Instructions: Put the class definition in EV.h and the implementation of the constructors and functions in EV.cpp 1. Define a class called "EV", that represents the information of an electric vehicle. The EV is defined with these attributes: model (string), range(int), unit (string) and year (int). Functions of the EV class must perform the following operations: A default constructor which sets all the numeric instance variables to zero. A constructor with 4 parameters which sets the 4 instance variables to the corresponding values passed. Implement a getter function for each of the 4 instance v
Instructions: Put the class definition in EV.h and the implementation of the constructors and functions in EV.cpp 1. Define a class called "EV", that represents the information of an electric vehicle. The EV is defined with these attributes: model (string), range(int), unit (string) and year (int). Functions of the EV class must perform the following operations: A default constructor which sets all the numeric instance variables to zero. A constructor with 4 parameters which sets the 4 instance variables to the corresponding values passed. Implement a getter function for each of the 4 instance v
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please solve this C++ program as per ALL the instructions!!(Please read all the instructions and make sure to input the correct output as shown at the end of the pictures. Thank you for your help!
(NOTE: DO NOT verify the validity of user input. You are supposed to use the POINTERS at different parts of the code as instructed Please do not write it any other way even if the output is same as shown.
Instructions:
Put the class definition in EV.h and the implementation of the constructors and functions in EV.cpp
1. Define a class called "EV", that represents the information of an electric vehicle. The EV is defined with these attributes: model (string), range(int), unit (string) and year (int). Functions of the EV class must perform the following operations:
- A default constructor which sets all the numeric instance variables to zero.
- A constructor with 4 parameters which sets the 4 instance variables to the corresponding values passed.
- Implement a getter function for each of the 4 instance variables that will return the value of the instance variable. For example, the getX() function for the instance variable model must be called getModel().
- Implement a setter function for each instance variable that will assign to the instance variable to the value passed. For example, the setX() function for the instance variable model must be called setModel().
- An info function: this function print information of object as following format:
"EV"+[model]+ "manufactured in" + [year] + "has a range of" + [range] + [unit] - A Convert function: This function converts the range from kilometers to miles and from miles to kilometers and returns the result. If the user enters "miles" for the unit and the range in kilometers, then this function must return the miles. As same if user enters 'miles' for the unit and the range in miles, it must return the kilo-meter value. Using the two equations below:
Equation 1: miles= kilometers* 1.609
Equation 2: kilometers= miles* 0.6214 -
A Compare function: This function has one input parameter which is a pointer to an EV object and prints a message according to following criteria:
o If the range of first object (the object that the Compare function is called from) is greater than second object (input parameter in Compare, the program prints:
info of first object (using info function) + new line + "drives longer distance on a single charge than" + new line + print info of second object.
o If the range of first object is less than second object, the program prints:
info of first object (using info function) + new line + "drives shorter distance on a single charge than" + new line + print info of second object.
o If the range of first object is equal to second object, the program prints:
info of first object + new line + "drives the same distance on a single charge as" +new line + print info of second object.
2. Driver Class (EV_driver.cpp)
Create a file EV_driver.cpp with the main function that asks the user to enter the information of two EV objects and it dynamically creates two objects of type EV. Then, using Convert, Info and Compare functions, the following results are displayed: (Text in green is user input)
(Please include the text of 3 code files : EV.h, EV.cpp, EV_driver.cpp as well as screenshots of the output.
![Put the class definition in EV.h and the implementation of the constructors and functions in EV.cpp
O
(2
1. Define a class called "EV", that represents the information of an electric vehicle. The EV is defined with these
attributes: model (string), range(int), unit (string) and year (int). Functions of the EV class must perform
the following operations:
1. A default constructor which sets all the numeric instance variables to zero.
2. A constructor with 4 parameters which sets the 4 instance variables to the corresponding values passed.
3. Implement a getter function for each of the 4 instance variables that will return the value of the instance
variable. For example, the getX() function for the instance variable model must be called
getModel().
4. Implement a setter function for each instance variable that will assign to the instance variable to the
value passed. For example, the setX() function for the instance variable model must be called
setModel().
5. An info function: this function print information of object as following format:
"EV" + [model]+ "manufactured in" + [year] + "has a range of" + [range] + [unit]
6. A Convert function: This function converts the range from kilometers to miles and from miles
to kilometers and returns the result. If the user enters "miles" for the unit and the range in
Kometers, then this function must return the miles. As same if user enters 'miles for the unit and
the range in miles, it must return the kilometer value. Using the two equations below:
miles = kilometers *1.609
kilometers ailes * 0.6214
$
7.) A Compare function: This function has one input parameter which is a pointer to an EV object and
prints a message according to following criteria:
o If the range of first object (the object that the Compare function is called from) is greater than
second object (input parameter in Compare, the program prints:
info of first object (using info function) + new line + "drives longer distance on a single charge
than"+new line + print info of second object.
If the range of first object is less than second object, the program prints:
info of first object (using info function) + new line + "drives shorter distance on a single charge
than"+new line + print info of second object.
O
O
Equation 1
Equation 2
If the range of first object is equal to second object, the program prints:
info of first object + new line + "drives the same distance on a single charge as" + new line
print info of second object.
Driver Class (EV_driver.cpp) (3)
Create a file EV_driver.cpp with the main function that asks the user to enter the information of two EV
objects and it dynamically creates two objects of type EV. Then, using Convert, Info and Compare
functions, the following results are displayed: (Text in green is user input)
Load first EV
Enter EV Model: Tesla Model 3.
Enterar manufactured: 2019.J
Enter range unit (Miles or Kilometers) : Kilometers.
You choose Kilometers, so please enter range in kilometers: 400.
Load second EV
Enter EV Model: Model Y
Enter year manufactured: 2021.J
Enter range unit (Miles or Kilometers): Miles.
You choose Miles, so please enter range in miles: 300.J
The EV Tesla Model 3 manufactured in 2019 has a range of 400 kilometers
drives shorter distance on a single charge than
The EV Tesla Model Y manufactured in 2021 has a range of 300 miler](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd5ee8f6f-b196-4b10-99ae-82f5314436a3%2F59cd630f-0c9b-4401-839c-033f5cc48af0%2F48uxvq_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Put the class definition in EV.h and the implementation of the constructors and functions in EV.cpp
O
(2
1. Define a class called "EV", that represents the information of an electric vehicle. The EV is defined with these
attributes: model (string), range(int), unit (string) and year (int). Functions of the EV class must perform
the following operations:
1. A default constructor which sets all the numeric instance variables to zero.
2. A constructor with 4 parameters which sets the 4 instance variables to the corresponding values passed.
3. Implement a getter function for each of the 4 instance variables that will return the value of the instance
variable. For example, the getX() function for the instance variable model must be called
getModel().
4. Implement a setter function for each instance variable that will assign to the instance variable to the
value passed. For example, the setX() function for the instance variable model must be called
setModel().
5. An info function: this function print information of object as following format:
"EV" + [model]+ "manufactured in" + [year] + "has a range of" + [range] + [unit]
6. A Convert function: This function converts the range from kilometers to miles and from miles
to kilometers and returns the result. If the user enters "miles" for the unit and the range in
Kometers, then this function must return the miles. As same if user enters 'miles for the unit and
the range in miles, it must return the kilometer value. Using the two equations below:
miles = kilometers *1.609
kilometers ailes * 0.6214
$
7.) A Compare function: This function has one input parameter which is a pointer to an EV object and
prints a message according to following criteria:
o If the range of first object (the object that the Compare function is called from) is greater than
second object (input parameter in Compare, the program prints:
info of first object (using info function) + new line + "drives longer distance on a single charge
than"+new line + print info of second object.
If the range of first object is less than second object, the program prints:
info of first object (using info function) + new line + "drives shorter distance on a single charge
than"+new line + print info of second object.
O
O
Equation 1
Equation 2
If the range of first object is equal to second object, the program prints:
info of first object + new line + "drives the same distance on a single charge as" + new line
print info of second object.
Driver Class (EV_driver.cpp) (3)
Create a file EV_driver.cpp with the main function that asks the user to enter the information of two EV
objects and it dynamically creates two objects of type EV. Then, using Convert, Info and Compare
functions, the following results are displayed: (Text in green is user input)
Load first EV
Enter EV Model: Tesla Model 3.
Enterar manufactured: 2019.J
Enter range unit (Miles or Kilometers) : Kilometers.
You choose Kilometers, so please enter range in kilometers: 400.
Load second EV
Enter EV Model: Model Y
Enter year manufactured: 2021.J
Enter range unit (Miles or Kilometers): Miles.
You choose Miles, so please enter range in miles: 300.J
The EV Tesla Model 3 manufactured in 2019 has a range of 400 kilometers
drives shorter distance on a single charge than
The EV Tesla Model Y manufactured in 2021 has a range of 300 miler
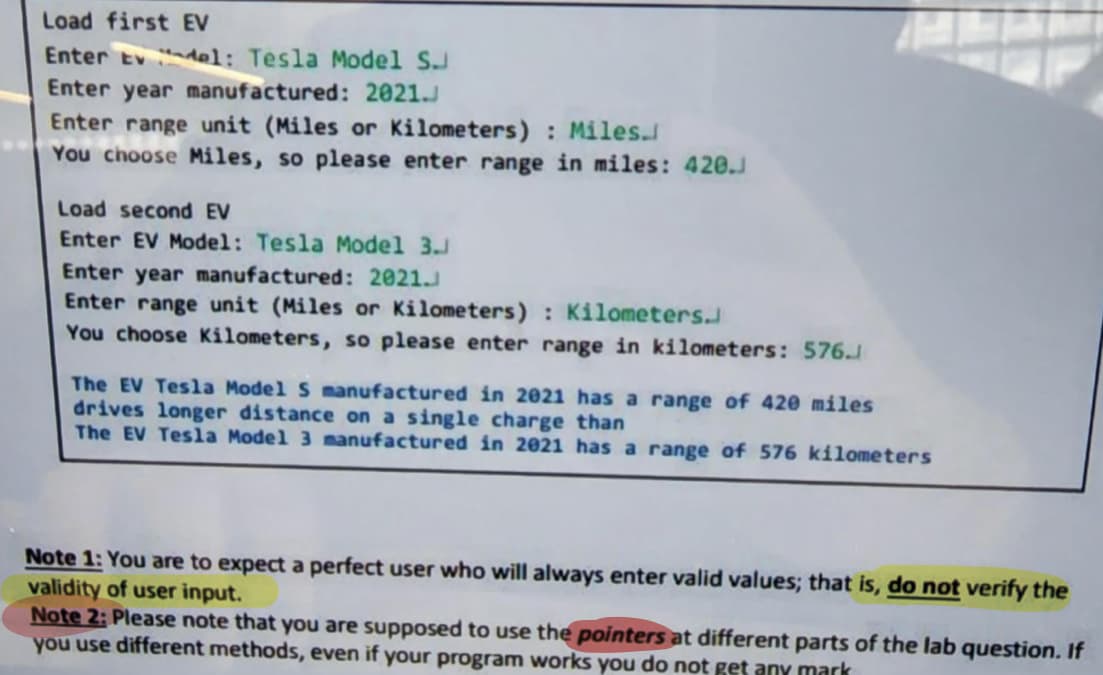
Transcribed Image Text:Load first EV
Enter Evdel: Tesla Model S.
Enter year manufactured: 2021.J
Enter range unit (Miles or Kilometers): Miles.l
You choose Miles, so please enter range in miles: 420.J
Load second EV
Enter EV Model: Tesla Model 3.
Enter year manufactured: 2021.
Enter range unit (Miles or Kilometers): Kilometers.
You choose Kilometers, so please enter range in kilometers: 576.
The EV Tesla Model S manufactured in 2021 has a range of 420 miles
drives longer distance on a single charge than
The EV Tesla Model 3 manufactured in 2021 has a range of 576 kilometers
Note 1: You are to expect a perfect user who will always enter valid values; that is, do not verify the
validity of user input.
Note 2: Please note that you are supposed to use the pointers at different parts of the lab question. If
you use different methods, even if your program works you do not get any mark
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
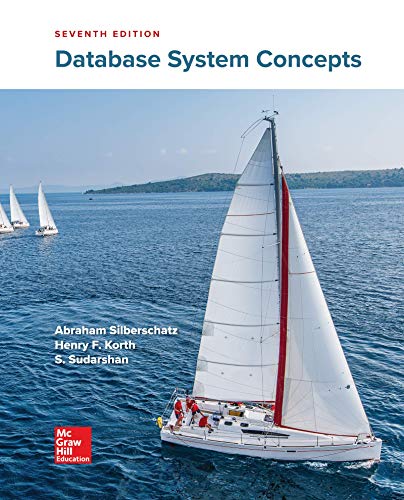
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
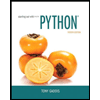
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
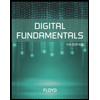
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
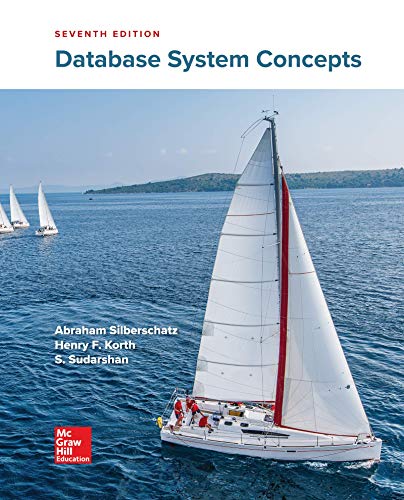
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
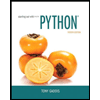
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
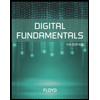
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
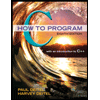
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
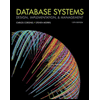
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
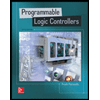
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education