Laboratory Exercise Understanding Algorithm with Python Objective: At the end of the exercise, the students should be able to: ▪ Construct a Python script based on the given algorithms. Software Requirement: ▪ Python 3.7 or higher Procedure: 1. Study the following sample Python syntaxes: Task Sample Syntax Remarks Variable declaration x = 5 No need to indicate the data type. Do not add semi-colon. Comment #This is a comment. input(“Enter your name”) User input name = Convert string to int(x) Convert to compare or int or float. float(x) compute values. Convert int or float str(x) Convert if the output must to string. show a number and a string. Combine conditional x > 5 and x <10 statements. x > 5 or x < 10 not(x < 5) if statement if x == 0: Use four (4) spaces per print(“Enter a higher number”) indentation level. else-if statement elif x == 0: print(“Enter a higher number”) else statement else: print(“Try again”) t = [“red”, “blue”, “green”] Python recommends the Create a list of items. my_lis use of lists than arrays. Display all items in a print(my_list) list. Create an empty list. my_list = [] t.append(“yellow”) Add an item to the end my_lis of a list. 2. Open Notepad++. 3. Create a Python script that will compare two (2) numbers entered by the user. Refer to the sample syntaxes and the algorithm below. 3.1. User enters the first number. 3.2. User enters the second number. 3.3. If the first number is less than, greater than, or equal the second number, a message is displayed. 4. Save the script as algo1.py. 5. To test and run the script, open Command Prompt. Navigate to your file’s location then type python algo1.py.
IT1815
Laboratory Exercise
Understanding
Objective:
At the end of the exercise, the students should be able to:
▪ Construct a Python script based on the given algorithms.
Software Requirement:
▪ Python 3.7 or higher
Procedure:
1. Study the following sample Python syntaxes:
Task Sample Syntax Remarks
Variable declaration x = 5 No need to indicate the
data type. Do not add
semi-colon.
Comment #This is a comment.
input(“Enter your name”)
User input name =
Convert string to int(x) Convert to compare or
int or float. float(x) compute values.
Convert int or float str(x) Convert if the output must
to string. show a number and a
string.
Combine conditional x > 5 and x <10
statements. x > 5 or x < 10
not(x < 5)
if statement if x == 0: Use four (4) spaces per
print(“Enter a higher number”) indentation level.
else-if statement elif x == 0:
print(“Enter a higher number”)
else statement else:
print(“Try again”)
t = [“red”, “blue”, “green”] Python recommends the
Create a list of items. my_lis
use of lists than arrays.
Display all items in a print(my_list)
list.
Create an empty list. my_list = []
t.append(“yellow”)
Add an item to the end my_lis
of a list.
2. Open Notepad++.
3. Create a Python script that will compare two (2) numbers entered by the user. Refer to the sample
syntaxes and the algorithm below.
3.1. User enters the first number.
3.2. User enters the second number.
3.3. If the first number is less than, greater than, or equal the second number, a message is
displayed.
4. Save the script as algo1.py.
5. To test and run the script, open Command Prompt. Navigate to your file’s location then type python
algo1.py.

Step by step
Solved in 3 steps with 2 images

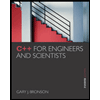
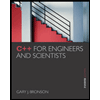