my main.cpp, if there's anything wrong with it in relation to the assignment, please point it out and how to solve it. I can't get my functions.cpp to connect properly to the main.cpp. I need the functions.cpp first, anything else comes second. Thank you very much. main.cpp: #include #include #include #include #include "functions.cpp" using namespace std; //struct for menuItemType
my main.cpp, if there's anything wrong with it in relation to the assignment, please point it out and how to solve it. I can't get my functions.cpp to connect properly to the main.cpp. I need the functions.cpp first, anything else comes second. Thank you very much. main.cpp: #include #include #include #include #include "functions.cpp" using namespace std; //struct for menuItemType
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
This is my main.cpp, if there's anything wrong with it in relation to the assignment, please point it out and how to solve it. I can't get my functions.cpp to connect properly to the main.cpp. I need the functions.cpp first, anything else comes second. Thank you very much.
main.cpp:
#include <iostream>
#include <fstream>
#include <iomanip>
#include <string>
#include "functions.cpp"
using namespace std;
//struct for menuItemType
struct menuItemType
{
string menuItem;
double menuPrice;
}
void getData(menuItemType menuList[]);
void showMenu(menuItemType menuList[], int x);
void printCheck(menuItemType menuList[], int menuOrder[], int x);
int main();
{
// Declare functions and variables
const int menuItems = 8;
menuItemType menuList[menuItems];
int menuOrder[menuItems] = {0};
int orderChoice = 0;
bool ordering = true;
int count = 0;
// Set the menu
getData(menuList);
// Order
showMenu(menuList, menuItems);
while(ordering)
{
cout << "Please enter the number for the item you would\n"
<< "like to order, or enter [0] to complete your order."
<< endl;
cin >> orderChoice;
if (orderChoice > 0 && orderChoice <= menuItems)
{
menuOrder[orderChoice - 1] += 1;
}
else
ordering = false;
}
printCheck(menuList, menuOrder, menuItems);
cin.ignore(2);
return 0;
}
void getData(menuItemType menuList[])
{
// Declare menuItemTypes
menuItemType plainEgg;
menuItemType baconEgg;
menuItemType muffin;
menuItemType frenchToast;
menuItemType fruitBasket;
menuItemType cereal;
menuItemType coffee;
menuItemType tea;
// Initialize menuItemTypes variables
plainEgg.menuItem = "Plain Egg";
plainEgg.menuPrice = 1.45;
baconEgg.menuItem = "Bacon and Egg";
baconEgg.menuPrice = 2.45;
muffin.menuItem = "Muffin";
muffin.menuPrice = 0.99;
frenchToast.menuItem = "French Toast";
frenchToast.menuPrice = 1.99;
fruitBasket.menuItem = "Fruit Basket";
fruitBasket.menuPrice = 2.49;
cereal.menuItem = "Cereal";
cereal.menuPrice = 0.69;
coffee.menuItem = "Coffee";
coffee.menuPrice = 0.50;
tea.menuItem = "Tea";
tea.menuPrice = 0.75;
menuList[0] = plainEgg;
menuList[1] = baconEgg;
menuList[2] = muffin;
menuList[3] = frenchToast;
menuList[4] = fruitBasket;
menuList[5] = cereal;
menuList[6] = coffee;
menuList[7] = tea;
}
void showMenu(menuItemType menuList[], int x)
{
// Function variables
int count;
cout << "Welcome to Bob Evans!" << endl;
cout << "What would you to order?" << endl;
for(count = 0; count < x; count++)
{
cout << setw(2) << left << "[" << count + 1 << "]"
<< menuList[count].menuItem << '$'
<< menuList[count].menuPrice << endl;
}
}
void printCheck(menuItemType menuList[], int menuOrder[], int menuItems)
{
double checkTotal = 0;
double checkTax = 0;
const double TAX = .05;
cout << "Thanks for eating at Bob Evans!"
<< "Customer check: " << endl;
for(int i = 0; i < menuItems; i++)
{
if(menuOrder[i] > 0) {
cout << setprecision(3) << setw(10) << left << menuList[i].menuItem
<< '$' << (menuList[i].menuPrice * menuOrder[i]) << endl;
checkTotal += (menuList[i].menuPrice * menuOrder[i]);
}
}
checkTax = checkTotal * TAX;
checkTotal += checkTax;
cout << setw(14) << left << "Tax" << checkTax << endl
<< setw(14) << left << "Total" << checkTotal << endl;
}
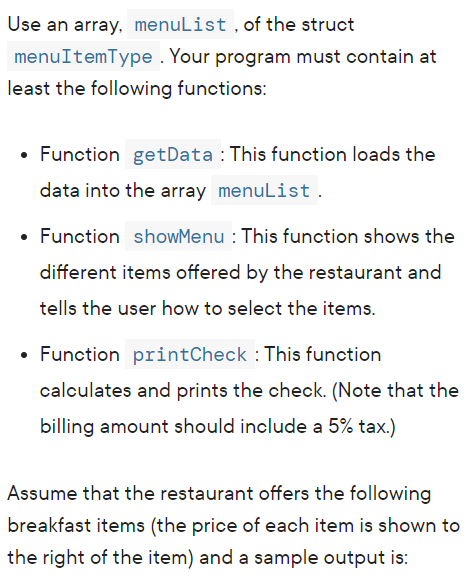
Transcribed Image Text:Use an array, menulist , of the struct
menuItemType . Your program must contain at
least the following functions:
• Function getData : This function loads the
data into the array menulist .
• Function showMenu : This function shows the
different items offered by the restaurant and
tells the user how to select the items.
Function printCheck : This function
calculates and prints the check. (Note that the
billing amount should include a 5% tax.)
Assume that the restaurant offers the following
breakfast items (the price of each item is shown to
the right of the item) and a sample output is:
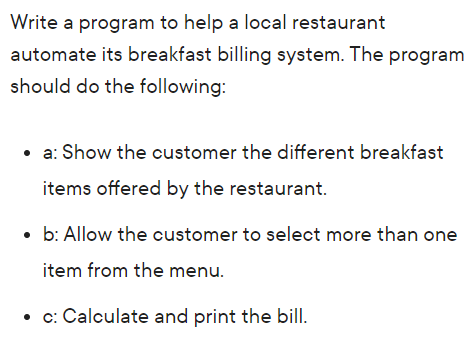
Transcribed Image Text:Write a program to help a local restaurant
automate its breakfast billing system. The program
should do the following:
• a: Show the customer the different breakfast
items offered by the restaurant.
• b: Allow the customer to select more than one
item from the menu.
• c: Calculate and print the bill.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
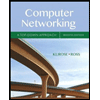
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
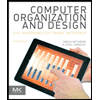
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
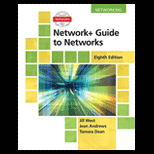
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
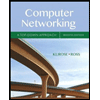
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
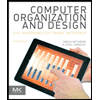
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
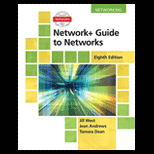
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
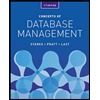
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
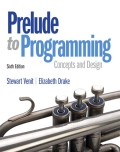
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
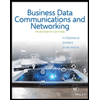
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY