luejack Library is one of the popular libraries in the town. This library has more than 50.000 books. Sadly the senior librarian wants to resign and he doesn’t have time to teach the new librarian. Bluejack Library needs a simple program to help the new librarian so he can easily manage and search books. Bluejack Library hires you as a programmer to help them create a program that can help a new librarian find and manage books in this library easily using a C programming language and hashtable data structure. The criteria of the program are: The program consists of 4 menus, there are: View Book Insert Book Remove Book Exit If the user chooses View Book (Menu 1), then:
Subject : Data Structure
Language : C
Topic : Hash Table
Sub Topic :
- Pop
- Push
- Search
Case :
Bluejack Library is one of the popular libraries in the town. This library has more than 50.000 books. Sadly the senior librarian wants to resign and he doesn’t have time to teach the new librarian. Bluejack Library needs a simple program to help the new librarian so he can easily manage and search books. Bluejack Library hires you as a programmer to help them create a program that can help a new librarian find and manage books in this library easily using a C programming language and hashtable data structure. The criteria of the program are:
- The program consists of 4 menus, there are:
- View Book
- Insert Book
- Remove Book
- Exit
- If the user chooses View Book (Menu 1), then:
* If there is no book, then show “There is no book(s) !” message
* Otherwise, the program will show all the book data
- If the user chooses Insert Book (Menu 2), then:
- The program will ask user to input the following data:
* Book Title
* Validate Book Title must be between 5 and 50 characters
* Validate Book Title must be unique
* Book Author
* Validate Book Author must start with “ “ or “Mrs. “ and its length must be between 3 and 25 characters
* ISBN
* Validate ISBN must be numeric and its length must be between 10 and 13 digits
* Page Number
* Validate Page Number must be at least 16
- After that, the program will generate a unique id for the inputted book data. The format will follow the following formula:
[Book ID] : [BXXXXX]-[ISBN]-[A][T]
X: The last inserted Book Id number increased by 1
A: The first character of Book Author in uppercase format
T: The first character of Book Title in uppercase format
For example:
The last inserted Book Id is B00001-0123423123-JH
Then the newly inserted Book Id will be B00002-……
- Then, the program will store the inputted new book data to the next item of the last item of the chaining hash table with size 1007 using the following hash function
Key = SUM % SIZE
Key : the hash table index that will store the data
SUM : The sum of the ascii from Book Id
SIZE : The size of the hash table (1007)
Example:
Book Id : B00001-0123423123-JH
SUM : 1044
Size : 1007
Key : 1044 % 1007 = 37
Then the book data will be stored at index 37 of hash table
- If user chooses Remove Book (Menu 3), then:
- The program will ask user to input a Book ID
* Validate if the inputted Book ID doesn’t exists then show “Book not found”
* Otherwise, show the book data and ask the user for confirmation. Validate input must be either “y” or “n”
- If user chooses “y”, then delete the data
- Otherwise, if user chooses “n” return back to main menu
- If user chooses Exit (Menu 4), then terminate the program
![Book Title
Input book title[5-50][unique]: Don't Make Me Think
The book title is already exists !
Input book title[5-50][unique]: Agile Project Management With Scrum
Input author name[3-25][Mr. IMrs. ]: Ken Schwaber
Input author name[3-25][Mr. İMrs. j: Mr. Ken Schwaber
Input ISBN[10-13][numeric]: test123123
Input ISBN[10-13][numeric]: 9780735619937
Input page number[>- 16]: 15
Input page number[>- 16]: 192
• Validate Book Title must be between 5 and 50 characters
• Validate Book Title must be unique
Book Author
• Validate Book Author must start with “Mr. “ or “Mrs. “ and its length must be
between 3 and 25 characters
Insert success !
ISBN
Figure 4. Insert Menu
• Validate ISBN must be numeric and its length must be between 10 and 13 digits
Page Number
If user chooses Remove Book (Menu 3), then:
• Validate Page Number must be at least 16
> The program will ask user to input a Book ID
> After that, the program will generate a unique id for the inputted book data. The format
Validate if the inputted Book ID doesn't exists then show "Book not found"
will follow the following formula:
Input book id to delete: Be0001-0123423123-JH
[Book ID] : [BXXXXX]-[ISBN]-[A][T]
X: The last inserted Book Id number increased by 1
Book not found !
Figure 5. The Inputted Book ID Doesn't Exists
A: The first character of Book Author in uppercase format
Otherwise, show the book data and ask the user for confirmation. Validate input must
T: The first character of Book Title in uppercase format
be either "y" or "n"
For example:
> If user chooses "y", then delete the data
The last inserted Book Id is B00001-0123423123-JH
> Otherwise, if user chooses “n" return back to main menu
Then the newly inserted Book Id will be B00002-..
Input book id to delete: BO0002-9780735619937-AM
> Then, the program will store the inputted new book data to the next item of the last item
Book Id: B00002-9780735619937-AM
Book Title: Agile Project Management With Scrum
Book Author: Mr. Ken Schwaber
Book ISBN: 9780735619937
Page Number: 192
Are you sure [yln]? Y
Are you sure (yln]? y
of the chaining hash table with size 1007 using the following hash function
Key = SUM % SIZE
Key : the hash table index that will store the data
SUM : The sum of the ascii from Book Id
SIZE : The size of the hash table (1007)
Delete success !
Figure 6. Confirmation To Delete
Example:
Book Id
B00001-0123423123-JH
: 1044
: 1007
1044 % 1007 = 37
SUM
If user chooses Exit (Menu 4), then terminate the program
Size
Key
Then the book data will be stored at index 37 of hash table
TVT C](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F437cf630-ee2c-4006-997f-853be3e29e41%2Fb7e80db2-da1a-49f7-a298-62cd97b59ab0%2F7l3kf5i_processed.png&w=3840&q=75)
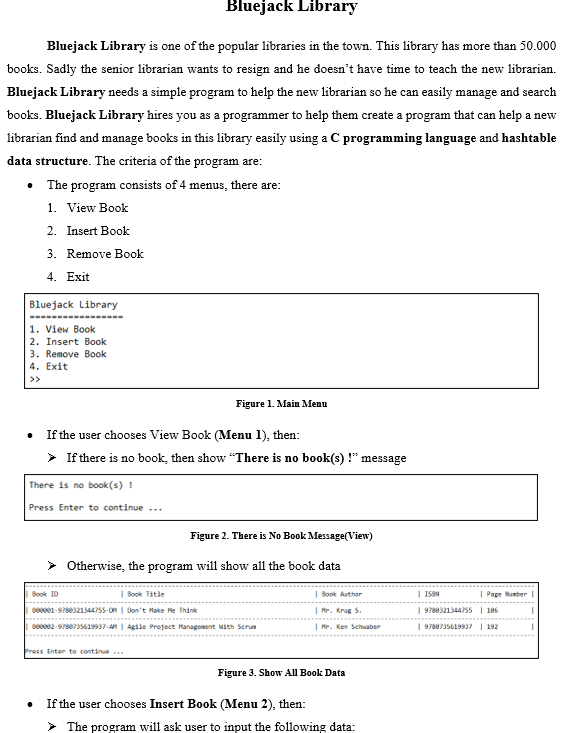

Step by step
Solved in 2 steps
