Naval Battleship Game You are a programmer writing code for a naval battleship game. A ship's position is denoted by (x, y) coordinates. The pieces in the game are different kinds of ships. At this point, there are frigates, stealth ships, and submarines. The frigate and submarine are rectangular in shape and the stealth ship is a circle. The ships have differences but also have the following in common: 1. All ships have x and y anchor points. 2. All ships have a name (e.g., USS John Kennedy) 3. All can have missiles fired at them 4. All have a maximum number of missiles strikes they can survive before they are destroyed 5. All can be repositioned to new x and y anchor points. The ship cannot be repositioned if it is destroyed. The anchor point for the frigate and submarine is the lower left hand (x, y) coordinate. They also have (x, y) coordinates for the point that is the upper right-hand corner of the ship. See the figure below. The anchor point and upper right hand corner define the rectangular space that the frigate and submarine occupies. The submarine has a third coordinate (z) which its depth below water. The anchor point of the stealth ship is the center of a circle. It also has a radius. The anchor point together with the radius defines the circular space that the stealth ship occupies. Missiles are shot at a set of x and y coordinates and a depth. The depth represents the distance below water. The frigate and stealth ship are always at depth zero. For a missile to strike a ship, it has to hit it within the shape defined by its coordinates and at the right depth. Missile at a depth below zero cannot hit the stealth ship and frigate. To hit a submarine, the missile has to strike within the rectangle defined by the coordinates and also at the exact depth. The stealth ship has shields that can be raised and lowered. If the shields are up, missile cannot strike the stealth ship. For each concrete class (non-abstract), write a menu-based main method that can be used to test the class.
Naval Battleship Game You are a programmer writing code for a naval battleship game. A ship's position is denoted by (x, y) coordinates. The pieces in the game are different kinds of ships. At this point, there are frigates, stealth ships, and submarines. The frigate and submarine are rectangular in shape and the stealth ship is a circle. The ships have differences but also have the following in common: 1. All ships have x and y anchor points. 2. All ships have a name (e.g., USS John Kennedy) 3. All can have missiles fired at them 4. All have a maximum number of missiles strikes they can survive before they are destroyed 5. All can be repositioned to new x and y anchor points. The ship cannot be repositioned if it is destroyed. The anchor point for the frigate and submarine is the lower left hand (x, y) coordinate. They also have (x, y) coordinates for the point that is the upper right-hand corner of the ship. See the figure below. The anchor point and upper right hand corner define the rectangular space that the frigate and submarine occupies. The submarine has a third coordinate (z) which its depth below water. The anchor point of the stealth ship is the center of a circle. It also has a radius. The anchor point together with the radius defines the circular space that the stealth ship occupies. Missiles are shot at a set of x and y coordinates and a depth. The depth represents the distance below water. The frigate and stealth ship are always at depth zero. For a missile to strike a ship, it has to hit it within the shape defined by its coordinates and at the right depth. Missile at a depth below zero cannot hit the stealth ship and frigate. To hit a submarine, the missile has to strike within the rectangle defined by the coordinates and also at the exact depth. The stealth ship has shields that can be raised and lowered. If the shields are up, missile cannot strike the stealth ship. For each concrete class (non-abstract), write a menu-based main method that can be used to test the class.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Pls, explain how this
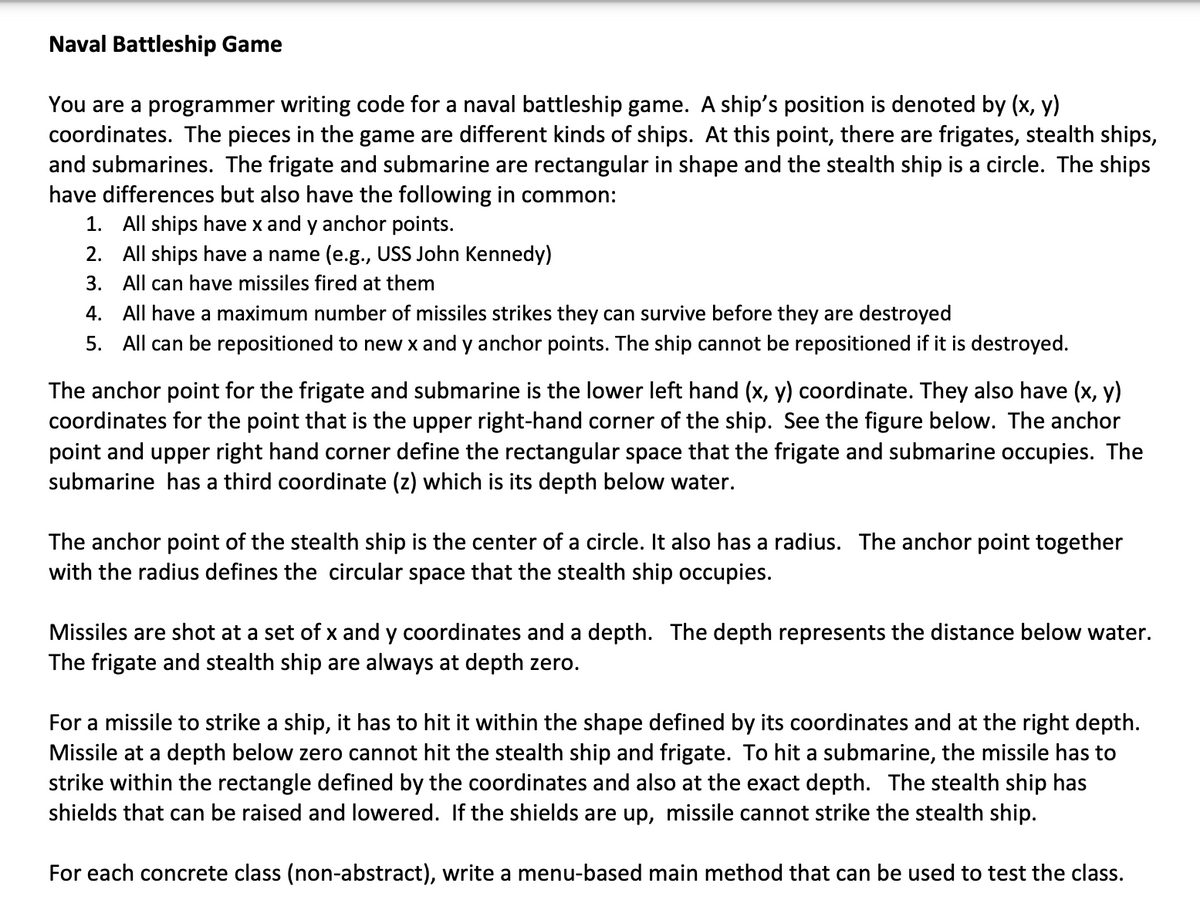
Transcribed Image Text:Naval Battleship Game
You are a programmer writing code for a naval battleship game. A ship's position is denoted by (x, y)
coordinates. The pieces in the game are different kinds of ships. At this point, there are frigates, stealth ships,
and submarines. The frigate and submarine are rectangular in shape and the stealth ship is a circle. The ships
have differences but also have the following in common:
1. All ships have x and y anchor points.
2.
All ships have a name (e.g., USS John Kennedy)
3.
All can have missiles fired at them
4. All have a maximum number of missiles strikes they can survive before they are destroyed
5.
All can be repositioned to new x and y anchor points. The ship cannot be repositioned if it is destroyed.
The anchor point for the frigate and submarine is the lower left hand (x, y) coordinate. They also have (x, y)
coordinates for the point that is the upper right-hand corner of the ship. See the figure below. The anchor
point and upper right hand corner define the rectangular space that the frigate and submarine occupies. The
submarine has a third coordinate (z) which is its depth below water.
The anchor point of the stealth ship is the center of a circle. It also has a radius. The anchor point together
with the radius defines the circular space that the stealth ship occupies.
Missiles are shot at a set of x and y coordinates and a depth. The depth represents the distance below water.
The frigate and stealth ship are always at depth zero.
For a missile to strike a ship, it has to hit it within the shape defined by its coordinates and at the right depth.
Missile at a depth below zero cannot hit the stealth ship and frigate. To hit a submarine, the missile has to
strike within the rectangle defined by the coordinates and also at the exact depth. The stealth ship has
shields that can be raised and lowered. If the shields are up, missile cannot strike the stealth ship.
For each concrete class (non-abstract), write a menu-based main method that can be used to test the class.
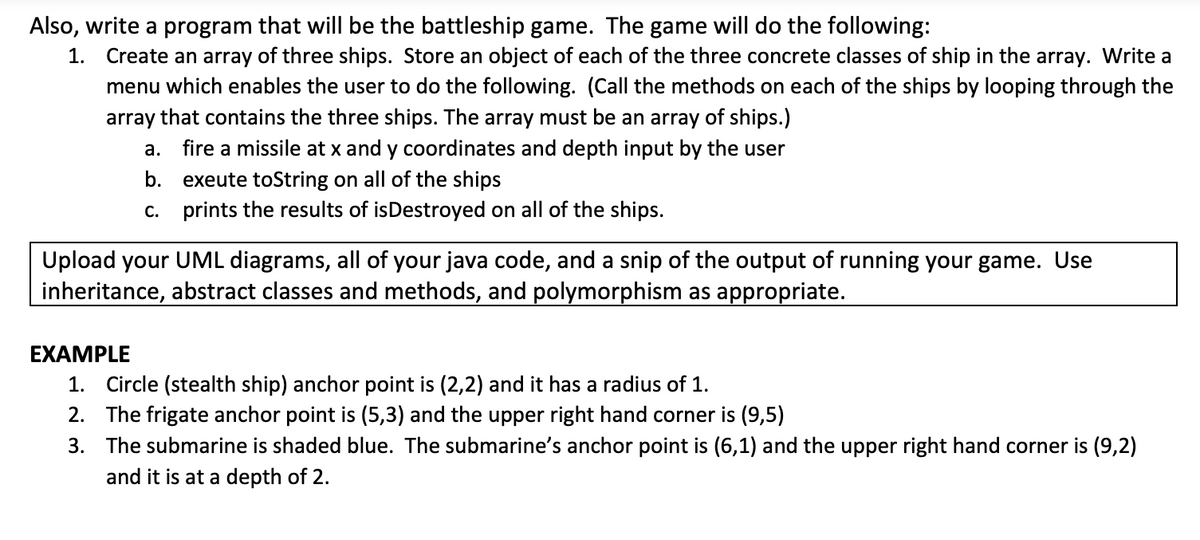
Transcribed Image Text:Also, write a program that will be the battleship game. The game will do the following:
1. Create an array of three ships. Store an object of each of the three concrete classes of ship in the array. Write a
menu which enables the user to do the following. (Call the methods on each of the ships by looping through the
array that contains the three ships. The array must be an array of ships.)
a. fire a missile at x and y coordinates and depth input by the user
b. exeute toString on all of the ships
c. prints the results of isDestroyed on all of the ships.
Upload your UML diagrams, all of your java code, and a snip of the output of running your game. Use
inheritance, abstract classes and methods, and polymorphism as appropriate.
EXAMPLE
1. Circle (stealth ship) anchor point is (2,2) and it has a radius of 1.
2.
The frigate anchor point is (5,3) and the upper right hand corner is (9,5)
3. The submarine is shaded blue. The submarine's anchor point is (6,1) and the upper right hand corner is (9,2)
and it is at a depth of 2.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 7 images

Recommended textbooks for you
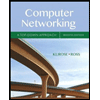
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
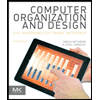
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
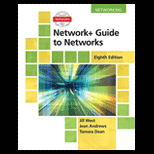
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
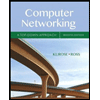
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
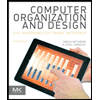
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
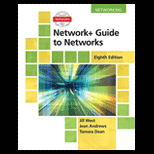
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
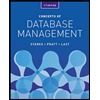
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
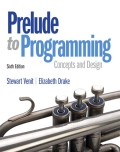
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
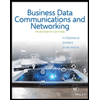
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY