Java program help Simulate a slot machine. The customer will add a fixed amount of money to the machine and play the slots. There should be 4 images for the game: "Apple", "Orange", "Banana", and "Cherry". If three of the images match, the customer wins $2 and is added to their balance. If all four match, the customer wins $10 and that is added to their balance. If less then four match there is no prize money, and the customer is charged $1 for the try. The customer can quit after each try, and the program will print out the customer's cash balance. Coding Details: Use an array of strings to store the 4 strings listed above. Use a do-while loop for the program. The program starts once the user enters how much money they have. The decision to stop occurs at the bottom of the loop. The do-while loop keeps going until the user quits, or there is no money left. The pseudocode for the program is shown below: Determine the fruits to display (step 3 below) and print them Determine if there are 3 or 4 of the same image Display the results Update the customer balance as necessary Prompt to play, or quit Continue loop if customer wants to play and there's money for another try. Use the Random class to generate a random number between 0 and 3. This random number will be an index into the array of strings. Add the string at that index to an ArrayList. This should be done four times. When finished the ArrayList will contain 4 strings that represent randomly selected fruit images. Write a custom method that is called within the do-while loop. This method will accept the array list as a parameter, and it will return an integer that represents the number of times an 'fruit' appeared in the array list. Inside this method, use the frequency method from the Collections class to determine how many times a particular value is in a ArrayList. The Collections.frequency should be called for each image to get the counts by image. If your custom method returns a three, then add $2 to the balance. If it returns a four, then add $10 to the customer's balance. Display the contents of the arraylist on the same line with spaces between them. Display the results of the play and the current balance. At the bottom of the do-while loop, prompt the user if they want to continue to play (P) or to quit (Q). Case should not matter. If the user enters a character other than those two characters, treat anything other than a 'Q' as the starting point. Sample Output Enter dollar amount to play --> 5 Apple Apple Orange Orange << Not a winner this time >> Balance is: $4 Play Again (P) or Quit (Q) --> P Banana Banana Orange Cherry << Not a winner this time >> Balance is: $3 Play Again (P) or Quit (Q) p Banana Apple Banana Banana << You matched 3! That's $2 >> Balance is: $5 Play Again (P) or Quit (Q) q Thanks for playing! Cash Balance is $5 I appricate any help
Java program help
Simulate a slot machine.
The customer will add a fixed amount of money to the machine and play the slots.
There should be 4 images for the game: "Apple", "Orange", "Banana", and "Cherry".
If three of the images match, the customer wins $2 and is added to their balance. If all four match, the customer wins $10 and that is added to their balance. If less then four match there is no prize money, and the customer is charged $1 for the try. The customer can quit after each try, and the program will print out the customer's cash balance.
Coding Details:
- Use an array of strings to store the 4 strings listed above.
- Use a do-while loop for the program. The program starts once the user enters how much money they have. The decision to stop occurs at the bottom of the loop. The do-while loop keeps going until the user quits, or there is no money left. The pseudocode for the program is shown below:
- Determine the fruits to display (step 3 below) and print them
- Determine if there are 3 or 4 of the same image
- Display the results
- Update the customer balance as necessary
- Prompt to play, or quit
- Continue loop if customer wants to play and there's money for another try.
- Use the Random class to generate a random number between 0 and 3. This random number will be an index into the array of strings. Add the string at that index to an ArrayList. This should be done four times. When finished the ArrayList will contain 4 strings that represent randomly selected fruit images.
- Write a custom method that is called within the do-while loop. This method will accept the array list as a parameter, and it will return an integer that represents the number of times an 'fruit' appeared in the array list.
- Inside this method, use the frequency method from the Collections class to determine how many times a particular value is in a ArrayList. The Collections.frequency should be called for each image to get the counts by image.
- If your custom method returns a three, then add $2 to the balance. If it returns a four, then add $10 to the customer's balance.
- Display the contents of the arraylist on the same line with spaces between them.
- Display the results of the play and the current balance.
- At the bottom of the do-while loop, prompt the user if they want to continue to play (P) or to quit (Q). Case should not matter. If the user enters a character other than those two characters, treat anything other than a 'Q' as the starting point.
Sample Output
Enter dollar amount to play --> 5
Apple Apple Orange Orange
<< Not a winner this time >>
Balance is: $4
Play Again (P) or Quit (Q) --> P
Banana Banana Orange Cherry
<< Not a winner this time >>
Balance is: $3
Play Again (P) or Quit (Q) p
Banana Apple Banana Banana
<< You matched 3! That's $2 >>
Balance is: $5
Play Again (P) or Quit (Q) q
Thanks for playing! Cash Balance is $5
I appricate any help!

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

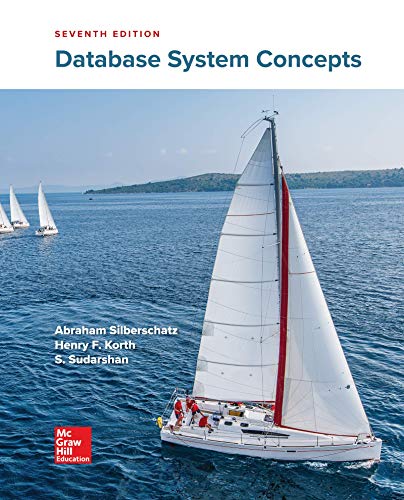
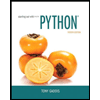
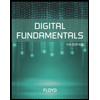
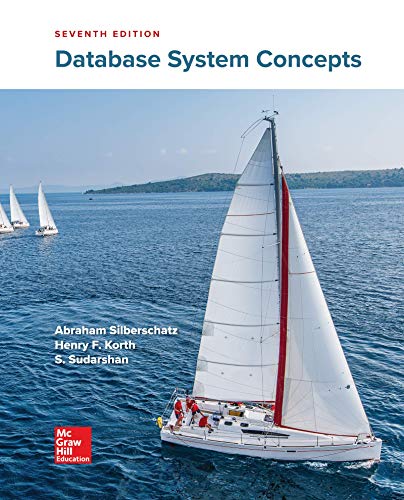
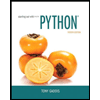
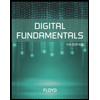
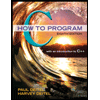
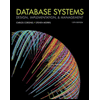
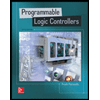