(Main java file) package P3Q4; import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.*; public class P3Q4 extends JFrame{ private JLabel jtaMessage=new JLabel(); private JLabel jtaText=new JLabel("Enter your password"); private JPasswordField jpfPassword = new JPasswordField(); private JButton jtnSubmit = new JButton("Submit"); private JLabel jtaEmpty = new JLabel(""); public P3Q4(){ JPanel passwordPanel= new JPanel(new GridLayout(2,2)); JPanel messagePanel= new JPanel(new FlowLayout(FlowLayout.LEFT,0,0)); messagePanel.setBackground(Color.WHITE); jtaText.setFont(new Font("Arial",Font.BOLD,14)); jtnSubmit.setFont(new Font("Arial",Font.BOLD,14)); jtaMessage.setFont(new Font("Arial",Font.BOLD,14)); jtaMessage.setVerticalAlignment(JLabel.TOP); jtaMessage.setPreferredSize(new Dimension(430,110)); passwordPanel.add(jtaText); passwordPanel.add(jpfPassword); passwordPanel.add(jtaEmpty); passwordPanel.add(jtnSubmit); messagePanel.add(jtaMessage); add(passwordPanel,BorderLayout.CENTER); add(messagePanel,BorderLayout.SOUTH); jtnSubmit.addActionListener(new ButtonListener()); setResizable(false); setTitle("Set Password"); setSize(400, 240); setLocationRelativeTo(null); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setVisible(true); } private class ButtonListener implements ActionListener { @Override public void actionPerformed(ActionEvent e) { String inputStr = new String(jpfPassword.getPassword()); try{ Password pw = new Password(inputStr); jtaMessage.setForeground(Color.BLUE); jtaMessage.setText("Congratulations! Your password is valid. "); } catch(InvalidPasswordException ex){ jtaMessage.setForeground(Color.RED); jtaMessage.setText(ex.getMessage()); jpfPassword.grabFocus(); } } } public static void main(String[] args) { new P3Q4(); } } (InvalidPasswordException) package P3Q4; public class InvalidPasswordException extends Exception{ public InvalidPasswordException(){ super("Invalid Password Exception"); } public InvalidPasswordException(String errMsg){ super(errMsg); } } (Password) package P3Q4; public class Password { public Password (String passwordStr) throws InvalidPasswordException { if (passwordStr== null ||passwordStr.equals("")) { throw new InvalidPasswordException("Invalid password: password cannot be null or an empty string therefore please key-in the correct password."); } else { int countDigits=0,countLetters=0,total=0; for(int i=0;i
Based on the java code given how to draw the program user interface design ?
(Main java file) package P3Q4; import java.awt.*; public class P3Q4 extends JFrame{ private JLabel jtaMessage=new JLabel(); @Override |
(InvalidPasswordException) package P3Q4; public class InvalidPasswordException extends Exception{ public InvalidPasswordException(){ |
(Password) package P3Q4; public class Password {
|
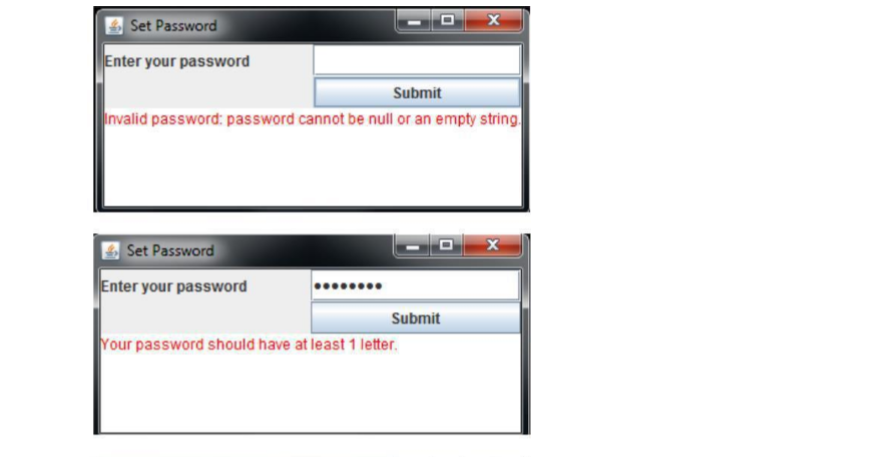
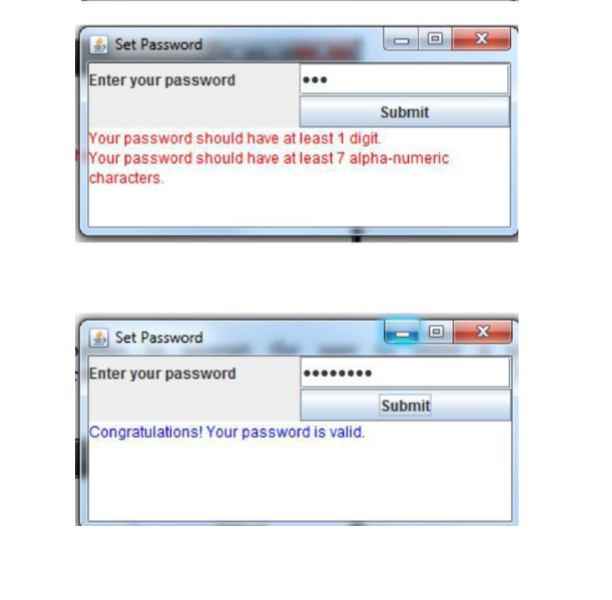

Step by step
Solved in 2 steps with 2 images

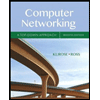
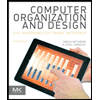
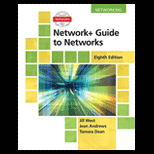
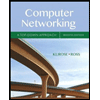
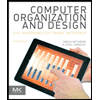
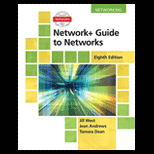
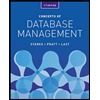
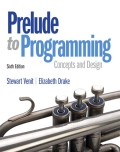
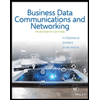