JAVA PROGRAM ASAP Pleasse get rid of that annoying space in the program as shown in the screenhot because it it is not allowing it to pass the freaking test cases once uploaded to hypergrade. Please modify this program even more please ASAP BECAUSE the program down below does not pass all the test cases when I upload it to hypergrade. The program must pass the test case when uploaded to Hypergrade. Chapter 9. PC #16. Morse Code Converter Morse code is a code where each letter of the English alphabet, each digit, and various punctuation characters are represented by a series of dots and dashes. Write a program that asks the user to enter a string, and then converts that string to Morse code and prints on the screen. Use hyphens for dashes and periods for dots. The Morse code table is given in a text file morse.txt. When printing morse code, display eight codes on each line except the last line. Codes should be separated from each other with one space. There should be no extra spaces at the beginning and at the end of the output. Uppercase and lowercase letters are translated the same way. import java.util.HashMap; import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; import java.util.Scanner; public class MorseEncoder { private static final HashMap codeMappings = new HashMap<>(); public static void main(String[] args) { initializeMappings(); Scanner textScanner = new Scanner(System.in); System.out.println("Please enter a string to convert to Morse code:"); String textForEncoding = textScanner.nextLine().toUpperCase(); if ("ENTER".equals(textForEncoding)) { System.out.println(); return; } String encodedOutput = encodeText(textForEncoding); System.out.println(encodedOutput); } private static void initializeMappings() { try (BufferedReader mappingFile = new BufferedReader(new FileReader("morse.txt"))) { String lineContent; while ((lineContent = mappingFile.readLine()) != null) { if (lineContent.length() < 5) { continue; } char alphaChar = lineContent.charAt(0); String morseString = lineContent.substring(4).trim(); codeMappings.put(alphaChar, morseString); } } catch (IOException ioEx) { ioEx.printStackTrace(); } } private static String encodeText(String textForEncoding) { StringBuilder encodedStringBuilder = new StringBuilder(); boolean lastCharWasSpace = false; for (char individualChar : textForEncoding.toCharArray()) { if (individualChar == ' ') { if (!lastCharWasSpace) { encodedStringBuilder.append(" "); lastCharWasSpace = true; } continue; } lastCharWasSpace = false; String morseSymbol = codeMappings.get(individualChar); if (morseSymbol != null) { encodedStringBuilder.append(morseSymbol).append(' '); } } return encodedStringBuilder.toString().trim(); } } Morse.txt 0 ----- 1 .---- 2 ..--- 3 ...-- 4 ....- 5 ..... 6 -.... 7 --... 8 ---.. 9 ----. , --..-- . .-.-.- ? ..--.. A .- B -... C -.-. D -.. E . F ..-. G --. H .... I .. J .--- K -.- L .-.. M -- N -. O --- P .--. Q --.- R .-. S ... T - U ..- V ...- W .-- X -..- Y -.-- Z --.. Test Case 1 Please enter a string to convert to Morse code:\n ENTER \n Test Case 2 Please enter a string to convert to Morse code:\n abcENTER .- -... -.-. \n Test Case 3 Please enter a string to convert to Morse code:\n This is a sample string 1234.ENTER
JAVA PROGRAM ASAP
Pleasse get rid of that annoying space in the program as shown in the screenhot because it it is not allowing it to pass the freaking test cases once uploaded to hypergrade. Please modify this program even more please ASAP BECAUSE the program down below does not pass all the test cases when I upload it to hypergrade. The program must pass the test case when uploaded to Hypergrade.
import java.util.HashMap;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.Scanner;
public class MorseEncoder {
private static final HashMap<Character, String> codeMappings = new HashMap<>();
public static void main(String[] args) {
initializeMappings();
Scanner textScanner = new Scanner(System.in);
System.out.println("Please enter a string to convert to Morse code:");
String textForEncoding = textScanner.nextLine().toUpperCase();
if ("ENTER".equals(textForEncoding)) {
System.out.println();
return;
}
String encodedOutput = encodeText(textForEncoding);
System.out.println(encodedOutput);
}
private static void initializeMappings() {
try (BufferedReader mappingFile = new BufferedReader(new FileReader("morse.txt"))) {
String lineContent;
while ((lineContent = mappingFile.readLine()) != null) {
if (lineContent.length() < 5) {
continue;
}
char alphaChar = lineContent.charAt(0);
String morseString = lineContent.substring(4).trim();
codeMappings.put(alphaChar, morseString);
}
} catch (IOException ioEx) {
ioEx.printStackTrace();
}
}
private static String encodeText(String textForEncoding) {
StringBuilder encodedStringBuilder = new StringBuilder();
boolean lastCharWasSpace = false;
for (char individualChar : textForEncoding.toCharArray()) {
if (individualChar == ' ') {
if (!lastCharWasSpace) {
encodedStringBuilder.append(" ");
lastCharWasSpace = true;
}
continue;
}
lastCharWasSpace = false;
String morseSymbol = codeMappings.get(individualChar);
if (morseSymbol != null) {
encodedStringBuilder.append(morseSymbol).append(' ');
}
}
return encodedStringBuilder.toString().trim();
}
}
0 -----
1 .----
2 ..---
3 ...--
4 ....-
5 .....
6 -....
7 --...
8 ---..
9 ----.
, --..--
. .-.-.-
? ..--..
A .-
B -...
C -.-.
D -..
E .
F ..-.
G --.
H ....
I ..
J .---
K -.-
L .-..
M --
N -.
O ---
P .--.
Q --.-
R .-.
S ...
T -
U ..-
V ...-
W .--
X -..-
Y -.--
Z --..
Test Case 1
ENTER
\n
Test Case 2
abcENTER
.- -... -.-. \n
Test Case 3
This is a sample string 1234.ENTER
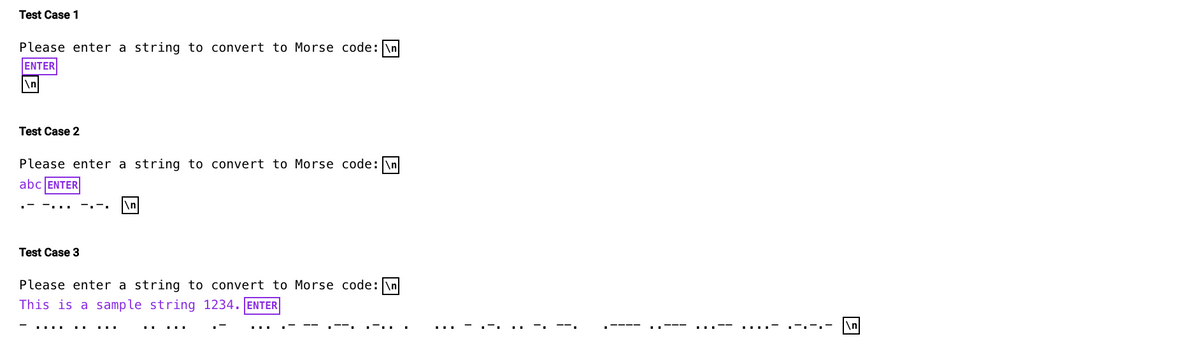
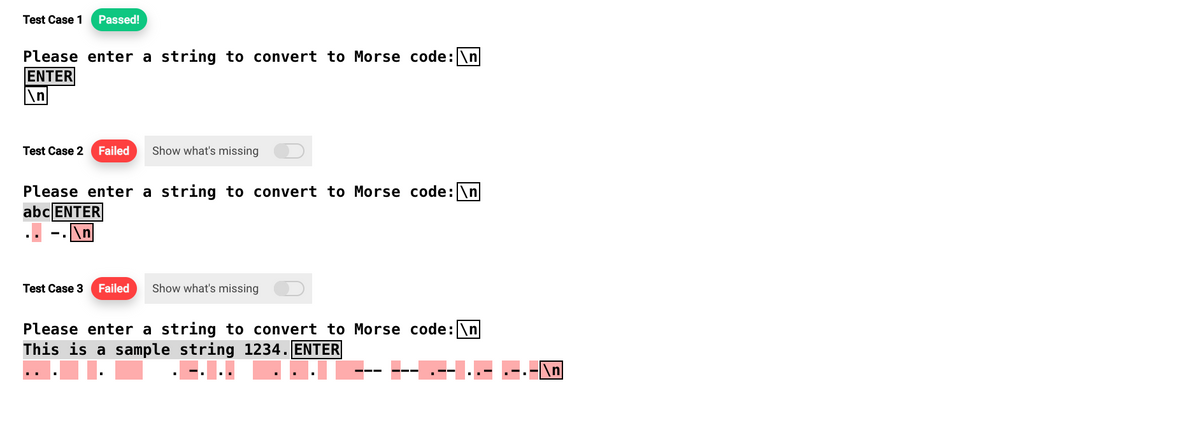

Step by step
Solved in 4 steps with 6 images

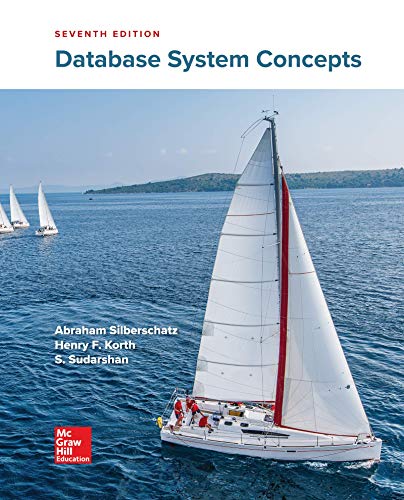
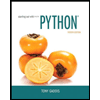
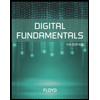
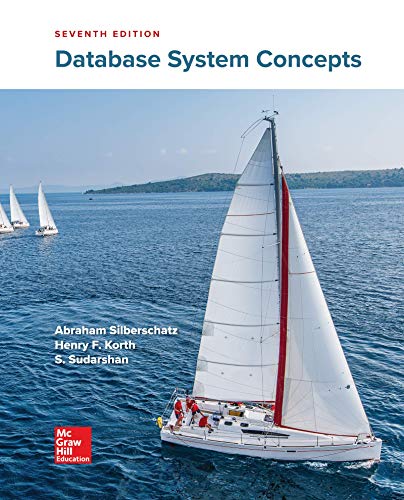
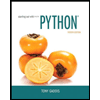
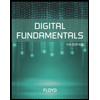
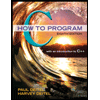
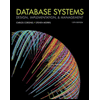
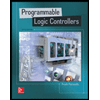