Java Program This assignment requires one project with two classes. Class Employee Class Employee- I will attach the code for this: //Import the required packages. import java.text.DecimalFormat; import java.text.NumberFormat; //Define the employee class. class Employee { //Define the data members. private String id, lastName, firstName; private int salary; //Create the constructor. public Employee(String id, String lastName, String firstName, int salary) { this.id = id; this.lastName = lastName; this.firstName = firstName; this.salary = salary; } //Define the getter methods. public String getId() { return id; } public String getLastName() { return lastName; } public String getFirstName() { return firstName; } public int getSalary() { return salary; } //Define the method to return the employee details. @Override public String toString() { //Use number format and decimal format //to format the salary to desired output. NumberFormat nfm = NumberFormat.getCurrencyInstance(); String pt = ((DecimalFormat) nfm).toPattern(); String npt = pt.replace("\u00A4", "").trim(); NumberFormat nfm2 = new DecimalFormat(npt); nfm2.setMinimumFractionDigits(0); //Return the attributes with message. return "ID " + id + ":" + lastName + ", " + firstName + ", salary $" + nfm2.format(salary); } } Executable Class create an array of Employee objects. create an ArrayList of Employee objects from that array. use an enhanced for loop to print all employees as shown in the sample output. create a TreeMap that uses Strings for keys and Employees as values. this TreeMap should map Employee ID numbers to their associated Employees. process the ArrayList to add elements to this map. print all employees in ID # order as shown, but do so using the TreeMap's forEach method and a lambda expression
Java Program
This assignment requires one project with two classes.
Class Employee
-
Class Employee- I will attach the code for this:
//Import the required packages.
import java.text.DecimalFormat;
import java.text.NumberFormat;
//Define the employee class.
class Employee {
//Define the data members.
private String id, lastName, firstName;
private int salary;
//Create the constructor.
public Employee(String id, String lastName,
String firstName, int salary) {
this.id = id;
this.lastName = lastName;
this.firstName = firstName;
this.salary = salary;
}
//Define the getter methods.
public String getId() {
return id;
}
public String getLastName() {
return lastName;
}
public String getFirstName() {
return firstName;
}
public int getSalary() {
return salary;
}
//Define the method to return the employee details.
@Override
public String toString() {
//Use number format and decimal format
//to format the salary to desired output.
NumberFormat nfm = NumberFormat.getCurrencyInstance();
String pt = ((DecimalFormat) nfm).toPattern();
String npt = pt.replace("\u00A4", "").trim();
NumberFormat nfm2 = new DecimalFormat(npt);
nfm2.setMinimumFractionDigits(0);
//Return the attributes with message.
return "ID " + id + ":" + lastName + ", " + firstName
+ ", salary $" + nfm2.format(salary);
}
}
Executable Class
- create an array of Employee objects.
- create an ArrayList of Employee objects from that array.
- use an enhanced for loop to print all employees as shown in the sample output.
- create a TreeMap that uses Strings for keys and Employees as values.
- this TreeMap should map Employee ID numbers to their associated Employees.
- process the ArrayList to add elements to this map.
- print all employees in ID # order as shown, but do so using the TreeMap's forEach method and a lambda expression
Output should look exactly like this:
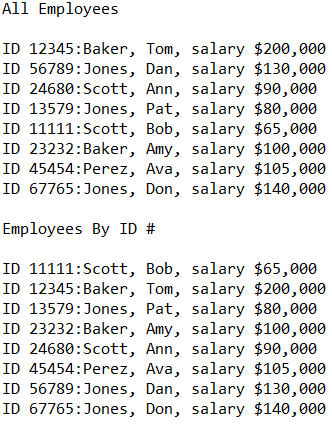

Step by step
Solved in 4 steps with 4 images

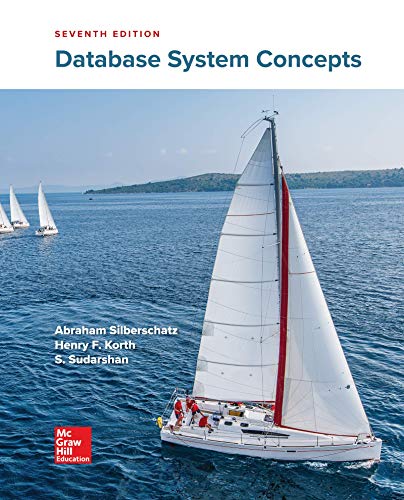
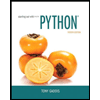
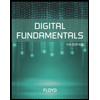
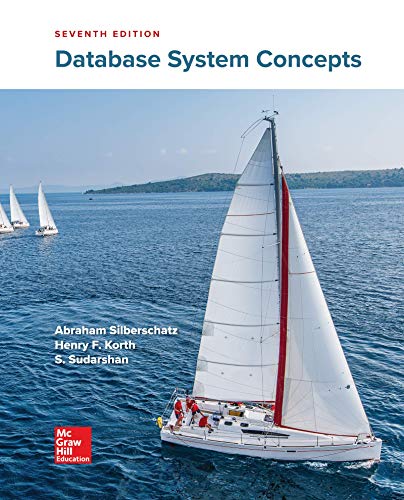
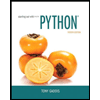
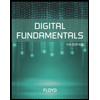
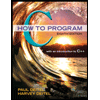
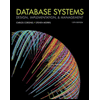
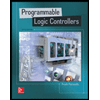