Modify your program created in the previous question so that it also looks for matching open and closing single AND double quotes. You may need to add an additional stack. Consider that matching quotes normally go inside any matching curly braces and parenthesis, but not the other way around. If curly braces or parentheses are found between matching quotes, consider them part of the string and NOT part of the programming code. Also, think about the differences between for what you use single and double quotes. Here is my code:
Computer Science
Modify your program created in the previous question so that it also looks for matching open and closing single AND double quotes. You may need to add an additional stack. Consider that matching quotes normally go inside any matching curly braces and parenthesis, but not the other way around. If curly braces or parentheses are found between matching quotes, consider them part of the string and NOT part of the
Here is my code:
// A small demonstration program for a stack.
#include <iostream> // Provides cin, cout
#include <stack> // Provides stack
#include <string> // Provides string
#include <fstream> //Creates and/or read files
using namespace std;
// PROTOTYPE for a function used by this demonstration program
bool balanceSymbols(const string& expr);
// Postcondition: A true return value indicates that the parentheses in the
// given expression are balanced. Otherwise, the return value is false.
int main()
{
string userInput;
cout << "Enter File name:(Sample 1.txt or Sample 2.txt) to check if symbols are balanced\n";
getline(cin, userInput);
if (balanceSymbols(userInput))
cout << "Those parentheses are balanced.\n";
else
cout << "Those parentheses are not balanced.\n";
return 0;
}
bool balanceSymbols(const string& fileName)
// Library facilities used: stack, string
{
string expression; //to store current line
ifstream inFile(fileName);
stack<char> store; // Stack to store the left parentheses as they occur
bool flag = false; // Becomes true if a needed parenthesis is not found
while (getline(inFile,expression)) {
for (int i = 0; !flag && (i < expression.length()); ++i)
{
if (expression[i] == '(')
store.push(expression[i]);
else if (expression[i] == '{')
store.push(expression[i]);
else if ((expression[i] == ')')) {
// if right paranthesis encountered, match with left paranthesis at top
if (store.empty())
flag = true;
else if (store.top() == '(')
store.pop(); // Pops the corresponding left parenthesis.
else {
flag = true; // if the stack top has other type of paranthesis
}
}
else if ((expression[i] == '}')) {
// if right paranthesis encountered, match with left paranthesis at top
if (store.empty())
flag = true;
else if (store.top() == '{')
store.pop(); // Pops the corresponding left parenthesis.
else {
flag = true; // if the stack top has other type of paranthesis
}
}
}
}
inFile.close();
return (store.empty() && !flag);
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

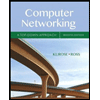
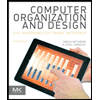
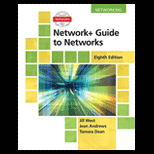
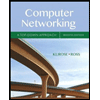
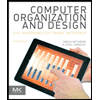
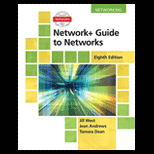
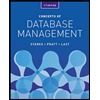
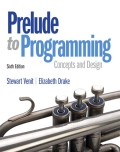
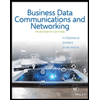