Language: C++ Given: Main.cpp #include #include "Shape.h" using namespace std; void main() { /////// Untouchable Block #1 ////////// Shape* shape; /////// End of Untouchable Block #1 ////////// /////// Untouchable Block #2 ////////// if (shape == nullptr) { cout << "What shape is this?! Good bye!"; return; } cout << "The perimeter of your " << shape->getShapeName() << ": " << shape->getPerimeter() << endl; cout << "The area of your " << shape->getShapeName() << ": " << shape->getArea() << endl; /////// End of Untouchable Block #2 ////////// } Shape.cpp string Shape::getShapeName() { switch (mShapeType) { case ShapeType::CIRCLE: return "circle"; case ShapeType::SQUARE: return "square"; case ShapeType::RECTANGLE: return "rectangle"; case ShapeType::TRIANGLE: return "triangle"; default: return "unknown shape"; } return "unknown shape"; } ShapeType.h #pragma once enum class ShapeType { CIRCLE, SQUARE, RECTANGLE, TRIANGLE }; Shape.h #pragma once #include #include "ShapeType.h" using namespace std; class Shape { private: ShapeType mShapeType; double mPerimenter; double mArea; public: Shape(ShapeType shapeType); ~Shape(); protected: void setPerimenter(double perimeter); void setArea(double area); public: double getPerimeter(); double getArea(); ShapeType getShapeType(); string getShapeName(); };
Please help, I just posted this question and got the wrong answer to it. This assignment is not graded, I just need to understand how to do it. all the information to the problem is in the screenshots and the code that is given is written below. please just follow the technical requirements, Thank you!
12 code files (Your 4 shape headers, your 4 shape
implementations, Shape header, Shape Implementation, ShapeType header, and the main.cpp files) four files are given to start the assignment and 8 more are needed, 4 shape headers and 4 shape implementations.
Problem Description
Let us create an interface where a user can select one of four shapes: circle, square, rectangle, or triangle. The user then enters information about the selected shape (for example, radius for circle, width and length for rectangle, etc.). Then the program displays information about the shape (perimeter and area). Use the following equations for calculation of perimeter and area.
Circle:
P = 2*pi*r, A = pi * r2
Square:
P = 4*s, A = s2
Rectangle:
P = 2*L + 2*w, A = L*w
Triangle:
P = a + b + c , A = b*h/2
a, b, and c are the sides of the triangle, where b is the base and h is the height.
You are given a starting point with some implemented code. Your job is to use this code to complete the solution to the problem described above.
Technical requirements-
- You are given a class called Shape, an enumeration called ShapeType, and a starting point to the main function.
- Create one class per shape required.
- Each class should be split into header/implementation files as we described in class
o Each class should publicly inherit from the Shape class
o Each class should have a fully parameterized constructor - The number of parameters in the constructor depend on the values needed to calculate the shape’s perimeter and area (one double for circle, two doubles for rectangle, etc.)
It should only contain parameters needed for the parameter and area calculation
Create the base instance by providing the correct ShapeType at construction time - Assign calculated perimeter and area using the available functions in Shape
• You should not modify any code in Shape, ShapeType, and the two given blocks in the main function - Give the user an interface to select the wanted shape and shape information
o Ask the user for the shape, e.g., A for Circle, B for Square, etc.
o Depending on the selected shape, ask for the shape’s information
For example, ask for radius if circle was selected, side if square was selected, etc.
o Create the selected shape with the given information and save it in the shape variable, which was declared in Untouchable Block #1
o If incorrect information is given (such as a letter that does not represent a shape or a negative value for shape information), create a null shape instead
o The rest of the program should automatically display the correct shape’s name, perimeter, and area to the user
• If you create your shapes with the new keyword, make sure you delete them to avoid memory leaks
Language: C++
Given:
Main.cpp
#include
#include "Shape.h"
using namespace std;
void main() {
/////// Untouchable Block #1 //////////
Shape* shape;
/////// End of Untouchable Block #1 //////////
/////// Untouchable Block #2 //////////
if (shape == nullptr) {
cout << "What shape is this?! Good bye!";
return;
}
cout << "The perimeter of your " << shape->getShapeName() << ": " <<
shape->getPerimeter() << endl;
cout << "The area of your " << shape->getShapeName() << ": " <<
shape->getArea() << endl;
/////// End of Untouchable Block #2 //////////
}
Shape.cpp
string Shape::getShapeName() {
switch (mShapeType) {
case ShapeType::CIRCLE:
return "circle";
case ShapeType::SQUARE:
return "square";
case ShapeType::RECTANGLE:
return "rectangle";
case ShapeType::TRIANGLE:
return "triangle";
default:
return "unknown shape";
}
return "unknown shape";
}
ShapeType.h
#pragma once
enum class ShapeType {
CIRCLE,
SQUARE,
RECTANGLE,
TRIANGLE
};
Shape.h
#pragma once
#include
#include "ShapeType.h"
using namespace std;
class Shape {
private:
ShapeType mShapeType;
double mPerimenter;
double mArea;
public:
Shape(ShapeType shapeType);
~Shape();
protected:
void setPerimenter(double perimeter);
void setArea(double area);
public:
double getPerimeter();
double getArea();
ShapeType getShapeType();
string getShapeName();
};

Step by step
Solved in 5 steps

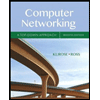
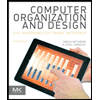
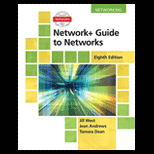
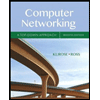
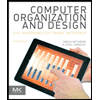
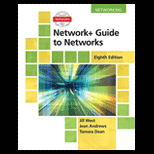
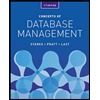
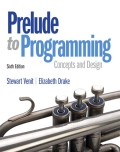
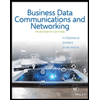