Modify the C++ class for the abstract stack type shown below to use a linked list representation and test it with the same code that appears in this chapter. #include class Stack { private: //** These members are visible only to other //** members and friends (see Section 11.6.4) int *stackPtr; int maxLen; int topSub; public: //** These members are visible to clients Stack() { //** A constructor stackPtr = new int [100]; maxLen = 99; topSub = -1; } ~Stack() {delete [] stackPtr;}; //** A destructor void push(int number) { if (topSub == maxLen) cerr << "Error in push--stack is full\n"; else stackPtr[++topSub] = number; } void pop() { if (empty()) cerr << "Error in pop--stack is empty\n"; else topSub--; } int top() { if (empty()) cerr << "Error in top--stack is empty\n"; else return (stackPtr[topSub]); } int empty() {return (topSub == -1);} }
Modify the C++ class for the abstract stack type shown below
to use a linked list representation and test it with the same code that
appears in this chapter.
#include <iostream.h>
class Stack {
private: //** These members are visible only to other
//** members and friends (see Section 11.6.4)
int *stackPtr;
int maxLen;
int topSub;
public: //** These members are visible to clients
Stack() { //** A constructor
stackPtr = new int [100];
maxLen = 99;
topSub = -1;
}
~Stack() {delete [] stackPtr;}; //** A destructor
void push(int number) {
if (topSub == maxLen)
cerr << "Error in push--stack is full\n";
else stackPtr[++topSub] = number;
}
void pop() {
if (empty())
cerr << "Error in pop--stack is empty\n";
else topSub--;
}
int top() {
if (empty())
cerr << "Error in top--stack is empty\n";
else
return (stackPtr[topSub]);
}
int empty() {return (topSub == -1);}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

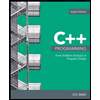
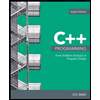