listsum() takes a list of integers and the list’s length. listsum() gets the sum by adding the sum of the halves. Each half is recursively divided into two until it reaches the base case (a list containing a single element or an empty list). Trace and illustrate what happens (draw the recursion of this code step-by-step using the format of the example attached.)
listsum() takes a list of integers and the list’s length. listsum() gets the sum by adding the sum of the halves. Each half is recursively divided into two until it reaches the base case (a list containing a single element or an empty list). Trace and illustrate what happens (draw the recursion of this code step-by-step using the format of the example attached.)
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter16: Searching, Sorting And Vector Type
Section: Chapter Questions
Problem 1TF
Related questions
Question
listsum() takes a list of integers and the list’s length. listsum() gets the sum by adding the sum of the halves. Each half is recursively divided into two until it reaches the base case (a list containing a single element or an empty list).
Trace and illustrate what happens (draw the recursion of this code step-by-step using the format of the example attached.)
![def listsum(numlist, size):
# - base case
# if the list is empty
if size 0:
return 0
# if the list contains a single element
elif size == 1:
return numlist[0]
10
# get the halfway point
11
# so we'll know where to divide the list in half
12
mid =
size//2
13
14
# --recursive step--
15
# add the sum of the left side with the sum of the right side
16
#tip: you need to pass the left side to one function call
17
# and the right side to the other
18
return listsum(numlist[0:mid], Len(numlist[0:mid])) + listsum(numlist[mid:], Len(numlist[mid:]
19
20
21
numbers
=
[3,5,4,1,7,2,9,8,0,6]
22 print(listsum(numbers, Len(numbers)))
1~3
2
3
4
5
6
7
8
9
FROM](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb6ac223c-9216-484a-9b59-f2ea9ea75fa6%2F105cad13-c495-44ab-829f-0a1bb87e8e56%2F5ee5z0p_processed.png&w=3840&q=75)
Transcribed Image Text:def listsum(numlist, size):
# - base case
# if the list is empty
if size 0:
return 0
# if the list contains a single element
elif size == 1:
return numlist[0]
10
# get the halfway point
11
# so we'll know where to divide the list in half
12
mid =
size//2
13
14
# --recursive step--
15
# add the sum of the left side with the sum of the right side
16
#tip: you need to pass the left side to one function call
17
# and the right side to the other
18
return listsum(numlist[0:mid], Len(numlist[0:mid])) + listsum(numlist[mid:], Len(numlist[mid:]
19
20
21
numbers
=
[3,5,4,1,7,2,9,8,0,6]
22 print(listsum(numbers, Len(numbers)))
1~3
2
3
4
5
6
7
8
9
FROM

Transcribed Image Text:sum(1,3,5,7,9)
sum (3,5,7,9)
sum (5,7,9)
sum (7,9)
1+
3+
-C
sum(9)
5+
10
7+
9
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
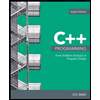
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
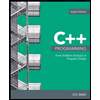
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning