lease explain the flow of this C++ Program. It doesn't have to be long as long as you explain the each function of the program, if you can explain it line by line then it is better and I will give you a good rating.
Please explain the flow of this C++ Program. It doesn't have to be long as long as you explain the each function of the program, if you can explain it line by line then it is better and I will give you a good rating.
#include <cstdlib>
#include <math.h>
#include <cstring>
#include <iostream>
using namespace std;
class HashTable {
string* table;
int N;
int count;
// TODO: Polynomial Hash Code using a=7
int hash_code(string key) {
int code;
int hash = 0;
for (int i = 0; i < key.size(); i++) {
char ch = key[i];
code += ((ch - 96) * pow(7, key.size() - (i + 1)));
}
return code;
}
// TODO: This hash table uses a MAD compression function
// where a = 11, b = 461, p = 919
int compress(int code) {
return (((11*code)+461) % 919) % N;
}
// Using the knowledge that a hash function is composed of two portions
int hashfn(string key) {
return compress(hash_code(key));
}
public:
HashTable(int N) {
this->N=N;
table=(string*)malloc(sizeof (string) * N);
for(int j=0; j<N; j++){
table[j] = "a";
}
count = 0;
}
// TODO Implement insert
int insert(string key) {
int hval = hashfn(key);
int coll = 0;
if(count != N){
for(int j=0; j < N; j++){
int i = (hval + j*j) % N;
if(table[i].compare(key) == 0){
return -1;
}
if (table[i] == "a"){
table[i] = key;
count++;
break;
}
coll++;
}
}
return coll;
}
// TODO Implement search
// Tip: str1.compare(str2) will return 0 if both strings are the same
int search(string key) {
int cells=0,i;
for(int j=0;j<N;j++){
i = (hashfn(key) +(j*j)) % N;
if(table[i].compare(key)==0){
return cells;
}
cells++;
}
return -1;
}
// TODO Implement remove
int remove(string key) {
int cells=0,i;
for(int j=0;j<N;j++){
i=(hashfn(key) +(j*j)) % N;
if(table[i].compare(key)==0){
table[i]="a";
return cells;
}
cells++;
}
return -1;
}
// TODO Implement print
void print() {
for(int i=0;i<N;i++){
cout<<i <<"\t";
}
cout<<endl;
for(int i=0;i<N;i++){
if (table[i]=="a"){
cout<<"\t";
} else{
cout<<table[i] <<"\t";
}
}
cout<<endl;
}
};

Step by step
Solved in 2 steps

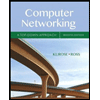
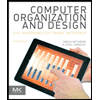
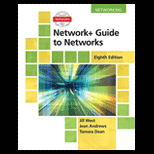
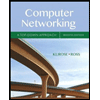
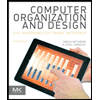
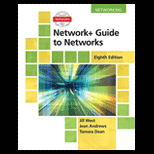
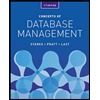
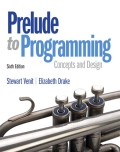
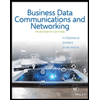