i++) {
printf("%d ", page_reference_sequence[i]);
}
printf("(total %d references)\n", n);
}
int main(int argc, char *argv[])
{
int i, n;
if (argc != 2) {
printf("Usage: %s input_filename\n", argv[0]);
exit(1);
}
n = read_file(argv[1]);
FIFO(n);
LRU(n);
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 3 images

- Pythonarrow_forwardUSE PYTHON MULTI THREADING TO COMPLETE DO NOT USE PROCESSES ONLY COMPLETE PART 2 using MULTI THREADING 1) Basic version with two levels of threads (master and slaves) One master thread aggregates and sums the result of n slave-threads where each slavethread sums a different range of values in an array of 1000 random integers (please program to generate 1000 random integers to populate the array). ************ONLY COMPLETE THIS PART BELOW*************** 2) Advanced version with more than two levels of threadsThe master thread creates two slave-threads where each slave-thread is responsible to sum half segment of the array. Each slave thread will fork/spawn two new slave-threads where each new slave-threadsums half of the array segment received by its parent. Each slave thread will return the subtotal to its parent thread and the parent thread aggregates and returns the total to its parent thread. Start with 7 nodes thread tree, when you are comfortable, you can extend it to a full…arrow_forwardPut the following code in a main.py file and a module.py file. Define the names sort, list_max and sum_of_list after putting the code into a main file and a module file #predefined modules import random import math #function to sort the list in ascending order def sort(x): #predefined function sort() x.sort() #print the sorted list print("\nSorted list is: ",str(x)) #function to find the sum of list elements def sum_of_list(x): #predefined function sum() Sum=sum(x) #return the sum of list elements return Sum #function to list the maximum from the list def list_max(x): #predefined function max() maximum=max(x) #return maximum return maximum #function to test the above three function def main(): #set a flag variable flag=True #create a list list1=list() #initialize the list element by using randrange() predefined function of random module list1=[random.randrange(1, 50, 1) for i in range(0,7)] #print the…arrow_forward
- PROGRAMMING EXERCISES: 4. How many double entries can aValues[MAX_A] store? 5. What is the value of aValues[2]? [Hint: the value is an address] 6. How many bytes are allocated to sentence?arrow_forwardUsing C Language In this function, b has the address of a memory buffer that is num_bytes long. The function should repeatedly copy the 16 byte pattern that pattern16 points at into the memory buffer until num_bytes have been written. If num_bytes is not a multple of 16, the final write of the 16 byte pattern should be truncated to finish filling the buffer. void memset16(void *b, int num_bytes, void *pattern16) For example if the 16 bytes that pattern16 points at is 00 11 22 33 44 55 66 77 88 99 aa bb cc dd ee ff, then memset(b, 20, pattern16) should write to the buffer pointed at by p the 20 bytes 00 11 22 33 44 55 66 77 88 99 aa bb cc dd ee ff 00 11 22 33. Use SSE instructions to improve efficiency. Here's pseudocode. x = SSE unaligned load from pattern16while (num_bytes >= 16) SSE unaligned store x to p advance p by 16 bytes decrement num_bytes by 16while (num_bytes > 0) store 1 byte from pattern16 to p advance p by 1 byte advance pattern16 by 1 byte…arrow_forwardcache.xls (or it can be a google sheet that you share with me in a cache.txt with the url in it )= a spreadsheet showing the cycle counts of a cached and non-cached machine. Also, you need to show the cache as the memory accesses progress in your program marking the hits and misses (misses in RED). You should have cells to show how many hits and how many misses your program has, and details on what is the cache replacement policy you implemented. Here is code: void c_cache_for_badge() { int na = 8, nb = -3, nc = 10; // pointers int *npa; npa = (int*)malloc(sizeof(int)*84); // init integer pointers for(int i = 0; i < 84; i++) { npa[i] = i; } // integer pointers for(int i = 9; i < 70; i= i + 3) { nb += npa[i % 5] + npa[i % 3] + npa[i % 6]; } } void main() { c_cache_for_badge(); }arrow_forward
- Computer Science #include<cmath>#include<stdio.h>__global__voidprocess_kernel1(float *input1,float *input2,float *output,int datasize){int idx = threadIdx.x + blockIdx.x * blockDim.x;int idy = threadIdx.y + blockIdx.y * blockDim.y;int idz = threadIdx.z + blockIdx.z * blockDim.z;int index = idz * (gridDim.x * blockDim.x) * (gridDim.y*blockDim.y) + idy * (gridDim.x * blockDim.x) +idx;if(index<datasize)output[index] = sinf(input1[index]) + cosf(input2[index]);}__global__voidprocess_kernel2(float *input,float *output,int datasize){int idx = threadIdx.x + blockIdx.x * blockDim.x;int idy = threadIdx.y + blockIdx.y * blockDim.y;int idz = threadIdx.z + blockIdx.z * blockDim.z;int index = idz * (gridDim.x * blockDim.x) * (gridDim.y*blockDim.y) + idy * (gridDim.x * blockDim.x) +idx;if(index<datasize)output[index] = logf(input[index]);}_global__voidprocess_kernel3(float *input,float *output,int datasize){int idx = threadIdx.x + blockIdx.x *…arrow_forwardtry to ans in 30 minarrow_forward3 4 5 5 7 3 9 3 1 2 B 4 5 7 9 0 2 3 4 5 8 0 1 def calculate_trip_time( iata_src: str, iata_dst: str, flight_walk: List[str], flights: FlightDir ) -> float: Return a float corresponding to the amount of time required to travel from the source airport to the destination airport to the destination airport, as outlined by the flight_walk. PERBE The start time of the trip should be considered zero. In other words, assuming we start the trip at 12:00am, this function should return the time it takes for the trip to finish, including all the waiting times before, and between the flights. If there is no path available, return -1.0 >>> calculate_trip_time("AA1", 2.0 >>> calculate_trip_time("AA1", >>> calculate_trip_time("AA1", >>> calculate_trip_time("AA1", 0.0 >>> calculate_trip_time("AA4", 14.0 >>> calculate_trip_time("AA1", 7.5 >>> calculate_trip_time("AA1", 2.0 *** "AA2", ["AA1", "AA2"], TEST_FLIGHTS_DIR_FOUR_CITIES) "AA7", ["AA7"] "AA1"], TEST_FLIGHTS_DIR_FOUR_CITIES) "AA7", ["AA1",…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
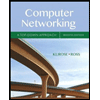
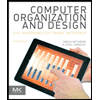
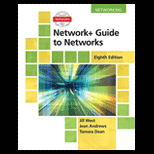
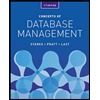
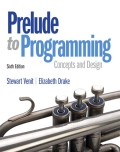
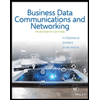