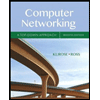
Java programming language
I need to complete the "public int remove" method in the bottom. Please help me.
image attached is the main.
public class ourArray {
//define an array of integers
private int[] Ar; //Ar is a reference
//declare
private int Capacity = 100;
private int size = 0;
private int Increment = 10;
//Default Constructor
public ourArray() {
Ar = new int[100];
Capacity = 100;
size = 0;
Increment = 10;
}
//Constructor that accepts the capacity (C). It creates an array of C integersm, sets capacity to C
// Increment to 10
public ourArray(int C) {
//create an array of C integers
Ar = new int[C];
size = 0;
Capacity = C;
Increment = 10;
}
//Constructor that accepts two integers (c, i) c for capacity and i for increment
public ourArray(int C, int I) {
//create an array of C integers
Ar = new int[C];
size = 0;
Capacity = C;
Increment = I;
}
//setter for Increment
public void setIncrement(int I) {
Increment = I;
}
//getter for size, capacity and increment.
public int getSize() {
return size;
}
public int getCapacity() {
return Capacity;
}
public int getIncrement() {
return Increment;
}
//- A method void add(int e); it adds e to the end of the array.
// A.add(-1); A.add(-5), A.add(-9) [-1, -5, -9]
public void add(int e) {
//add only if there is space
if(size >= Capacity) {
System.out.println("The array is full");
//get out
System.exit(1);
}
//if you are here it is not full
Ar[size] = e;
size++;
}
//isEmpty returns true if it is empty and false it it is not.
public boolean isEmpty() {
return (size == 0);
}
public String toString() {
if(this.isEmpty())
return "[]";
String st = "[";
//build your string [-1, -3, -9]
for(int i = 0; i < size-1; i++) {
//in the loop your are always dealing with element i
//v element i is Ar[i]
//append Ar[i], to the string
st += Ar[i] + ", ";
}
//close the bracket
st += Ar[size-1] + "]";
return st;
}
//HW1: Design a method that adds an element at the frsont
// [-2, -5, -9] to push the numbers up by one ===> [ , -2, -5, -9]
/*
* Code is
* 1. for(int i = 0; i< size; i++){
* 2. A[i+1] = A[i];
* }
* Trace this code for the example above
* 1. i = 0; 0 < 3 true
* 2. A[1] = A[0] = -2 [-2, -2, -9]
* 1. i = 1; 1 <3 true
* 2. A[2] = A[1] = -2 [-2, -2, -2]
* 1. i = 2; 2 < 3 true
* 2. A[3] = A[2] = -2 [-2, -2, -2, -2]
* 1. i = 3; 3 < 3 false get out
*
* THIS CODE DOEN'T WORK
*
* Trace this code [-2, -5, -9]
* 1. for(int i = 0; i< size; i++){
2.A[size-i] = A[size-i-1];
}
1. i = 0;
2. A[3-0] = A[3-0-1] = -9 [-2, -5, -9, -9]
1. i = 1
2. A[3 - 1] = A[1] = -5 [-2,-5, -5, -9]
1. i = 2;
2. A[1] = A[0] = -2 [-2,-2, -5, -9]
*/
public void addFront(int e) {
//check there is space
if (size >= this.Capacity) {
System.out.println("Array is full");
System.exit(1);
}
//bring all values up by one
for(int i = size; i > 0; i--){
Ar[i] = Ar[i-1];
}
//add e to index 0
Ar[0] = e;
size++;
}
//remove removes the last item and returns it
public int remove() {
if(this.isEmpty()) {
System.out.println("Error: the array is empty");
return -1;
}
int save = Ar[size-1]; //save the last element to be returned
//delete by just reduing the size
size--;
//return the removed item
return save;
}
//removeFront() removes the first item and returns it
//removeFront() removes the first item and returns it
public int removeFront() {
if(this.isEmpty()) {
System.out.println("Error: the array is empty");
return -1;
}
int save = Ar[0];
//bring the rest down by one (size-1)( element down)
for(int i = 0; i < size-1; i++) {
Ar[i] = Ar[i+1];//if youy use size instead of size-1 you may get
//outofbounds exception
}
size--;
return save;
}
//This method will add element e at index ind
public void add(int ind, int e) {
if (size >= this.Capacity) {
System.out.println("Array is full");
System.exit(1);
}
if(ind == 0)
this.addFront(e);
else if (ind == size)
this.add(e);
else if(ind < 0 || ind > size) {
System.out.println("Error: cannot add at those indices");
return;
}
//bring all values up by one
for(int i = size; i > ind; i--){
Ar[i] = Ar[i-1];
}
//add e to index 0
Ar[ind] = e;
size++;
}
//int remove(intind) removes element e at index ind and returns it.
public int remove(int ind) {
return 0;
}
}
![public class Tester
public static void main(String [] args) {
//create an object ourArray
//className objectName :
ourArray A = new ourArray();
= new className();
+ A.getSize() +
+ A.getIncrement ());
System.out.println("size: "
//display while empty
System.out.println("size: "
//add -3, -1, -9
A.add(-3);
A.add(-1);
A.add(-9);
Increment:
+ A.getSize() +
" + A.toString( ));
//show the content
+ A.getSize() +
+ A. toString 0);
System.out.println("size:
A.addFront(-13);
System.out.println("size: "
A.addFront (-25);
System.out.println("size: " + A.getSize() +
System.out.println("removing:
System.out.println("size:
System.out.println("removing:
System.out.println("size:
:
+ A.getSize() +
+ A.toString(0);
+ A.toString(0);
+ A. remove());
+ A.getSize( +
+ A. removeFront ());
+ A.getSize(O + "
%3D
+ A. toString(0);
%3D
" + A.toString( ));
}](https://content.bartleby.com/qna-images/question/33eba9e5-eb7d-44a1-93e3-ed0ee897e17f/2004bd9d-884e-450f-b9db-fc53a6332104/fbt62ga_thumbnail.png)

Step by stepSolved in 3 steps

- Write a program in Java that handles the order list for an online retailer. Your program should contain a minimum of three classes: Order Class Display Class Main Class Create a Order Class that uses a queue to hold the orders in the order they were taken. The queue should contain the customer last name, order number, and order total cost. Create a Display Class that will store a copy of the order queue in two arrays. Each array will contain the order list but one will be sorted by name and the other sorted by order number. When a order is taken and stored in the Order Class the program will update theDisplay Class arrays automatically sort them in descending order using quick sort and outputs the the contents to the console upon each entry of new data. Create a Main Class that will handle operator data input and when an order is added or removed, the program will update the Order Class and Display Class. The user will be presented a menu to add a order, remove a order and display…arrow_forwardGiven: public class Team { private Player[] players; private int squadSize; public Team(Player[] players) { this.players = players; this.size = players.length; } public Team() { this.players = new Player[4]; this.size = 0; } }How would I go about creating the given methods? (Rating variable in supplied java file.)arrow_forwardElevator simulation. I need help fixing this class The Simulation Class initializes and holds objects for Passengers, Elevators, and Floors. It reads and parses input files to create the necessary objects and sets the number of simulation iterations. The class also has methods to move the elevators up and down the building, as well as to pick up and drop off passengers. public class Simulation { private int numFloors; private ArrayList<Passenger> passengers; private ArrayList<Elevator> elevators; private ArrayList<Floor> floors; private int numIterations; public Simulation(String inputFile) throws FileNotFoundException { passengers = new ArrayList<>(); elevators = new ArrayList<>(); floors = new ArrayList<>(); // Read input file and initialize simulation parameters Scanner scanner = new Scanner(new File(inputFile)); while (scanner.hasNextLine()) { String line =…arrow_forward
- In java ****arrow_forwardpublic class Accumulator { private int total private String name; public Accummulator (string name , int total) { this .name = name; this .total=total; } } 3. In a main method, create an object of Accumulator with the name as "Mary" and total as 100.arrow_forwardIn java Define and create an array (called emps) of object references for objects of class Employee of length 100. (b) Create three objects for classes SalariedEmp, WeeklyEmp, and HourlyEmp (where these 3 classes are subclasses of class Employee); and with references e1, e2, e3 for the 3 objects:(c) Assign e1,e2, e3 to the first three elements of array emps:(d) Print the earnings of the 100 employee in the array emps in one loop (with polymorphism) where earnings are calculated with method earnings():arrow_forward
- Task 3: Implement method setup in PE1 class. The description of what is wanted is given in the starter code. public class PE1 { MazedogMaze; /** * This method sets up the maze using the given input argument * @param maze is a maze that is used to construct the dogMaze */ publicvoidsetup(String[][]maze){ /* insert your code here to create the dogMaze * using the input argument. */ } /** * This method returns true if the number of * gates in dogMaze >= 2. * @return it returns true, if enough gate exists (at least 2), otherwise false. */ publicbooleanenoughGate(){ // insert your code here. Change the return value to fit your purpose. returntrue; } /** * This method finds a path from the entrance gate to * the exit gate. * @param row is the index of the row, where the entrance is. * @param column is the index of the column, where the entrance is. * @return it returns a string that contains the path from the start to the end. * The return value should…arrow_forwardTask 3: Implement method setup in PE1 class. The description of what is wanted is given in the starter code. TASK 1 and 2 are done in class maze. Please use PE1 class. public class PE1 { MazedogMaze; /** * This method sets up the maze using the given input argument * @param maze is a maze that is used to construct the dogMaze */ publicvoidsetup(String[][]maze){ /* insert your code here to create the dogMaze * using the input argument. */ } /** * This method returns true if the number of * gates in dogMaze >= 2. * @return it returns true, if enough gate exists (at least 2), otherwise false. */ publicbooleanenoughGate(){ // insert your code here. Change the return value to fit your purpose. if() returntrue; } /** * This method finds a path from the entrance gate to * the exit gate. * @param row is the index of the row, where the entrance is. * @param column is the index of the column, where the entrance is. * @return it returns a string that contains the…arrow_forward2-7 Given the code below, which lines are valid and invalid.arrow_forward
- Please help debug this: Debugging Exercise 4-3, Farrell, Joyce, Java Programming, 9th edition, Cengage Learning public class DebugBox { private int width; private int length; private int height; private FixDebugBox() { length = 1; width = 1; height = 1; } public DebugBox(int width, int length, int height) { width = width; length = length; height = height; } public void showData() { System.out.println("Width: " + width + " Length: " + length + " Height: " + height); } public double getVolume() { double vol = length - width - height; return vol; } }arrow_forwardJavaarrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
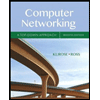
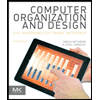
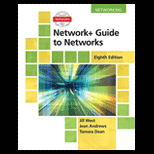
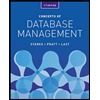
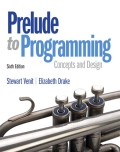
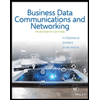