mutex_t lock; // declare a lock cond_t cv; // declare a condition variable A condition variable (CV) is a queue of waiting threads. A single lock is associated with each CV (see below for usage) Two operations for a CV: cond_wait(cond_t *cv, mutex_t *lock) Assumes the lock is held when cond_wait() is called Puts caller to sleep + releases the lock (atomically) When awoken, reacquires lock before returning cond_signal(cond_t *cv) Wake a single waiting thread (if >= 1 thread is waiting) If there is no waiting, return w/o doing anything Using the above functions, please answer the following two questions: 4.8.1. Implementing Join with CV: void thread_exit() { done = 1; cond_signal(&c); } void thread_join() { mutex_lock (&m); if (done == 0) // a // b mutex_unlock (&m); // w // x cond_wait (&c, &m); // y // Z } How do you fix the above implementation using a lock (*m) and CV (*c)? (hint: insert function calls in the code)
mutex_t lock; // declare a lock cond_t cv; // declare a condition variable A condition variable (CV) is a queue of waiting threads. A single lock is associated with each CV (see below for usage) Two operations for a CV: cond_wait(cond_t *cv, mutex_t *lock) Assumes the lock is held when cond_wait() is called Puts caller to sleep + releases the lock (atomically) When awoken, reacquires lock before returning cond_signal(cond_t *cv) Wake a single waiting thread (if >= 1 thread is waiting) If there is no waiting, return w/o doing anything Using the above functions, please answer the following two questions: 4.8.1. Implementing Join with CV: void thread_exit() { done = 1; cond_signal(&c); } void thread_join() { mutex_lock (&m); if (done == 0) // a // b mutex_unlock (&m); // w // x cond_wait (&c, &m); // y // Z } How do you fix the above implementation using a lock (*m) and CV (*c)? (hint: insert function calls in the code)
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter8: Advanced Data Handling Concepts
Section: Chapter Questions
Problem 20RQ
Related questions
Question
please solve / answer within 30 minutes....

Transcribed Image Text:mutex_t lock; // declare a lock
cond_t cv; // declare a condition variable
A condition variable (CV) is a queue of waiting threads.
A single lock is associated with each CV (see below for usage)
Two operations for a CV:
cond_wait(cond_t *cv, mutex_t *lock)
●
Assumes the lock is held when cond_wait() is called
Puts caller to sleep + releases the lock (atomically)
When awoken, reacquires lock before returning
cond_signal(cond_t *cv)
Wake a single waiting thread (if >= 1 thread is waiting)
If there is no waiting, return w/o doing anything
Using the above functions, please answer the following two questions:
4.8.1. Implementing Join with CV:
void thread_exit() {
done = 1;
cond_signal(&c);
}
void thread_join() {
mutex_lock (&m);
if (done == 0)
// a
// b
mutex_unlock (&m);
// w
// x
cond_wait (&c, &m); // y
// z
}
How do you fix the above implementation using a lock (*m) and CV (*c)? (hint: insert
function calls in the code)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
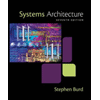
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
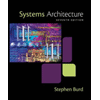
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning