Need python help. For problems 1 and 2, add your code to the file Lab2.java. Add your tests in the main function of Lab2.java. Do not use static variables in class Lab2 to implement recursive methods. Problem 1: Implement a recursive method min that accepts an array and returns the minimum element in the array. The recursive step should divide the array into two halves and find the minimum in each half. The program should run in O(n) time and consume O(logn) memory. Demonstrate the output of min on the array int [] a = { 2, 3, 5, 7, 11, 13, 17, 19, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97, 23, 29, 31, 37, 41, 43 } Problem 2 You have been offered a job that pays as follows: On the first day, you are paid 1 cent, on the second day, 2 cents, on the third day, 4 cents and so on. In other words, your pay doubles every day. Write a recursive method computePay that for a given day number computes the pay in cents. Assume that you accumulate all the money that you are paid. Write a recursive method computeSavings that computes the sum that you have accumulated on a given day. Show the output of computePay and computeSavings for day number 39. Java file (Lab2.java): public class Lab2 { public static void main(String[] arg) { // write your test code here } // Problem 1 public static int min(int [] a, int begin, int end) { } // Problem 2 public static long computePay(int day) { } public static long computeSavings(int day) { } }
Need python help.
For problems 1 and 2, add your code to the file Lab2.java. Add your tests in the main function of Lab2.java. Do not use static variables in class Lab2 to implement recursive methods.
Problem 1:
Implement a recursive method min that accepts an array and returns the minimum
element in the array. The recursive step should divide the array into two halves and find the minimum in each half. The program should run in O(n) time and consume O(logn) memory.
Demonstrate the output of min on the array
int [] a = { 2, 3, 5, 7, 11, 13, 17, 19, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97, 23, 29, 31,
37, 41, 43 }
Problem 2
You have been offered a job that pays as follows:
On the first day, you are paid 1 cent, on the second day, 2 cents, on the third day, 4 cents and so on. In other words, your pay doubles every day. Write a recursive method computePay that for a given day number computes the pay in cents.
Assume that you accumulate all the money that you are paid. Write a recursive method
computeSavings that computes the sum that you have accumulated on a given day.
Show the output of computePay and computeSavings for day number 39.
Java file (Lab2.java):
public class Lab2 {
public static void main(String[] arg) {
// write your test code here
}
// Problem 1
public static int min(int [] a, int begin, int end) {
}
// Problem 2
public static long computePay(int day) {
}
public static long computeSavings(int day) {
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

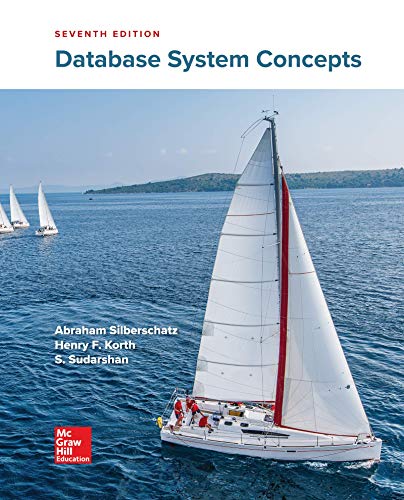
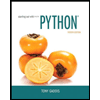
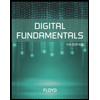
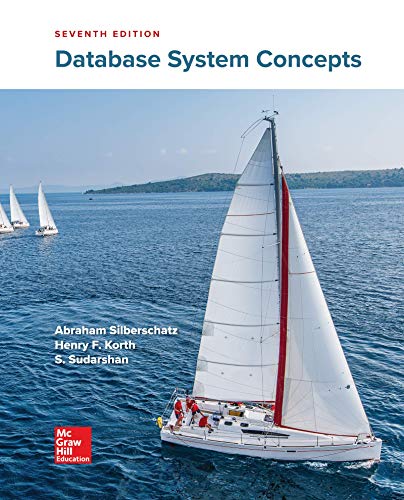
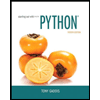
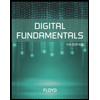
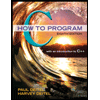
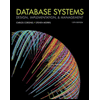
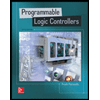