Java Your program will read in a credit card number and expiration date from the user and validate the data. The program will continue to ask for user input until a valid number and date are entered. The rules for what is valid are specified below. readYear() and readMonth(int) methods Complete the readYear() and readMonth(int) methods. These methods ask the user to input the expiration year and month of their credit card. Each method should contain a loop inside the method so the code repeats until a valid month or year is entered by the user. If the user enters an invalid year or month, print an error message and ask them to try again. The readMonth(int) method reads in the month as a number. A valid month is any number between 1 and 12 (inclusive). A valid year is 2022 or later. readCreditCardNumber() method Complete the readCreditCardNumber() method. This method asks the user to input their credit card number. This method should contain a loop so the code repeats until a valid credit card number is entered by the user. A number is valid if it passes three tests: It is 16 digits long. It starts with a 4, 5, or 6 as the first digit. It passes the checkSum test (described next). If the user enters an invalid number, print an error message describing the reason why the number is invalid and ask them to try again. Note that you do not have to account for a user entering non-numeric input. We expect your program to crash if this happens. passesCheckSum(String) method Complete the passesCheckSum(String) method. The method determines if those numbers pass the checkSum test, which is described on the Check Sum Algorithm page and is attached as two pictures. This method assumes you already have a String that is 16 digits long. You will invoke this method from the readCreditCardNumber() method. Output Print the valid card and date information back to the user. Display the credit card number in four-digit groupings with a space in between each grouping (e.g., 0000 0000 0000 0000). Display the month and year in "month/year" format (e.g., 12/2022, 4/2023). Code Design Follow Java coding conventions and best practices. Follow naming conventions for variables, methods, and constants. Use constants to improve readability and maintainability. Properly indent your code. Design your code (including loops and conditionals) to reduce repeated or duplicated code. Choose conditional and loop structures that are the best logical match to the task. Write helper methods when it will help clarify the code or reduce repeated code. Below is the provided template: public class CreditCardValidator { public static void main(String[] args) { int year = readUserYear(); int month = readUserMonth(year); long creditCardNumber = readUserCreditCardNumber(); // YOUR CODE HERE: output credit card number and date in the specified format } public static int readUserYear() { // YOUR CODE HERE return 0; // placeholder: remove when you write your own code } public static int readUserMonth() { // YOUR CODE HERE return 0; // placeholder: remove when you write your own code } public static long readUserCreditCardNumber() { // YOUR CODE HERE return 0; // placeholder: remove when you write your own code } // this method assumes cardNumber has 16 digits public static boolean passesCheckSum(String cardNumber) { // YOUR CODE HERE return false; // placeholder: remove when you write your own code } /* * Below is a collection of helper methods you might or might not use. */ // example: pass in the char '3' and return the int 3 public static int convertDigitCharToInt(char digit) { return Integer.valueOf(String.valueOf(digit)); } // example: pass in the String "4" and return the int 4 public static int convertDigitStringToInt(String digit) { return Integer.valueOf(digit); } // example: pass in the int 5 and return the String "5" public static String convertIntToString(int number) { return Integer.toString(number); } // this method assumes the int parameter is a single digit; it will not work properly otherwise // example: pass in the int 6 and return the char '6' public static char convertSingleDigitIntToChar(int digit) { return convertIntToString(digit).charAt(0); } }
Java
Your program will read in a credit card number and expiration date from the user and validate the data. The program will continue to ask for user input until a valid number and date are entered. The rules for what is valid are specified below.
readYear() and readMonth(int) methods
Complete the readYear() and readMonth(int) methods. These methods ask the user to input the expiration year and month of their credit card.
- Each method should contain a loop inside the method so the code repeats until a valid month or year is entered by the user.
- If the user enters an invalid year or month, print an error message and ask them to try again.
- The readMonth(int) method reads in the month as a number. A valid month is any number between 1 and 12 (inclusive).
- A valid year is 2022 or later.
readCreditCardNumber() method
Complete the readCreditCardNumber() method. This method asks the user to input their credit card number.
- This method should contain a loop so the code repeats until a valid credit card number is entered by the user.
- A number is valid if it passes three tests:
- It is 16 digits long.
- It starts with a 4, 5, or 6 as the first digit.
- It passes the checkSum test (described next).
- If the user enters an invalid number, print an error message describing the reason why the number is invalid and ask them to try again.
- Note that you do not have to account for a user entering non-numeric input. We expect your program to crash if this happens.
passesCheckSum(String) method
Complete the passesCheckSum(String) method. The method determines if those numbers pass the checkSum test, which is described on the Check Sum
- This method assumes you already have a String that is 16 digits long.
- You will invoke this method from the readCreditCardNumber() method.
Output
Print the valid card and date information back to the user.
- Display the credit card number in four-digit groupings with a space in between each grouping (e.g., 0000 0000 0000 0000).
- Display the month and year in "month/year" format (e.g., 12/2022, 4/2023).
Code Design
- Follow Java coding conventions and best practices.
- Follow naming conventions for variables, methods, and constants.
- Use constants to improve readability and maintainability.
- Properly indent your code.
- Design your code (including loops and conditionals) to reduce repeated or duplicated code.
- Choose conditional and loop structures that are the best logical match to the task.
- Write helper methods when it will help clarify the code or reduce repeated code.
Below is the provided template:
public class CreditCardValidator {
public static void main(String[] args) {
int year = readUserYear();
int month = readUserMonth(year);
long creditCardNumber = readUserCreditCardNumber();
// YOUR CODE HERE: output credit card number and date in the specified format
}
public static int readUserYear() {
// YOUR CODE HERE
return 0; // placeholder: remove when you write your own code
}
public static int readUserMonth() {
// YOUR CODE HERE
return 0; // placeholder: remove when you write your own code
}
public static long readUserCreditCardNumber() {
// YOUR CODE HERE
return 0; // placeholder: remove when you write your own code
}
// this method assumes cardNumber has 16 digits
public static boolean passesCheckSum(String cardNumber) {
// YOUR CODE HERE
return false; // placeholder: remove when you write your own code
}
/*
* Below is a collection of helper methods you might or might not use.
*/
// example: pass in the char '3' and return the int 3
public static int convertDigitCharToInt(char digit) {
return Integer.valueOf(String.valueOf(digit));
}
// example: pass in the String "4" and return the int 4
public static int convertDigitStringToInt(String digit) {
return Integer.valueOf(digit);
}
// example: pass in the int 5 and return the String "5"
public static String convertIntToString(int number) {
return Integer.toString(number);
}
// this method assumes the int parameter is a single digit; it will not work properly otherwise
// example: pass in the int 6 and return the char '6'
public static char convertSingleDigitIntToChar(int digit) {
return convertIntToString(digit).charAt(0);
}
}
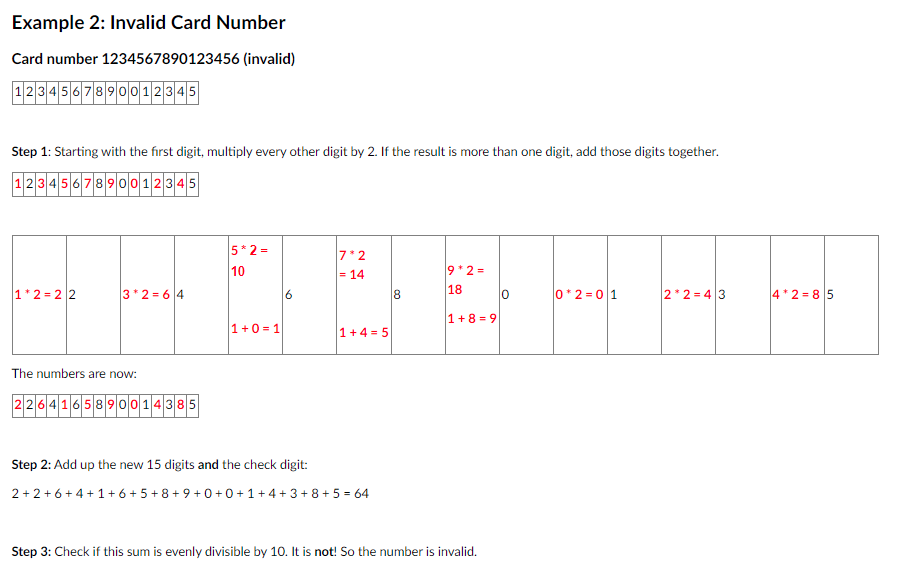
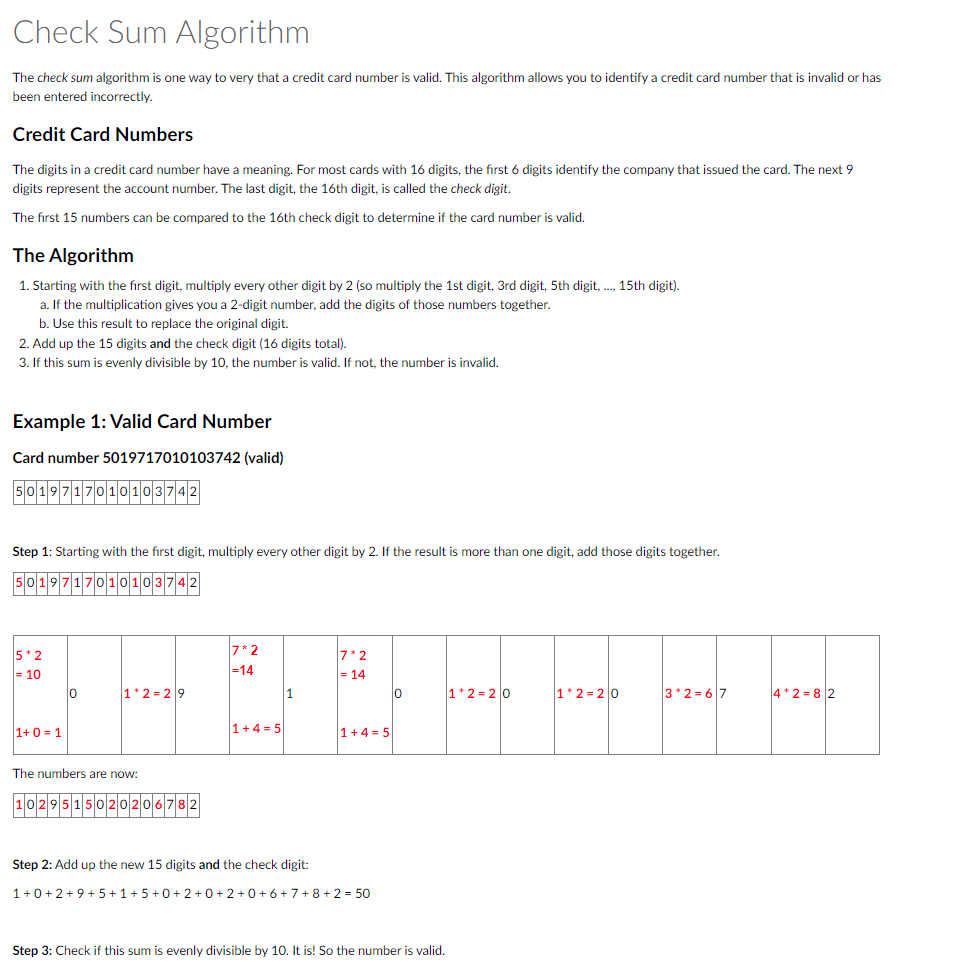

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

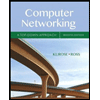
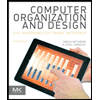
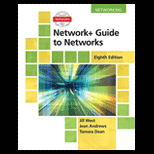
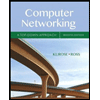
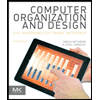
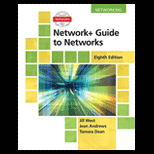
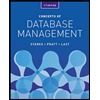
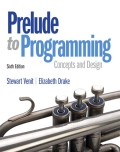
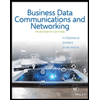