Modify the code below to create a "Dice game" program that is contained in 5 files: Dic_game.cpp Dice.cpp Die.cpp Dice.h Die.h ou must include these features: Try-Throw-Catch Use .h and .cpp files for classes Must be your own code written now Dice Game: In DrA’s dice game, you use two dice. On the first roll of the dice the player immediately wins when the roll is 7 or 11, and loses when the roll is 2, 3, or 12. If 4, 5, 6, 8, 9, or 10 is rolled that number becomes the “key." The player keeps rolling the dice until either 7 or the key is rolled. If the key is rolled first, then the player loses. If the player rolls a 7 first, then the player wins. (There is a loop here.) Write a program that plays the game once, using the rules stated above. There is no user input. Run it a few times watching the behavior in the debugger until you are convinced that it is playing by the rules. Instead of asking for a wager, the program should just determine if the player would win or lose. The program should simulate rolling the two dice and calculate the sum. code to be modified: Dice_Game.cpp #include #include "Die.h" using namespace std; // a struct for game variables struct GameState { int turn = 1; int score = 0; int score_this_turn = 0; bool turn_over = false; bool game_over = false; Die die; }; // declare functions void display_rules(); void play_game(GameState&); void take_turn(GameState&); void roll_die(GameState&); void hold_turn(GameState&); int main() { display_rules(); GameState game; play_game(game); } // define functions void display_rules() { cout << "Dice Game Rules: \n" << "\n" << "* See how many turns it takes you to get to 20.\n" << "* Turn ends when you hold or roll a 1.\n" << "* If you roll a 1, you lose all points for the turn.\n" << "* If you hold, you save all points for the turn.\n\n"; } void play_game(GameState& game) { while (!game.game_over) { take_turn(game); } cout << "Game over!\n"; } void take_turn(GameState& game) { cout << "TURN " << game.turn << endl; game.turn_over = false; while (!game.turn_over) { char choice; cout << "Roll or hold? (r/h): "; cin >> choice; if (choice == 'r') roll_die(game); else if (choice == 'h') hold_turn(game); else cout << "Invalid choice. Try again.\n"; } } void roll_die(GameState& game) { game.die.roll(); cout << "Die: " << game.die.getValue() << endl; if (game.die.getValue() == 1) { game.score_this_turn = 0; game.turn += 1; game.turn_over = true; cout << "Turn over. No score.\n\n"; } else { game.score_this_turn += game.die.getValue(); } } void hold_turn(GameState& game) { game.score += game.score_this_turn; game.turn_over = true; cout << "Score for turn: " << game.score_this_turn << endl; cout << "Total score: " << game.score << "\n\n"; game.score_this_turn = 0; if (game.score >= 20) { game.game_over = true; cout << "You finished in " << game.turn << " turns!\n\n"; } else { game.turn += 1; } } Dice.cpp #include "Dice.h" Dice::Dice() {} void Dice::add_die(Die die) { dice.push_back(die); } void Dice::roll_all() { for (Die& die : dice) { die.roll(); } } std::vector Dice::get_dice() const { return dice; } Die.cpp #include #include #include "Die.h" Die::Die() { srand(time(NULL)); // seed the rand() function value = 1; } void Die::roll() { value = rand() % 6; // value is >= 0 and <= 5 ++value; // value is >= 1 and <= 6 } int Die::getValue() { return value; } Dice.h ifndef DICE_H #define DICE_H #include #include "Die.h" class Dice { private: std::vector dice; public: Dice(); void add_die(Die die); void roll_all(); std::vector get_dice() const; }; #endif // DICE_H Die.h #ifndef DIE_H #define DIE_H class Die { private: int value; public: Die(); void roll(); int getValue(); }; #endif
Modify the code below to create a "Dice game" program that is contained in 5 files:
- Dic_game.cpp
- Dice.cpp
- Die.cpp
- Dice.h
- Die.h
ou must include these features:
- Try-Throw-Catch
- Use .h and .cpp files for classes
- Must be your own code written now
Dice Game: In DrA’s dice game, you use two dice. On the first roll of the dice the player immediately wins when the roll is 7 or 11, and loses when the roll is 2, 3, or 12.
If 4, 5, 6, 8, 9, or 10 is rolled that number becomes the “key."
The player keeps rolling the dice until either 7 or the key is rolled. If the key is rolled first, then the player loses. If the player rolls a 7 first, then the player wins. (There is a loop here.)
Write a program that plays the game once, using the rules stated above. There is no user input. Run it a few times watching the behavior in the debugger until you are convinced that it is playing by the rules.
Instead of asking for a wager, the program should just determine if the player would win or lose. The program should simulate rolling the two dice and calculate the sum.
code to be modified:
Dice_Game.cpp
#include <iostream>
#include "Die.h"
using namespace std;
// a struct for game variables
struct GameState
{
int turn = 1;
int score = 0;
int score_this_turn = 0;
bool turn_over = false;
bool game_over = false;
Die die;
};
// declare functions
void display_rules();
void play_game(GameState&);
void take_turn(GameState&);
void roll_die(GameState&);
void hold_turn(GameState&);
int main()
{
display_rules();
GameState game;
play_game(game);
}
// define functions
void display_rules()
{
cout << "Dice Game Rules: \n"
<< "\n"
<< "* See how many turns it takes you to get to 20.\n"
<< "* Turn ends when you hold or roll a 1.\n"
<< "* If you roll a 1, you lose all points for the turn.\n"
<< "* If you hold, you save all points for the turn.\n\n";
}
void play_game(GameState& game)
{
while (!game.game_over)
{
take_turn(game);
}
cout << "Game over!\n";
}
void take_turn(GameState& game)
{
cout << "TURN " << game.turn << endl;
game.turn_over = false;
while (!game.turn_over)
{
char choice;
cout << "Roll or hold? (r/h): ";
cin >> choice;
if (choice == 'r')
roll_die(game);
else if (choice == 'h')
hold_turn(game);
else
cout << "Invalid choice. Try again.\n";
}
}
void roll_die(GameState& game)
{
game.die.roll();
cout << "Die: " << game.die.getValue() << endl;
if (game.die.getValue() == 1)
{
game.score_this_turn = 0;
game.turn += 1;
game.turn_over = true;
cout << "Turn over. No score.\n\n";
}
else
{
game.score_this_turn += game.die.getValue();
}
}
void hold_turn(GameState& game)
{
game.score += game.score_this_turn;
game.turn_over = true;
cout << "Score for turn: " << game.score_this_turn << endl;
cout << "Total score: " << game.score << "\n\n";
game.score_this_turn = 0;
if (game.score >= 20)
{
game.game_over = true;
cout << "You finished in " << game.turn << " turns!\n\n";
}
else
{
game.turn += 1;
}
}
Dice.cpp
#include "Dice.h"
Dice::Dice() {}
void Dice::add_die(Die die)
{
dice.push_back(die);
}
void Dice::roll_all()
{
for (Die& die : dice)
{
die.roll();
}
}
std::
{
return dice;
}
Die.cpp
#include <cstdlib>
#include <ctime>
#include "Die.h"
Die::Die()
{
srand(time(NULL)); // seed the rand() function
value = 1;
}
void Die::roll()
{
value = rand() % 6; // value is >= 0 and <= 5
++value; // value is >= 1 and <= 6
}
int Die::getValue()
{
return value;
}
Dice.h
ifndef DICE_H
#define DICE_H
#include <vector>
#include "Die.h"
class Dice
{
private:
std::vector<Die> dice;
public:
Dice();
void add_die(Die die);
void roll_all();
std::vector<Die> get_dice() const;
};
#endif // DICE_H
Die.h
#ifndef DIE_H
#define DIE_H
class Die
{
private:
int value;
public:
Die();
void roll();
int getValue();
};
#endif

Step by step
Solved in 3 steps with 1 images

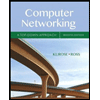
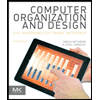
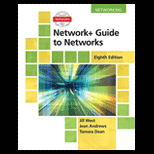
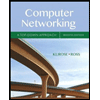
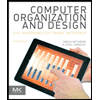
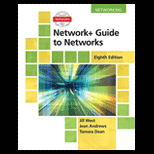
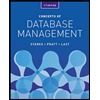
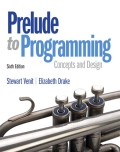
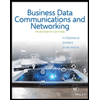