Instructions: Turn all instances of classes into pointers. You will also need to combine the player and vector into one vector objects and fix all issues this causes. #ifndef ITEM_H #define ITEM_H class Item { public: Item() {}; enum class Type { sword, armor, shield, numTypes }; Item(Type classification, int bonusValue); Type getClassification() const; int getBonusValue() const; private: Type classification{ Type::numTypes }; int bonusValue{ 0 }; }; std::ostream& operator<< (std::ostream& o, const Item& src); bool operator< (const Item& srcL, const Item& srcR); int& operator+=(int& srcL, const Item& srcR); #endif // !ITEM_H #ifndef MONSTER_H #define MONSTER_H #include "Object.h" class Player; class Monster : public Object { public: Monster() {}; Monster(const Player& player); void update(Player& player, std::vector& monsters) override; int attack() const override; void defend(int damage) override; void print(std::ostream& o) const override; private: int AC{ 0 }; }; #endif // !MONSTER_H #ifndef OBJECT_H #define OBJECT_H #include #include class Player; class Monster; class Object { public: static std::random_device seed; static std::default_random_engine engine; static bool nameOnly; enum class Type { player, slime, orc, sprite, dragon, numTypes }; Object() {} Object(Type name, int strength, int health, int level); virtual int attack() const = 0; virtual void defend(int damage) = 0; virtual void update(Player& player, std::vector& monsters) = 0; virtual void print(std::ostream& o) const; bool isDead(); Type getName() const; int getLevel() const; int getHealth() const; protected: int damageDone(int modification) const; int damageTaken(int damageDone, int AC); Type name{ Type::numTypes }; int strength{ 0 }; int health{ 0 }; int level{ 0 }; }; std::ostream& operator<< (std::ostream& o, const Object& src); #endif // !OBJECT_H #ifndef PLAYER_H #define PLAYER_H #include "Object.h" #include "Item.h" class Player : public Object { public: Player(); void levelUp(); void update(Player& player, std::vector& monsters) override; int getLevel() const; int getSP() const; int attack() const override; void defend(int damage) override; void print(std::ostream& o) const override; std::map getInventory() const; void heal(); private: std::map inventory; int SP{ 0 }; }; std::ostream& operator<< (std::ostream& o, const std::map& src); #endif // !PLAYER_H
Instructions: Turn all instances of classes into pointers. You will also need to combine the player and

Step by step
Solved in 3 steps

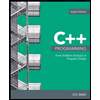
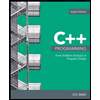