Keypad.h
Keypad.h & LiquidCrystal.h files needed to run this code
#include <Keypad.h>
#include <LiquidCrystal.h>
const byte ROWS = 4; //four rows
const byte COLS = 4; //three columns
char keys[ROWS][COLS] = {
{'1', '2', '3', 'A'},
{'4', '5', '6', 'B'},
{'7', '8', '9', 'C'},
{'*', '0', '#', 'D'}
};
byte rowPins[ROWS] = {53, 51, 49, 47}; //connect to the row pinouts of the keypad
byte colPins[COLS] = {45, 43, 41, 39 }; //connect to the column pinouts of the keypad
float intprice = 0;
int total_stamps = 99; //The default total number, you can change it or make it a 'n' digits one
float total_money = 0;// default total money =0 dollar
String domestic_quantity = " ";
String international_quantity = " ";
float amount_ordered = 0;
float amount_ordered_int = 0;
Keypad keypad = Keypad( makeKeymap(keys), rowPins, colPins, ROWS, COLS );
LiquidCrystal lcd(8, 9, 4, 5, 6, 7);
void setup() {
lcd.begin (16, 2);
lcd.setCursor(0, 0);
lcd.print("*STAMP VENDING*");
lcd.setCursor(1, 1);
lcd.print("MACHINE!!"); //What's written on the LCD you can change
Serial.begin(9600);
delay(100);
Serial.println("code starting");
}
char key = ' ';
void loop() {
char key = keypad.getKey();
if (key)Serial.println(key);
if (key == '1') { //
Serial.println(key);
lcd.clear();
lcd.setCursor(0, 0);
//lcd.println("*DOMESTIC STAMPS"); //Message to show
// lcd.setCursor(1, 1);
// lcd.print("DOMESTIC:$0.5");
// delay(1000);
ReadDomestic(); //Getting code function
} else if (key == '2') {
Serial.println(key);
// lcd.clear();
// lcd.setCursor(0, 1);
// lcd.print("inte :$2");
delay(1000);
ReadInternational(); //Getting code function
}
else if (key == 'D') {
Serial.println(key);
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("stamp rem :");
lcd.print(total_stamps);
lcd.setCursor(0, 1);
lcd.print("total money : ");
lcd.print(total_money);
delay(3000);
}
delay(200);
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("1.DOMESTIC");
lcd.setCursor(0, 1);
lcd.print("2.INTERNATIONAL");
//Return to standby mode it's the message do display when waiting
}
void ReadDomestic() { //Getting code sequence
lcd.clear();
lcd.print("1.DOMESTIC $0.5");
lcd.setCursor(0, 1);
lcd.print("# To cancel");
while (1) {
key = keypad.getKey();
if (key == '1') {
dom();
break;
}
else if (key == '#') {
break;
}
}
}
void dom() {
int i = 0; //All variables set to 0
int a = 0;
int j = 0;
lcd.clear();
lcd.print("Enter amount ");
while (1) { //The user press 0 to confirm the code otherwise he can keep typing
key = keypad.getKey();
if (key ) { //If the char typed isn't A and neither "nothing"
Serial.println(key);
lcd.setCursor(j, 1); //This to write KEYPRESEED on the LCD whenever a key is pressed it's position is controlled by j
lcd.print(key);
j++;
domestic_quantity += key;
}
if (j == 2) {
j = 0;
Serial.println(domestic_quantity);
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("amount ordered: ");
lcd.setCursor(0, 1);
amount_ordered =0.5*(domestic_quantity.toInt());
lcd.print(amount_ordered);
delay(2000);
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("press* confirm");
lcd.setCursor(0, 1);
lcd.print("press# cancel");
for (;;) {
key = keypad.getKey();
if (key == '*') {
amount_ordered = 0.5 * (domestic_quantity.toInt());
total_stamps = total_stamps - (domestic_quantity.toInt());
total_money = total_money + amount_ordered;
domestic_quantity = " ";
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Sucessful");
delay(2000);
break;
}
else if (key == '#') {
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("cancelled");
domestic_quantity = " ";
delay(2000);
break;
}
}
break;
}
}
}
void ReadInternational() {
lcd.clear();
lcd.print("1. zone 1 :$ 1.5");
lcd.setCursor(0, 1);
lcd.print("2. zone 2 :$2");
while (1) {
key = keypad.getKey();
if (key == '1') {
intprice = 1.5;
inte();
break;
}
else if (key == '2') {
intprice = 2;
inte();
break;
}
else if (key == '#') {
break;
}
}
}
void inte() {
//Getting code sequence
int i = 0; //All variables set to 0
int a = 0;
int j = 0;
lcd.clear();
lcd.print("Enter amount");
while (1) { //The user press 0 to confirm the code otherwise he can keep typing
key = keypad.getKey();
if (key ) { //If the char typed isn't A and neither "nothing"
Serial.println(key);
lcd.setCursor(j, 1 ); //This to write KEYPRESEED on the LCD whenever a key is pressed it's position is controlled by j
lcd.print(key);
j++;
international_quantity += key;
}
if (j == 2 ) {
delay(1000);
j = 0;
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Amount ordered: ");
lcd.setCursor(0, 1);
amount_ordered_int = intprice*(international_quantity.toInt());
lcd.print(amount_ordered_int);
delay(2000);
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("press* confirm ");
lcd.setCursor(0, 1);
lcd.print("press# cancel");
while (1) {
key = keypad.getKey();
if (key == '*') {
amount_ordered_int = intprice * (international_quantity.toInt());
total_stamps = total_stamps - (international_quantity.toInt());
total_money = total_money + amount_ordered_int;
international_quantity = " ";
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Sucessful");
delay(2000);
break;
}
else if (key == '#') {
lcd.clear();
lcd.setCursor(0, 0);
lcd.print("Cancelled");
delay(2000);
international_quantity = " ";
break;
}
}
break;
}
}
}
![[Error] Keypad.h: No such file or directory](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4c0302c3-9463-45ae-ba24-48b4ac042a0f%2F808d2f89-05e3-49a6-afb0-50f8c8f5b607%2Fio6cm3_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps

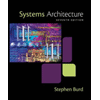
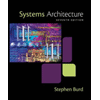