LEASE FIX THE CODE PROPERLY BELOW AND MAKE SURE IT WILL RUN PROPERLY ONLY USE C LANGUAGE BECAUSE ITS NOT RUNNING IN C COMPILER #include #include #include #include typedef struct Date Date; struct Date{ int day, month, year; void print() { cout << day << "-" << month << "-" << year << endl; } //Input data from the user }; Date _Date(int d = 1, int m = 1, int y = 2000) { day = d; month = m; year = y; } void input() { int day,month,year; printf("Enter day:"); scanf("%d", &day); ; printf ("Enter month:"); scanf("%d", &month); printf( "Enter year:"); scanf("%d", &year); int getYear() { return year; } int getMonth() { return month; } int getDay() { return day; } } // A medicine class is made struct Medicine { protected: int medID; int price; Date expiryDate; int quantityInStock; public: //Constructor Medicine() {} //Parametrized Constructor }; Medicine _Medicine(int id, int p, Date e, int q) { medID = id; price = p; expiryDate = e; quantityInStock = q; } //Input medicine detail void input() { printf(" Enter Medicine Id:\n"); scanf("%d", &medID); printf("Enter price of medicine:"); scanf("%d", &price); printf("Enter quantity in stock:\n"); scanf("%d", &quantityInStock); //expiryDate is an object of class Date so the input method of Date class is called using the object expiryDate expiryDate.input(); } //Display medicine record void display() { printf("Medicine ID: %d:" , medID ,"\n"); printf("Price: %d:", price, "\n"); printf("Quantity in Stock: %d:" ,quantityInStock,"\n"); printf("Expiry Date: %d:"); expiryDate.print(); printf( "---------- \n"); } void setquantity(int q) { quantityInStock = q; } void setID(int id) { medID = id; } int getID() { return medID; } int getquantity() { return quantityInStock; } static int countRecords() { ifstream f("medData.dat", ios::binary); f.seekg(0, ios::end); int loc = f.tellg(); return loc / sizeof(Medicine); } friend void saveMedicineData(Medicine m); friend void displayAllData(); void updateStock(int mID, int quantity) { fstream f; http://f.open("medData.dat", ios::out | ios::in | ios::binary); int n = Medicine::countRecords(); //Read all records from file into an array Medicine* e = new Medicine[n]; for (int i = 0; i < n; i++) { http://f.read(reinterpret_cast(&e[i]), sizeof(Medicine)); if (mID == medID) { int newquantity = e[i].getquantity() - quantity; e[i].setquantity(newquantity); } } f.seekp(0); f.write(reinterpret_cast(e), sizeof(Medicine) * n); f.close(); } void saveMedicineData(Medicine m) { ofstream outFile; http://outFile.open("medData.dat", ios::binary | ios::app); outFile.write(reinterpret_cast (&m), sizeof(Medicine)); outFile.close(); printf("\n\nMedicine record has been created successfully "); sin.ignore(); sin.get(); system("pause"); } void displayAllData() { Medicine me; ifstream inFile; http://inFile.open("medData.dat", ios::binary); if (!inFile) { printf( "File could not be open !! Press any Key..."); sin.ignore(); sin.get()in.ignore(); sin.get(); system("pause"); } } int main() {//First object of medicine Medicine med; med.input(); med.display(); saveMedicineData(med); displayAllData(); //Updation int medToSell; printf("Enter ID of medicine to sale \n"); scanf("%d", &medToSell); int quantitySold; printf("Enter the quantity to be sold\n"); scanf("%d", &quantitySold); printf("----------\n"); printf("Selling medicine: %d:",medToSell , " to a customer.\n"); printf("Quantity Sold: %d:" ,quantitySold ,"\n"); med.updateStock(medToSell, quantitySold); printf("----------\n" ); //See the updated list of medicines after sale printf("Updated Stock after sales:\n"); displayAllData(); printf( "---End---\n" )
PLEASE FIX THE CODE PROPERLY BELOW AND MAKE SURE IT WILL RUN PROPERLY ONLY USE C LANGUAGE BECAUSE ITS NOT RUNNING IN C COMPILER
#include <stdio.h>
#include<conio.h>
#include<string.h>
#include<math.h>
typedef struct Date Date;
struct Date{
int day, month, year;
void print() {
cout << day << "-" << month << "-" << year << endl;
}
//Input data from the user
};
Date _Date(int d = 1, int m = 1, int y = 2000) {
day = d;
month = m;
year = y;
}
void input() {
int day,month,year;
printf("Enter day:");
scanf("%d", &day); ;
printf ("Enter month:");
scanf("%d", &month);
printf( "Enter year:");
scanf("%d", &year);
int getYear() { return year; }
int getMonth() { return month; }
int getDay() { return day; }
}
// A medicine class is made
struct Medicine {
protected:
int medID;
int price;
Date expiryDate;
int quantityInStock;
public:
//Constructor
Medicine() {}
//Parametrized Constructor
};
Medicine _Medicine(int id, int p, Date e, int q) {
medID = id;
price = p;
expiryDate = e;
quantityInStock = q;
}
//Input medicine detail
void input()
{
printf(" Enter Medicine Id:\n");
scanf("%d", &medID);
printf("Enter price of medicine:");
scanf("%d", &price);
printf("Enter quantity in stock:\n");
scanf("%d", &quantityInStock);
//expiryDate is an object of class Date so the input method of Date class is called using the object expiryDate
expiryDate.input();
}
//Display medicine record
void display() {
printf("Medicine ID: %d:" , medID ,"\n");
printf("Price: %d:", price, "\n");
printf("Quantity in Stock: %d:" ,quantityInStock,"\n");
printf("Expiry Date: %d:");
expiryDate.print();
printf( "---------- \n");
}
void setquantity(int q) { quantityInStock = q; }
void setID(int id) { medID = id; }
int getID() { return medID; }
int getquantity() { return quantityInStock; }
static int countRecords() {
ifstream f("medData.dat", ios::binary);
f.seekg(0, ios::end);
int loc = f.tellg();
return loc / sizeof(Medicine);
}
friend void saveMedicineData(Medicine m);
friend void displayAllData();
void updateStock(int mID, int quantity) {
fstream f;
http://f.open("medData.dat", ios::out | ios::in | ios::binary);
int n = Medicine::countRecords();
//Read all records from file into an array
Medicine* e = new Medicine[n];
for (int i = 0; i < n; i++) {
http://f.read(reinterpret_cast<char*>(&e[i]), sizeof(Medicine));
if (mID == medID)
{
int newquantity = e[i].getquantity() - quantity;
e[i].setquantity(newquantity);
}
}
f.seekp(0);
f.write(reinterpret_cast<char*>(e), sizeof(Medicine) * n);
f.close();
}
void saveMedicineData(Medicine m)
{
ofstream outFile;
http://outFile.open("medData.dat", ios::binary | ios::app);
outFile.write(reinterpret_cast<char*> (&m), sizeof(Medicine));
outFile.close();
printf("\n\nMedicine record has been created successfully ");
sin.ignore();
sin.get();
system("pause");
}
void displayAllData()
{
Medicine me;
ifstream inFile;
http://inFile.open("medData.dat", ios::binary);
if (!inFile)
{
printf( "File could not be open !! Press any Key...");
sin.ignore();
sin.get()in.ignore();
sin.get();
system("pause");
}
}
int main()
{//First object of medicine
Medicine med;
med.input();
med.display();
saveMedicineData(med);
displayAllData();
//Updation
int medToSell;
printf("Enter ID of medicine to sale \n");
scanf("%d", &medToSell);
int quantitySold;
printf("Enter the quantity to be sold\n");
scanf("%d", &quantitySold);
printf("----------\n");
printf("Selling medicine: %d:",medToSell , " to a customer.\n");
printf("Quantity Sold: %d:" ,quantitySold ,"\n");
med.updateStock(medToSell, quantitySold);
printf("----------\n" );
//See the updated list of medicines after sale
printf("Updated Stock after sales:\n");
displayAllData();
printf( "---End---\n" );
}

Step by step
Solved in 2 steps

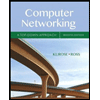
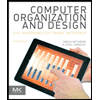
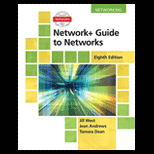
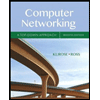
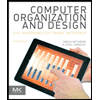
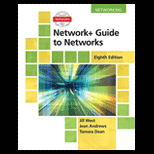
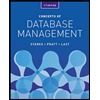
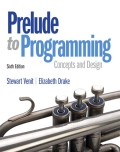
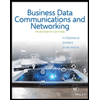