Make a class called Wallet. Wallet must hold the following 2 public attributes: int moneyAmt string owner Include 2 constructors, 1 default constructor and a 2-arg constructor in the order of amt then owner (see examples). The default constructor must assign the values 0, and "No Funds" to moneyAmt and owner, respectively. You must also add code to Wallet so that the following code will compile and compare 2 Wallet objects with the greater-than operator so that the Wallet with a greater moneyAmt is greater than the one with a lesser amount. Should both Wallet objects have the same amount, the one with the greater owner name is greater. For instance Wallet kar(9, "Karl"), al(9, "Alice"); In the above, kar must compare as greater than al. In other words, the following expression must be true: kar > al Also, Wallet must be able to compare not equals. Two Wallet objects are not equal if their moneyAmt and owner are different Examine the code in the main template and make it work without changing it. You may change it to test, but ensure you load the template and submit that to ensure everything is working as expected and that the output test passes. You only need add code to Wallet.h and Wallet.cpp
Make a class called Wallet. Wallet must hold the following 2 public attributes:
int moneyAmt
string owner
Include 2 constructors, 1 default constructor and a 2-arg constructor in the order of amt then owner (see examples). The default constructor must assign the values 0, and "No Funds" to moneyAmt and owner, respectively.
You must also add code to Wallet so that the following code will compile and compare 2 Wallet objects with the greater-than operator so that the Wallet with a greater moneyAmt is greater than the one with a lesser amount. Should both Wallet objects have the same amount, the one with the greater owner name is greater. For instance
Wallet kar(9, "Karl"), al(9, "Alice");
In the above, kar must compare as greater than al. In other words, the following expression must be true:
kar > al
Also, Wallet must be able to compare not equals. Two Wallet objects are not equal if their moneyAmt and owner are different
Examine the code in the main template and make it work without changing it. You may change it to test, but ensure you load the template and submit that to ensure everything is working as expected and that the output test passes.
You only need add code to Wallet.h and Wallet.cpp
Hints. Examine the code to determine which operators need to be overloaded. If you overload more than 2 operators, you are doing too much. The string class has overloaded all boolean operators to work as alphabetical order.
main.cpp:
#include<iostream>
#include<string>
using namespace std;
#include "Wallet.h"
int main()
{
//ALL of the following code must work and output:
/*
Normal relationship
different
Zoe is first, then Alvin
*/
//Examine the code so you can know what must work.
//You can change this to test, but ensure you refresh the template before submitting
Wallet reg, poor(2, "Pennywise"), rich(27734, "Goldman"), owes(-3, "Borrower");
Wallet *first = new Wallet(50, "Zoe"), *second = new Wallet(50, "Alvin");
if( poor > rich)
cout << "Something wrong";
else
cout << "Normal relationship";
cout << endl;
if(owes != reg)
cout << "different";
else
cout << "Something wrong";
cout << endl;
if(*first > *second) //this must evaluate to true since both have 50, but Zoe is after Alvin alphabetically
cout << first->owner << " is first, then " << second->owner;
else
cout << "Something not correct, Zoe should be first";
cout << endl;
return 0;
}
wallet.h:
#include<string>
using namespace std;
//finish the Wallet class prototype
class Wallet
{
public:
int moneyAmt;
string owner;
};
Wallet.cpp:
#include "Wallet.h"
//Implement any Wallet methods here

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

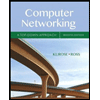
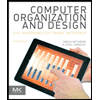
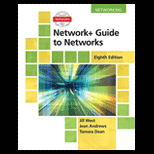
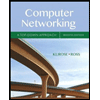
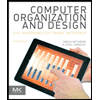
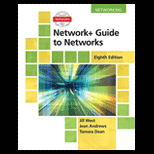
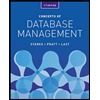
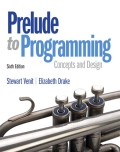
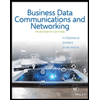