MarkovHodel MarkovModel (text, k) constructs a Markov model = of order x from text returns the order of = returns the number of occurrences of kgran in = returns the number of times character e follows kgra in = using =, finds and returns a random character following kgram using =, builds and returns a string of length n, the first characters of which is kgram 2.order () z. kgran freq (kgran) 2. char treq(kgran, e) z.rand (kgraz) z. gen (kgran, n) • Constructor To implement the data type, define two instance variables: an integer k that stores the order of the Markov model, and a symbol table _at whose keys are all the k-grams from the given text. The value corresponding to each key (say kgram) in et is a symbol table whose keys are the characters that follow kgram in the text, and the corresponding values are their frequencies. You may assume that the input text is a sequence of characters over the ASCII alphabet so that all values are between 0 and 127. The frequencies should be tallied as if the text were circular (i.e., as if it repeated the first k characters at the end). For example, if the text is 'gaggagaggegagaaa' and k = 2, then the symbol table _at should store the following information: 'aa': {'a': 1, 'g': 1}, 'ag': {'a': 3, 'g': 2}, 'cg': {'a': 1}, 'ga': {'a': 1. '8': 4}, 'ge': {'g'i 1}. '88': {'a': 1, 'c': 1, 'g': 1} If you are careful enough, the entire symbol table can be built in just one pass through the circular text. Note that there is no reason to save the original text or the circular text as an attribute of the data type. That would be a grossly
MarkovHodel MarkovModel (text, k) constructs a Markov model = of order x from text returns the order of = returns the number of occurrences of kgran in = returns the number of times character e follows kgra in = using =, finds and returns a random character following kgram using =, builds and returns a string of length n, the first characters of which is kgram 2.order () z. kgran freq (kgran) 2. char treq(kgran, e) z.rand (kgraz) z. gen (kgran, n) • Constructor To implement the data type, define two instance variables: an integer k that stores the order of the Markov model, and a symbol table _at whose keys are all the k-grams from the given text. The value corresponding to each key (say kgram) in et is a symbol table whose keys are the characters that follow kgram in the text, and the corresponding values are their frequencies. You may assume that the input text is a sequence of characters over the ASCII alphabet so that all values are between 0 and 127. The frequencies should be tallied as if the text were circular (i.e., as if it repeated the first k characters at the end). For example, if the text is 'gaggagaggegagaaa' and k = 2, then the symbol table _at should store the following information: 'aa': {'a': 1, 'g': 1}, 'ag': {'a': 3, 'g': 2}, 'cg': {'a': 1}, 'ga': {'a': 1. '8': 4}, 'ge': {'g'i 1}. '88': {'a': 1, 'c': 1, 'g': 1} If you are careful enough, the entire symbol table can be built in just one pass through the circular text. Note that there is no reason to save the original text or the circular text as an attribute of the data type. That would be a grossly
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:Problem 1. (Markov Model Data Type) Define a data type called xarkovModel in zarkov _zodel.py to represent a Markov model
of order k from a given text string. The data type must support the following API:
Markovlodel
MarkovModel (text, k)
constructs a Markov model = of order z from text
2.order ()
returns the order of =
returns the number of occurrences of kgram in =
returns the number of times character e follows kgra in =
using =, finds and returns a random character following kgraz
using =, builds and returns a string of length n, the first x characters of which is zgraz
z. kgran freq(kgram)
z. char_treq(kgran, c)
z.rand (kgraz)
z. gen (kgran, n)
• Constructor To implement the data type, define two instance variables: an integer k that stores the order of the
Markov model, and a symbol table _at whose keys are all the k-grams from the given text. The value corresponding
to each key (say kgram) in _at is a symbol table whose keys are the characters that follow kgram in the text, and the
corresponding values are their frequencies. You may assume that the input text is a sequence of characters over the
ASCII alphabet so that all values are between 0 and 127. The frequencies should be tallied as if the text were circular
(i.e., as if it repeated the first k characters at the end). For example, if the text is 'gagggageggegagaaa' and k = 2, then the
symbol table _at should store the following information:
'aa': {'a': 1, 'g': 1},
'ag': {'a': 3. '8': 2}.
cg': {'a': 1},
'ga': {'a': 1. '8': 43,
'ge': {'g': 1}.
'gg': {'a': 1, 'c': 1, 'g': 1}
If you are careful enough, the entire symbol table can be built in just one pass through the circular text. Note that
there is no reason to save the original text or the circular text as an attribute of the data type. That would be a grossly
inefficient waste of space. Your Markovkodel object does not need either of these strings after the symbol table is built.
• Order. Return the order k of the Markov Model.
3 /8
Project 6 (Markov Model)
Frequency. There are two frequency methods.
- kgraz treq (kgran) returns the number of times kgram was found in the original text. Returns 0 when kgram is not
found. Raises an error if kgram is not of length k.
- char_treq(kgran, c) returns the number of times kgram was followed by the character e in the original text. Returns
O when kgram or e is not found. Raises an error if kgram is not of length k.
• Randomly generate a character. Return a character. It must be a character that followed the kgram in the original
text. The character should be chosen randomly, but the results of calling rand (kgran) several times should mirror the
frequencies of characters that followed the kgram in the original text. Raise an error if kgram is not of length k or if
kgram is unknown.
• Generate pseudo-random text. Return a string of length n that is a randomly generated stream of characters whose first
k characters are the argument kgram. Starting with the argument kgram, repeatedly call rand() to generate the next
character. Successive k-grams should be formed by using the most recent k characters in the newly generated text.
To avoid dead ends, treat the input text as a circular string: the last character is considered to precede the first character.
For example, if k = 2 and the text is the 17-character string 'geggagaggegagaaa', then the salient features of the Markov model
are captured in the table below:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
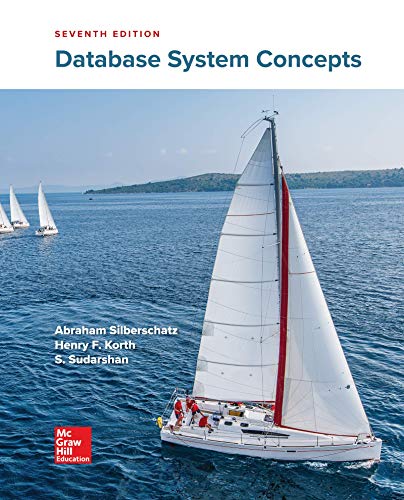
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
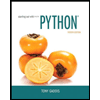
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
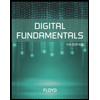
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
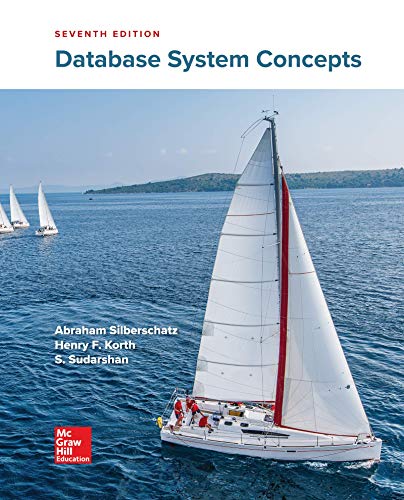
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
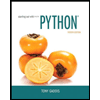
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
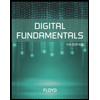
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
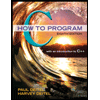
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
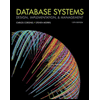
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
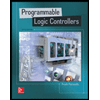
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education