Java Files Recursive.java RecursiveDemo.java The purpose of the assignment is to practice writing methods that are recursive. We will write four methods each is worth 15 points. a- int sum_sqr_rec(stack stk) which will receive a stack of "int" and output the sum of the squares of the elements in the stack. b- int plus_minus_rec(stack stk) which will receive a stack of "int" (example: {a,b,c,d,e,f,g,h,i,j}) and output the sum of the elements in the stack as follows: a - b + c - d + e - f + g - h + i -j c- void prt_chars_rev_rec(stack stk) which will receive a stack of "char" and print its elements in reverse. d- void prt_chars_rec(queue stk) which will receive a queue of "char" and print its elements Remember to use the stack and queue STL. The Assignment will require you to create 2 files: Recursive.java which contains the details of creating the 4 methods as specified above: int sum_sqr_rec(stack stk), (15 points) int plus_minus_rec(stack stk), (15 points) void prt_chars_rev_rec(stack stk), (15 points) void prt_chars_rec(queue stk), (15 points) RecursiveDemo.java which: A- reads a string expression: {(1+2)+[4*(2+3)]} and store the expression in a stack and a queue.(15 points) a- prints the corresponding expression "in reverse" using: prt_chars_rev_rec ( 5 points) b- prints the corresponding expressing "as is" using: prt_chars_rec.( 5 points) B- reads an array of integers: { 1, 2 , 3 , 4 , 5 , 6 , 7 , 8 , 9 , 10 } and store them in a stack of ints.(5 points) Then it: C- prints the sum of the squares of the elements in the stack using int sum_sqr_rec(stack stk) and outputting the value(5 points): 385 D- prints the sum of the elements in the stack using: int plus_minus_rec(stack stk) and outputting the value(5 points): 1 - 2 + 3 - 4 + 5 - 6 + 7 - 8 + 9 - 10 = -5
Java Files Recursive.java RecursiveDemo.java The purpose of the assignment is to practice writing methods that are recursive. We will write four methods each is worth 15 points. a- int sum_sqr_rec(stack stk) which will receive a stack of "int" and output the sum of the squares of the elements in the stack. b- int plus_minus_rec(stack stk) which will receive a stack of "int" (example: {a,b,c,d,e,f,g,h,i,j}) and output the sum of the elements in the stack as follows: a - b + c - d + e - f + g - h + i -j c- void prt_chars_rev_rec(stack stk) which will receive a stack of "char" and print its elements in reverse. d- void prt_chars_rec(queue stk) which will receive a queue of "char" and print its elements Remember to use the stack and queue STL. The Assignment will require you to create 2 files: Recursive.java which contains the details of creating the 4 methods as specified above: int sum_sqr_rec(stack stk), (15 points) int plus_minus_rec(stack stk), (15 points) void prt_chars_rev_rec(stack stk), (15 points) void prt_chars_rec(queue stk), (15 points) RecursiveDemo.java which: A- reads a string expression: {(1+2)+[4*(2+3)]} and store the expression in a stack and a queue.(15 points) a- prints the corresponding expression "in reverse" using: prt_chars_rev_rec ( 5 points) b- prints the corresponding expressing "as is" using: prt_chars_rec.( 5 points) B- reads an array of integers: { 1, 2 , 3 , 4 , 5 , 6 , 7 , 8 , 9 , 10 } and store them in a stack of ints.(5 points) Then it: C- prints the sum of the squares of the elements in the stack using int sum_sqr_rec(stack stk) and outputting the value(5 points): 385 D- prints the sum of the elements in the stack using: int plus_minus_rec(stack stk) and outputting the value(5 points): 1 - 2 + 3 - 4 + 5 - 6 + 7 - 8 + 9 - 10 = -5
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
Java Files
Recursive.java
RecursiveDemo.java
The purpose of the assignment is to practice writing methods that are recursive. We will write four methods each is worth 15 points.
- a- int sum_sqr_rec(stack<int> stk)
- which will receive a stack of "int" and output the sum of the squares of the elements in the stack.
- b- int plus_minus_rec(stack<int> stk)
- which will receive a stack of "int" (example: {a,b,c,d,e,f,g,h,i,j}) and output the sum of the elements in the stack as follows:
- a - b + c - d + e - f + g - h + i -j
- c- void prt_chars_rev_rec(stack<char> stk)
- which will receive a stack of "char" and print its elements in reverse.
- d- void prt_chars_rec(queue<char> stk) which will receive a queue of "char" and print its elements
Remember to use the stack and queue STL.
The Assignment will require you to create 2 files:
- Recursive.java which contains the details of creating the 4 methods as specified above:
- int sum_sqr_rec(stack<int> stk), (15 points)
- int plus_minus_rec(stack<int> stk), (15 points)
- void prt_chars_rev_rec(stack<char> stk), (15 points)
- void prt_chars_rec(queue<char> stk), (15 points)
- RecursiveDemo.java which:
- A- reads a string expression:
- {(1+2)+[4*(2+3)]}
- and store the expression in a stack and a queue.(15 points)
- a- prints the corresponding expression "in reverse" using: prt_chars_rev_rec ( 5 points)
- b- prints the corresponding expressing "as is" using: prt_chars_rec.( 5 points)
- B- reads an array of integers: { 1, 2 , 3 , 4 , 5 , 6 , 7 , 8 , 9 , 10 }
- and store them in a stack of ints.(5 points)
- Then it:
- C- prints the sum of the squares of the elements in the stack using int sum_sqr_rec(stack<int> stk) and outputting the value(5 points):
- 385
- D- prints the sum of the elements in the stack using:
- int plus_minus_rec(stack<int> stk) and outputting the value(5 points):
- 1 - 2 + 3 - 4 + 5 - 6 + 7 - 8 + 9 - 10 = -5
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
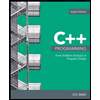
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
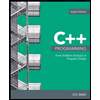
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning