need the last line sorted in numerical order from greatest to least. 2 4 6 8 1 3 5 7 9 8 6 4 2 1 3 5 7 9 9 7 5 3 1 2 4 6 8 here's the code #include #include using namespace std; void print(vector v) { for (auto i : v) { cout << i << " "; } cout << endl; } void print_reverse(vector v) { for (int i = v.size() - 1; i >= 0; i--) { cout << v[i] << " "; } cout << endl; } string convert(string s) { for (int i = 0; i < s.size(); i++) { if ((s[i] >= 'A' && s[i] <= 'Z') || (s[i] >= 'a' && s[i] <= 'z')) continue; else s[i] = '!'; } return s; } string erase(string s) { string ans; for (int i = 0; i < s.length(); i++) { if (s[i] != '!') ans += s[i]; } return ans; } vector add(vectora, vectorb) { for (int i = 0; i < b.size(); i++) { a.insert(a.begin(), b[i]); } return a; } int main() { vector A; A.push_back(2); A.push_back(4); A.push_back(6); A.push_back(8); print(A); vector B = { 1,3,5,7,9 }; print(B); B = add(B, A); print(B); (B.begin(), B.end()); print_reverse(B); string C; cout << "Enter a string: "; cin >> C; C = convert(C); cout << C << endl; C = erase(C); cout << C; cout << endl; }
I need the last line sorted in numerical order from greatest to least.
2 4 6 8
1 3 5 7 9
8 6 4 2 1 3 5 7 9
9 7 5 3 1 2 4 6 8
here's the code
#include <iostream>
#include <
using namespace std;
void print(vector<int> v)
{
for (auto i : v)
{
cout << i << " ";
}
cout << endl;
}
void print_reverse(vector<int> v)
{
for (int i = v.size() - 1; i >= 0; i--)
{
cout << v[i] << " ";
}
cout << endl;
}
string convert(string s)
{
for (int i = 0; i < s.size(); i++)
{
if ((s[i] >= 'A' && s[i] <= 'Z') || (s[i] >= 'a' && s[i] <= 'z'))
continue;
else
s[i] = '!';
}
return s;
}
string erase(string s)
{
string ans;
for (int i = 0; i < s.length(); i++)
{
if (s[i] != '!')
ans += s[i];
}
return ans;
}
vector<int> add(vector<int>a, vector<int>b)
{
for (int i = 0; i < b.size(); i++)
{
a.insert(a.begin(), b[i]);
}
return a;
}
int main()
{
vector<int> A;
A.push_back(2);
A.push_back(4);
A.push_back(6);
A.push_back(8);
print(A);
vector<int> B = { 1,3,5,7,9 };
print(B);
B = add(B, A);
print(B);
(B.begin(), B.end());
print_reverse(B);
string C;
cout << "Enter a string: ";
cin >> C;
C = convert(C);
cout << C << endl;
C = erase(C);
cout << C;
cout << endl;
}

Step by step
Solved in 2 steps with 2 images

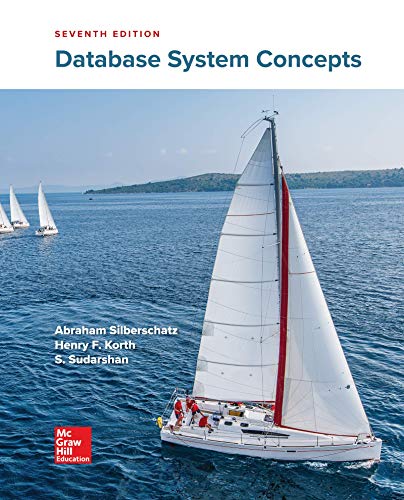
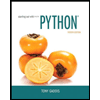
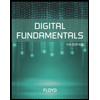
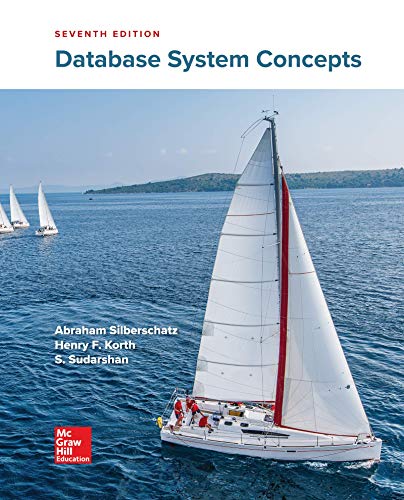
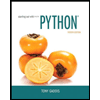
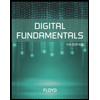
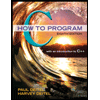
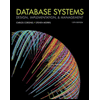
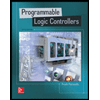