Modify program P34 1.cpp to compute the side area, total area, and volume of a cylinder and the area and volume of a sphere, depending on the choice that the user makes. Your program should ask users to enter 1 to choose cylinder or 2 for sphere, and display an "invalid choice error" for other values. For a cylinder, we want to compute: Side area: (2*PI*r) * h Total Area: 2*(PI*r2) + Side area Volume: (PI*r2)*h For a sphere, we want to compute: Surface area: 4*PI*r2 Volume: (4.0/3.0)*PI*r3. Use overloading whenever possible.
Modify program P34 1.cpp to compute the side area, total area, and volume of a cylinder and the area and volume of a sphere, depending on the choice that the user makes. Your program should ask users to enter 1 to choose cylinder or 2 for sphere, and display an "invalid choice error" for other values. For a cylinder, we want to compute: Side area: (2*PI*r) * h Total Area: 2*(PI*r2) + Side area Volume: (PI*r2)*h For a sphere, we want to compute: Surface area: 4*PI*r2 Volume: (4.0/3.0)*PI*r3. Use overloading whenever possible.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter4: Selection Structures
Section: Chapter Questions
Problem 14PP
Related questions
Question
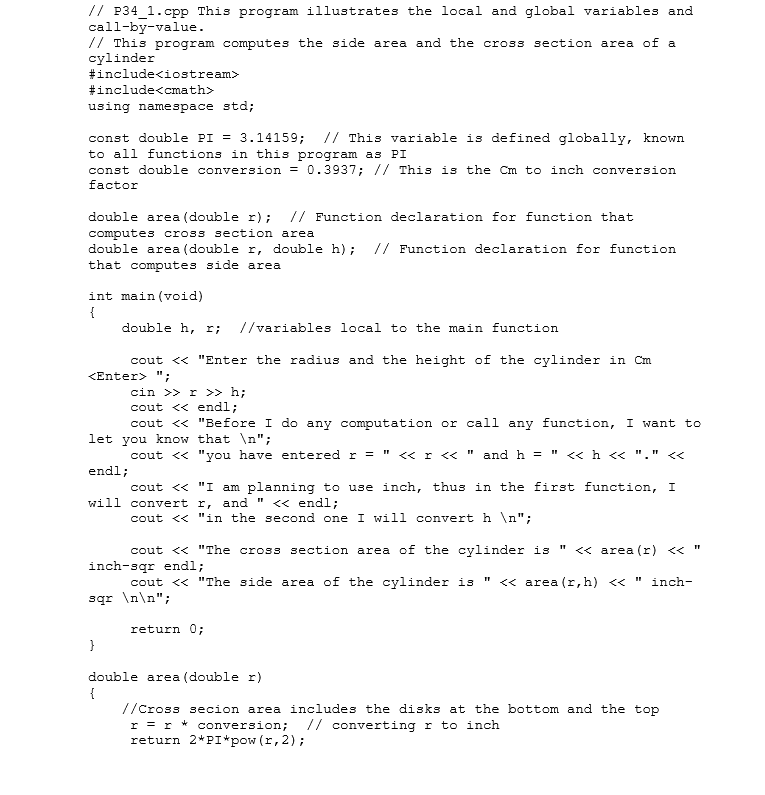
Transcribed Image Text:// P34 1.cpp This program illustrates the local and global variables and
call-by-value.
// This program computes the side area and the cross section area of a
cylinder
#include<iostream>
#include<cmath>
using namespace std;
// This variable is defined globally, known
const double PI = 3.14159;
to all functions in this program as PI
const double conversion = 0.3937; // This is the Cm to inch conversion
factor
double area (double r);
// Function declaration for function that
computes cross section area
double area (double r, double h);
that computes side area
// Function declaration for function
int main (void)
{
double h, r;
//variables local to the main function
cout << "Enter the radius and the height of the cylinder in Cm
<Enter> ";
cin >> r >> h;
cout << endl;
cout << "Before I do any computation or call any function, I want to
let you know that \n";
cout << "you have entered r = " «r <« " and h = " <« h << "." <«
endl;
cout << "I am planning to use inch, thus in the first function, I
will convert r, and " << endl;
cout << "in the second one I will convert h \n";
cout << "The cross section area of the cylinder is
inch-sqr endl;
cout << "The side area of the cylinder is
sqr \n\n";
<< area (r) << "
<< area (r,h) << " inch-
return 0;
}
double area (double r)
{
//Cross secion area includes the disks at the bottom and the top
r = r * conversion;
return 2*PI*pow (r, 2);
// converting r to inch
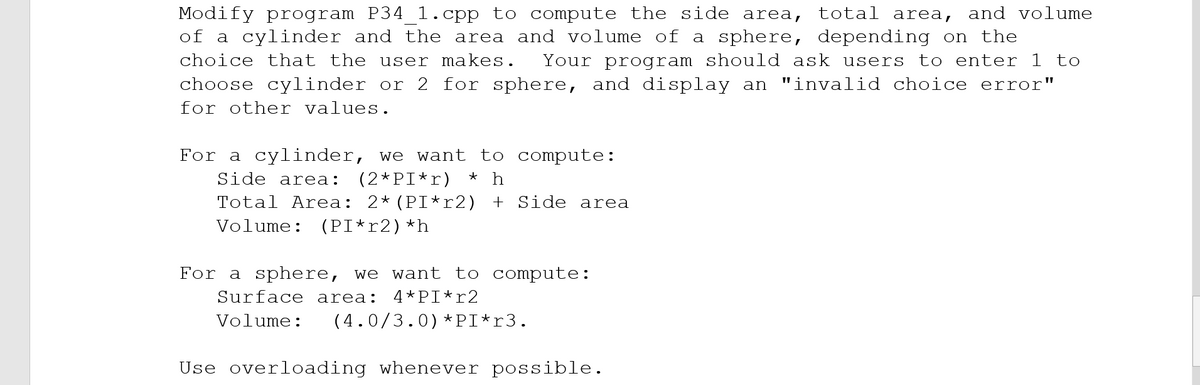
Transcribed Image Text:Modify program P34_1.cpp to compute the side area,
of a cylinder and the area and volume of a sphere, depending on the
choice that the user makes.
total area, and volume
Your program should ask users to enter 1 to
choose cylinder or 2 for sphere, and display an "invalid choice error"
for other values.
For a cylinder, we want to compute:
Side area:
(2*PI*r)
h
Total Area: 2*(PI*r2) + Side area
Volume:
(PI*r2)*h
For a sphere, we want to compute:
Surface area:
4 *PI*r2
Volume:
(4.0/3.0)*PI*r3.
Use overloading whenever possible.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
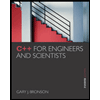
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
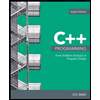
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
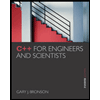
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
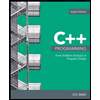
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning