Instructions C++ a. Add the following operation to the class stackType. void reverseStack(stackType &otherStack); This operation copies the elements of a stack in reverse order onto another stack. Consider the following statements: stackType stack1; stackType stack2; The statement stack1.reverseStack(stack2); copies the elements of stack1 onto stack2 in reverse order. That is, the top element of stack1 is the bottom element of stack2, and so on. The old contents of stack2 are destroyed, and stack1 is unchanged. b. Write the definition of the function template to implement the operation reverseStack. Write a program to test the class stackType. myStack.h //Header file: myStack.h #ifndef H_StackType #define H_StackType #include #include #include "stackADT.h" using namespace std; template class stackType: public stackADT { public: const stackType& operator=(const stackType&); void initializeStack(); bool isEmptyStack() const; bool isFullStack() const; void push(const Type& newItem); Type top() const; void pop(); stackType(int stackSize = 100); stackType(const stackType& otherStack); ~stackType(); void reverseStack(stackType &otherStack); private: int maxStackSize; int stackTop; Type *list; void copyStack(const stackType& otherStack); }; template void stackType::initializeStack() { stackTop = 0; }//end initializeStack template bool stackType::isEmptyStack() const { return(stackTop == 0); }//end isEmptyStack template bool stackType::isFullStack() const { return(stackTop == maxStackSize); } //end isFullStack template void stackType::push(const Type& newItem) { if (!isFullStack()) { list[stackTop] = newItem; //add newItem to the //top of the stack stackTop++; //increment stackTop } else cout << "Cannot add to a full stack." << endl; }//end push template Type stackType::top() const { assert(stackTop != 0); //if stack is empty, //terminate the program return list[stackTop - 1]; //return the element of the //stack indicated by //stackTop - 1 }//end top template void stackType::pop() { if (!isEmptyStack()) stackTop--; //decrement stackTop else cout << "Cannot remove from an empty stack." << endl; }//end pop template stackType::stackType(int stackSize) { if (stackSize <= 0) { cout << "Size of the array to hold the stack must " << "be positive." << endl; cout << "Creating an array of size 100." << endl; maxStackSize = 100; } else maxStackSize = stackSize; //set the stack size to //the value specified by //the parameter stackSize stackTop = 0; //set stackTop to 0 list = new Type[maxStackSize]; //create the array to //hold the stack elements }//end constructor template stackType::~stackType() //destructor { delete [] list; //deallocate the memory occupied //by the array }//end destructor template void stackType::copyStack(const stackType& otherStack) { delete [] list; maxStackSize = otherStack.maxStackSize; stackTop = otherStack.stackTop; list = new Type[maxStackSize]; //copy otherStack into this stack for (int j = 0; j < stackTop; j++) list[j] = otherStack.list[j]; } //end copyStack template stackType::stackType(const stackType& otherStack) { list = nullptr; copyStack(otherStack); }//end copy constructor template const stackType& stackType::operator= (const stackType& otherStack) { if (this != &otherStack) //avoid self-copy copyStack(otherStack); return *this; } //end operator= #endif stackADT.h //Header file: stackADT.h #ifndef H_StackADT #define H_StackADT template class stackADT { public: virtual void initializeStack() = 0; virtual bool isEmptyStack() const = 0; virtual bool isFullStack() const = 0; virtual void push(const Type& newItem) = 0; virtual Type top() const = 0; virtual void pop() = 0; }; #endif I need Main.cpp #include #include "myStack.h" using namespace std; int main() { // Write your main here return 0; }
Instructions C++ a. Add the following operation to the class stackType. void reverseStack(stackType &otherStack); This operation copies the elements of a stack in reverse order onto another stack. Consider the following statements: stackType stack1; stackType stack2; The statement stack1.reverseStack(stack2); copies the elements of stack1 onto stack2 in reverse order. That is, the top element of stack1 is the bottom element of stack2, and so on. The old contents of stack2 are destroyed, and stack1 is unchanged. b. Write the definition of the function template to implement the operation reverseStack. Write a program to test the class stackType. myStack.h //Header file: myStack.h #ifndef H_StackType #define H_StackType #include #include #include "stackADT.h" using namespace std; template class stackType: public stackADT { public: const stackType& operator=(const stackType&); void initializeStack(); bool isEmptyStack() const; bool isFullStack() const; void push(const Type& newItem); Type top() const; void pop(); stackType(int stackSize = 100); stackType(const stackType& otherStack); ~stackType(); void reverseStack(stackType &otherStack); private: int maxStackSize; int stackTop; Type *list; void copyStack(const stackType& otherStack); }; template void stackType::initializeStack() { stackTop = 0; }//end initializeStack template bool stackType::isEmptyStack() const { return(stackTop == 0); }//end isEmptyStack template bool stackType::isFullStack() const { return(stackTop == maxStackSize); } //end isFullStack template void stackType::push(const Type& newItem) { if (!isFullStack()) { list[stackTop] = newItem; //add newItem to the //top of the stack stackTop++; //increment stackTop } else cout << "Cannot add to a full stack." << endl; }//end push template Type stackType::top() const { assert(stackTop != 0); //if stack is empty, //terminate the program return list[stackTop - 1]; //return the element of the //stack indicated by //stackTop - 1 }//end top template void stackType::pop() { if (!isEmptyStack()) stackTop--; //decrement stackTop else cout << "Cannot remove from an empty stack." << endl; }//end pop template stackType::stackType(int stackSize) { if (stackSize <= 0) { cout << "Size of the array to hold the stack must " << "be positive." << endl; cout << "Creating an array of size 100." << endl; maxStackSize = 100; } else maxStackSize = stackSize; //set the stack size to //the value specified by //the parameter stackSize stackTop = 0; //set stackTop to 0 list = new Type[maxStackSize]; //create the array to //hold the stack elements }//end constructor template stackType::~stackType() //destructor { delete [] list; //deallocate the memory occupied //by the array }//end destructor template void stackType::copyStack(const stackType& otherStack) { delete [] list; maxStackSize = otherStack.maxStackSize; stackTop = otherStack.stackTop; list = new Type[maxStackSize]; //copy otherStack into this stack for (int j = 0; j < stackTop; j++) list[j] = otherStack.list[j]; } //end copyStack template stackType::stackType(const stackType& otherStack) { list = nullptr; copyStack(otherStack); }//end copy constructor template const stackType& stackType::operator= (const stackType& otherStack) { if (this != &otherStack) //avoid self-copy copyStack(otherStack); return *this; } //end operator= #endif stackADT.h //Header file: stackADT.h #ifndef H_StackADT #define H_StackADT template class stackADT { public: virtual void initializeStack() = 0; virtual bool isEmptyStack() const = 0; virtual bool isFullStack() const = 0; virtual void push(const Type& newItem) = 0; virtual Type top() const = 0; virtual void pop() = 0; }; #endif I need Main.cpp #include #include "myStack.h" using namespace std; int main() { // Write your main here return 0; }
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 1TF
Related questions
Question
Instructions C++
a. Add the following operation to the class stackType.
void reverseStack(stackType<Type> &otherStack);This operation copies the elements of a stack in reverse order onto another stack.
Consider the following statements:
stackType<int> stack1;stackType<int> stack2;
The statement
stack1.reverseStack(stack2);copies the elements of stack1 onto stack2 in reverse order. That is, the top element of stack1 is the bottom element of stack2, and so on. The old contents of stack2 are destroyed, and stack1 is unchanged.
b. Write the definition of the function template to implement the operation reverseStack.
Write a program to test the class stackType.
myStack.h
//Header file: myStack.h
#ifndef H_StackType
#define H_StackType
#include <iostream>
#include <cassert>
#include "stackADT.h"
using namespace std;
template <class Type>
class stackType: public stackADT<Type>
{
public:
const stackType<Type>& operator=(const stackType<Type>&);
void initializeStack();
bool isEmptyStack() const;
bool isFullStack() const;
void push(const Type& newItem);
Type top() const;
void pop();
stackType(int stackSize = 100);
stackType(const stackType<Type>& otherStack);
~stackType();
void reverseStack(stackType<Type> &otherStack);
private:
int maxStackSize;
int stackTop;
Type *list;
void copyStack(const stackType<Type>& otherStack);
};
template <class Type>
void stackType<Type>::initializeStack()
{
stackTop = 0;
}//end initializeStack
template <class Type>
bool stackType<Type>::isEmptyStack() const
{
return(stackTop == 0);
}//end isEmptyStack
template <class Type>
bool stackType<Type>::isFullStack() const
{
return(stackTop == maxStackSize);
} //end isFullStack
template <class Type>
void stackType<Type>::push(const Type& newItem)
{
if (!isFullStack())
{
list[stackTop] = newItem; //add newItem to the
//top of the stack
stackTop++; //increment stackTop
}
else
cout << "Cannot add to a full stack." << endl;
}//end push
template <class Type>
Type stackType<Type>::top() const
{
assert(stackTop != 0); //if stack is empty,
//terminate the program
return list[stackTop - 1]; //return the element of the
//stack indicated by
//stackTop - 1
}//end top
template <class Type>
void stackType<Type>::pop()
{
if (!isEmptyStack())
stackTop--; //decrement stackTop
else
cout << "Cannot remove from an empty stack." << endl;
}//end pop
template <class Type>
stackType<Type>::stackType(int stackSize)
{
if (stackSize <= 0)
{
cout << "Size of the array to hold the stack must "
<< "be positive." << endl;
cout << "Creating an array of size 100." << endl;
maxStackSize = 100;
}
else
maxStackSize = stackSize; //set the stack size to
//the value specified by
//the parameter stackSize
stackTop = 0; //set stackTop to 0
list = new Type[maxStackSize]; //create the array to
//hold the stack elements
}//end constructor
template <class Type>
stackType<Type>::~stackType() //destructor
{
delete [] list; //deallocate the memory occupied
//by the array
}//end destructor
template <class Type>
void stackType<Type>::copyStack(const stackType<Type>& otherStack)
{
delete [] list;
maxStackSize = otherStack.maxStackSize;
stackTop = otherStack.stackTop;
list = new Type[maxStackSize];
//copy otherStack into this stack
for (int j = 0; j < stackTop; j++)
list[j] = otherStack.list[j];
} //end copyStack
template <class Type>
stackType<Type>::stackType(const stackType<Type>& otherStack)
{
list = nullptr;
copyStack(otherStack);
}//end copy constructor
template <class Type>
const stackType<Type>& stackType<Type>::operator=
(const stackType<Type>& otherStack)
{
if (this != &otherStack) //avoid self-copy
copyStack(otherStack);
return *this;
} //end operator=
#endif
stackADT.h//Header file: stackADT.h
#ifndef H_StackADT
#define H_StackADT
template <class Type>
class stackADT
{
public:
virtual void initializeStack() = 0;
virtual bool isEmptyStack() const = 0;
virtual bool isFullStack() const = 0;
virtual void push(const Type& newItem) = 0;
virtual Type top() const = 0;
virtual void pop() = 0;
};
#endif
I need Main.cpp
#include <iostream>
#include "myStack.h"
using namespace std;
int main() {
// Write your main here
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
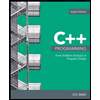
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
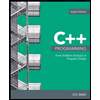
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning