It's time to put your Java knowledge to use. Create a basic version of rock- paper-scissors that allows users to play against the computer in the console. The game consists of a few main features: • Play rock-paper-scissors against a computer player. Play rock-paper-scissors against a human player. Hint: Use a random number generator to make the computer's choice. . Feature Requirements Your game must: • Have a main menu with options to enter 2 players or vs. computer. . If the user enters 2 players, they should be able to play rock-paper- scissors against a human competitor. . If the user enters vs. computer, they should be able to play against the computer. • When the game is over, the winner should be declared. Technical Requirements • Use classes to remove repetitive parts of code, and create an abstract Player class to manage the player's state (if they won or lost, how many points they have, what move they made). In addition, interfaces should be used in places where they are necessary. • Handle invalid user input. • Handle incorrect capitalization of otherwise valid user input ("rock," "Rock," "Rock," "ROCK," and more). • Each class (including a Player class) should have methods associated with it and be instantiated as an object (as opposed to a singleton or an interface). € • Use public, private, and static variables, methods, and members within each class appropriately. Technical Requirements • Use classes to remove repetitive parts of code, and create an abstract Player class to manage the player's state (if they won or lost, how many points they have, what move they made). In addition, interfaces should be used in places where they are necessary. • Handle invalid user input. • Handle incorrect capitalization of otherwise valid user input ("rock," "Rock," "Rock," "ROCK," and more). • Each class (including a Player class) should have methods associated with it and be instantiated as an object (as opposed to a singleton or an interface). • Use public, private, and static variables, methods, and members within each class appropriately. • Incorporate exception handling to make sure your game crashes gracefully if it receives bad input. • Get standard input with Java using a Scanner, or use Processing to get mouse, keyboard, or other input. • Use arrays or array lists to store game history (if applicable). Bonus Ideas • Write automated JUnit tests for your application. • Use an Agile project management framework for your game. . If the user enters history, the program should display previous game history (winner's name, game date, and more). • Use Java packages to modularize code. Place any helper tools in these packages - they could be related input, networking, or graphics. • Use Maven to install external dependencies your game might require. • Use generics on collections such as arrays and array lists to store different data composed of different types. • Use multithreading to handle concurrent requests (like in multiplayer games). €
It's time to put your Java knowledge to use. Create a basic version of rock- paper-scissors that allows users to play against the computer in the console. The game consists of a few main features: • Play rock-paper-scissors against a computer player. Play rock-paper-scissors against a human player. Hint: Use a random number generator to make the computer's choice. . Feature Requirements Your game must: • Have a main menu with options to enter 2 players or vs. computer. . If the user enters 2 players, they should be able to play rock-paper- scissors against a human competitor. . If the user enters vs. computer, they should be able to play against the computer. • When the game is over, the winner should be declared. Technical Requirements • Use classes to remove repetitive parts of code, and create an abstract Player class to manage the player's state (if they won or lost, how many points they have, what move they made). In addition, interfaces should be used in places where they are necessary. • Handle invalid user input. • Handle incorrect capitalization of otherwise valid user input ("rock," "Rock," "Rock," "ROCK," and more). • Each class (including a Player class) should have methods associated with it and be instantiated as an object (as opposed to a singleton or an interface). € • Use public, private, and static variables, methods, and members within each class appropriately. Technical Requirements • Use classes to remove repetitive parts of code, and create an abstract Player class to manage the player's state (if they won or lost, how many points they have, what move they made). In addition, interfaces should be used in places where they are necessary. • Handle invalid user input. • Handle incorrect capitalization of otherwise valid user input ("rock," "Rock," "Rock," "ROCK," and more). • Each class (including a Player class) should have methods associated with it and be instantiated as an object (as opposed to a singleton or an interface). • Use public, private, and static variables, methods, and members within each class appropriately. • Incorporate exception handling to make sure your game crashes gracefully if it receives bad input. • Get standard input with Java using a Scanner, or use Processing to get mouse, keyboard, or other input. • Use arrays or array lists to store game history (if applicable). Bonus Ideas • Write automated JUnit tests for your application. • Use an Agile project management framework for your game. . If the user enters history, the program should display previous game history (winner's name, game date, and more). • Use Java packages to modularize code. Place any helper tools in these packages - they could be related input, networking, or graphics. • Use Maven to install external dependencies your game might require. • Use generics on collections such as arrays and array lists to store different data composed of different types. • Use multithreading to handle concurrent requests (like in multiplayer games). €
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
hello please code this for me in java.. and please make sure that its meets the requirements in the image and also matches the sample output
also please provide some comments.. please follow the instructions as it is mentioned in the image
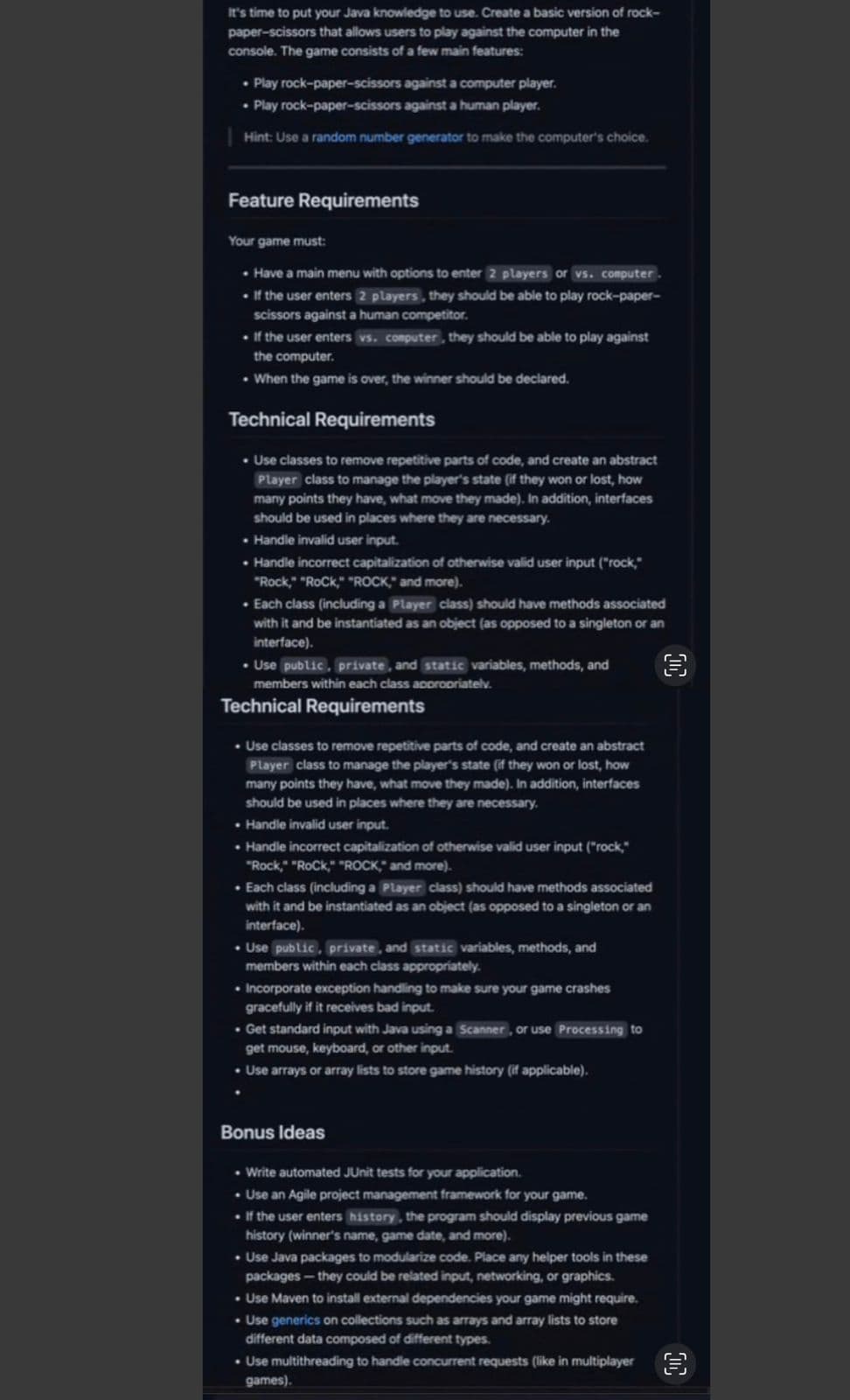
Transcribed Image Text:It's time to put your Java knowledge to use. Create a basic version of rock-
paper-scissors that allows users to play against the computer in the
console. The game consists of a few main features:
• Play rock-paper-scissors against a computer player.
• Play rock-paper-scissors against a human player.
Hint: Use a random number generator to make the computer's choice.
Feature Requirements
Your game must:
• Have a main menu with options to enter 2 players or vs. computer.
. If the user enters 2 players, they should be able to play rock-paper-
scissors against a human competitor.
. If the user enters vs. computer, they should be able to play against
the computer.
• When the game is over, the winner should be declared.
Technical Requirements
• Use classes to remove repetitive parts of code, and create an abstract
Player class to manage the player's state (if they won or lost, how
many points they have, what move they made). In addition, interfaces
should be used in places where they are necessary.
• Handle invalid user input.
• Handle incorrect capitalization of otherwise valid user input ("rock,"
"Rock," "Rock," "ROCK," and more).
• Each class (including a Player class) should have methods associated
with it and be instantiated as an object (as opposed to a singleton or an
interface).
€
• Use public, private, and static variables, methods, and
members within each class appropriately.
Technical Requirements
• Use classes to remove repetitive parts of code, and create an abstract
Player class to manage the player's state (if they won or lost, how
many points they have, what move they made). In addition, interfaces
should be used in places where they are necessary.
• Handle invalid user input.
• Handle incorrect capitalization of otherwise valid user input ("rock,"
"Rock," "Rock," "ROCK," and more).
• Each class (including a Player class) should have methods associated
with it and be instantiated as an object (as opposed to a singleton or an
interface).
• Use public, private, and static variables, methods, and
members within each class appropriately.
• Incorporate exception handling to make sure your game crashes
gracefully if it receives bad input.
• Get standard input with Java using a Scanner, or use Processing to
get mouse, keyboard, or other input.
• Use arrays or array lists to store game history (if applicable).
Bonus Ideas
• Write automated JUnit tests for your application.
• Use an Agile project management framework for your game.
• If the user enters history, the program should display previous game
history (winner's name, game date, and more).
• Use Java packages to modularize code. Place any helper tools in these
packages - they could be related input, networking, or graphics.
• Use Maven to install external dependencies your game might require.
• Use generics on collections such as arrays and array lists to store
different data composed of different types.
• Use multithreading to handle concurrent requests (like in multiplayer
games).
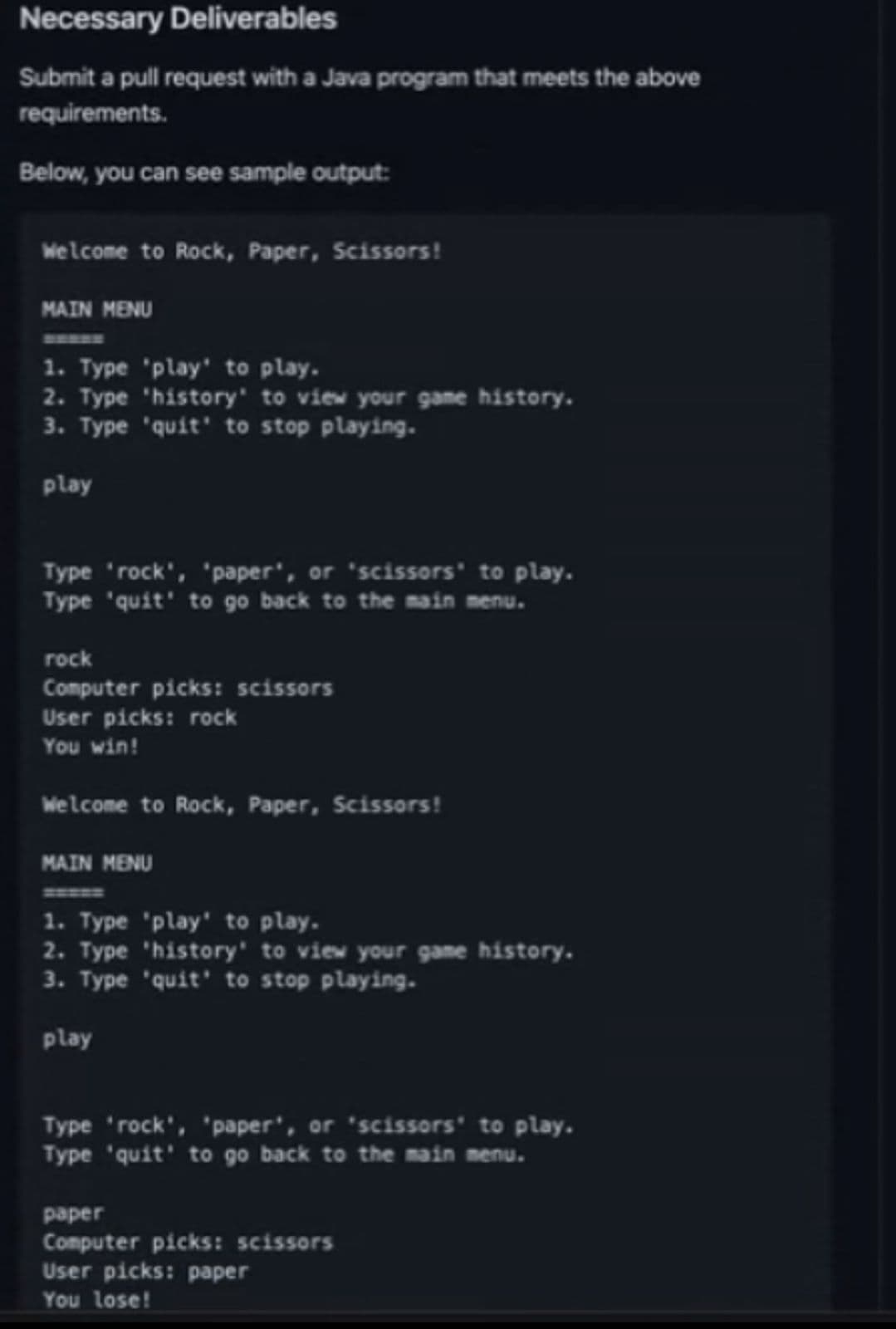
Transcribed Image Text:Necessary Deliverables
Submit a pull request with a Java program that meets the above
requirements.
Below, you can see sample output:
Welcome to Rock, Paper, Scissors!
MAIN MENU
1. Type 'play' to play.
2. Type 'history' to view your game history.
3. Type 'quit' to stop playing.
play
Type 'rock', 'paper', or 'scissors' to play.
Type 'quit' to go back to the main menu.
rock
Computer picks: scissors
User picks: rock
You win!
Welcome to Rock, Paper, Scissors!
MAIN MENU
1. Type 'play' to play.
2. Type 'history' to view your game history.
3. Type 'quit' to stop playing.
play
Type 'rock', 'paper', or 'scissors' to play.
Type 'quit' to go back to the main menu.
paper
Computer picks: scissors
User picks: paper
You lose!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
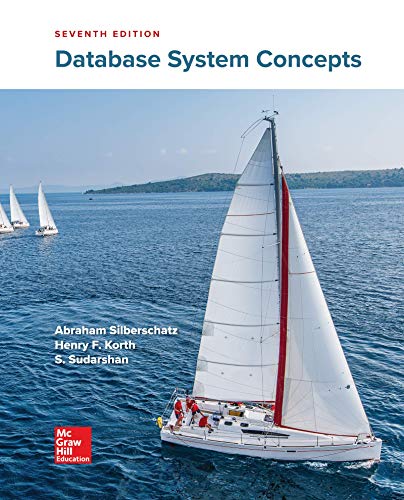
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
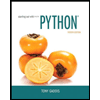
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
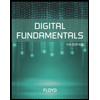
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
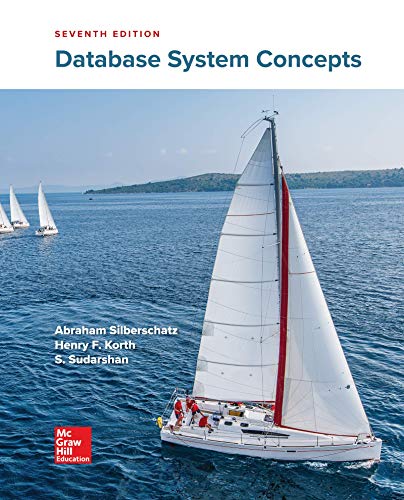
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
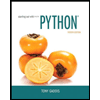
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
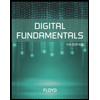
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
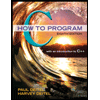
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
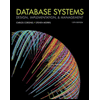
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
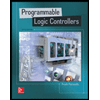
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education