interleaved Write a function interleaved that accepts two sorted sequences of numbers and returns a sorted sequence of all numbers obtained by interleaving the two sequences. Guidelines: • each argument is a list • assume that each argument is sorted in non-decreasing order (goes up or stays the same, never goes down) • you are not allowed to use sort or sorted (or any other form of sorting) in your solution • you must interleave by iterating over the two sequences simultaneously, choosing the smallest current number from each sequence.
![interleaved
Write a function interleaved that accepts two sorted sequences of numbers and returns a
sorted sequence of all numbers obtained by interleaving the two sequences. Guidelines:
• each argument is a list
• assume that each argument is sorted in non-decreasing order (goes up or stays the same,
never goes down)
• you are not allowed to use sort or sorted (or any other form of sorting) in your solution
• you must interleave by iterating over the two sequences simultaneously, choosing the
smallest current number from each sequence.
Sample usage:
1
>>> interleaved( [-7, -2, -1], [-4, 0, 4, 8])
2
[-7, -4, -2, -1, 0, 4, 8]
3
>>> interleaved( [-4, 0, 4, 8], [-7, -2, -1])
4
[-7, -4, -2, -1, 0, 4, 8]
>>> interleaved( [-8, 4, 4, 5, 6, 6, 6, 9, 9], [-6, -2, 3, 4, 4, 5, 6, 7,
8])
[-8, -6, -2, 3, 4, 4, 4, 4, 5, 5, 6, 6, 6, 6, 7, 8, 9, 9]
7
>>> interleaved( [-3, -2, 0, 2, 2, 2, 3, 3, 3], [-3, -2, 2, 3])
8
[-3, -3, -2, -2, 0, 2, 2, 2, 2, 3, 3, 3, 3]
9.
>>> interleaved( [-3, -2, 2, 3], [-3, -2, 0, 2, 2, 2, 3, 3, 3])
10
[-3, -3, -2, -2, 0, 2, 2, 2, 2, 3, 3, 3, 3]
11
>>> interleaved([1,2,2],[])
12
[1, 2, 2]
13
>>> interleaved([],[1,2,2])
14
[1, 2, 2]
15
>>> interleaved ([],[])
16
[]
17
>>> interleaved( list(range(-2,12,3)), list(range (20,50,5)) )
18
[-2, 1, 4, 7, 10, 20, 25, 30, 35, 40, 45]
19
>>> interleaved( list(range (20,50,5)), list(range(-2,12,3)) )==[-2, 1, 4,
7, 10, 20, 25, 30, 35, 40, 45]
20
True](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc4b85f9b-2931-43f1-91f6-17c8a9947553%2Fda6918c0-0b08-4a2d-8c58-e26036165735%2Fu4fsi2_processed.jpeg&w=3840&q=75)

#function interleaved takes two sorted lists, then returns a sorted sequence of all numbers by interleaving the two sequences
def interleaved(l1,l2):
i=j=0#i is used to traverse l1, j is used to traverse l2
l3=[]#new empty list to store sorted sequence of all numbers in both arrays
while i<len(l1) and j<len(l2):#runs until one of the index counter reaches its arrays length
if l1[i]<=l2[j]:#if current element of l1 is smaller than l2's element
l3=l3+[l1[i]]#then adding current element of l1 and leaving element of l2
i+=1 #moving index of l1
elif l2[j]<l1[i]:#if current element of l2 is smaller than l1's element
l3=l3+[l2[j]]#then adding current element of l2 and leaving element of l1
j+=1 #moving index of l2
#since one of the counter will reach the size of its array
#we must add all elements remaining in the other array
while i<len(l1):#adding all remaining elements of l1 to l3
l3=l3+[l1[i]]
i+=1
while j<len(l2):#adding all remaining elements of l2 to l3
l3=l3+[l2[j]]
j+=1
#finally returning sorted l3
return l3
#testing above method
print(interleaved([-7,-2,-1],[-4,0,4,8]))
print(interleaved([-4,0,4,8],[-7,-2,-1]))
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

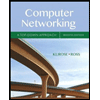
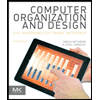
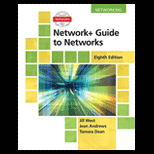
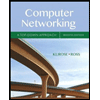
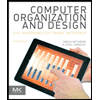
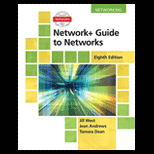
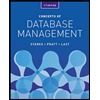
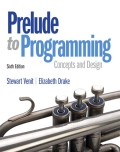
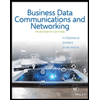