(Needs to write a java program. The imput file that the code will be tested on will be really large, but a code that can work on the provided sample in the picture should work just fine) An image is an array, or a matrix, of pixels (picture elements) arranged in columns and rows. RGB is one of the models used in color pixels. In a color image, each RGB pixel is an integer number which contains the mixture of red, green and blue colors. In this assignment, you will be provided with an image data file, image.dat. The first line in data file contains the height (rows) and width (columns) of the image. The following lines gives the red, green and blue color integer values for each pixel. The following sample.dat is given as an example: (The given example can be seen on the picture down) According to this input file, the image has 4 rows and 2 columns. The pixel at [0][0] has red value 117, green value 117 and blue value 245. Write a Java program that reads from the given input file and creates an RGB image object with the given width and height. Then, it'll read red, green and blue color values for each pixel from data file, and calculate RGB integer value using only bitwise shifting and masking operations. This is how you'll mix the colors and express the color pixel with one single integer. After generating RGB value for each pixel, your program will set the RGB value of each pixel with the computed RGB value. Your program will generate a .png image file as an output. Sample output: sample.png (The sample output can be seen on the picture down)
(Needs to write a java program. The imput file that the code will be tested on will be really large, but a code that can work on the provided sample in the picture should work just fine)
An image is an array, or a matrix, of pixels (picture elements) arranged in columns and rows.
RGB is one of the models used in color pixels. In a color image, each RGB pixel is an integer
number which contains the mixture of red, green and blue colors.
In this assignment, you will be provided with an image data file, image.dat. The first line in data
file contains the height (rows) and width (columns) of the image. The following lines gives the
red, green and blue color integer values for each pixel.
The following sample.dat is given as an example:
(The given example can be seen on the picture down)
According to this input file, the image has 4 rows and 2 columns. The pixel at [0][0] has red
value 117, green value 117 and blue value 245.
Write a Java program that reads from the given input file and creates an RGB image object with
the given width and height. Then, it'll read red, green and blue color values for each pixel from
data file, and calculate RGB integer value using only bitwise shifting and masking operations.
This is how you'll mix the colors and express the color pixel with one single integer. After
generating RGB value for each pixel, your program will set the RGB value of each pixel with the
computed RGB value. Your program will generate a .png image file as an output.
Sample output: sample.png
(The sample output can be seen on the picture down)
HINTS
• The following Java statement create an image object with the given height, width and image
type of RGB
BufferedImage canvas = new BufferedImage(width, height, BufferedImage.TYPE_INT_RGB);
• The following Java statement updates the RGB value of the pixel located at row y, and
column x of the image.
canvas.setRGB(x, y, rgb);
• The following Java statement created a .png file(image.png) from a BufferedImage object.
ImageIO.write(canvas,"PNG",new File("image.png"));
Here is an image of what a file might look like and the output.
![Page
2
of 5
ZOOM
+
Part Il: Image Representation
An image is an array, or a matrix, of pixels (picture elements) arranged in columns and rows.
RGB is one of the models used in color pixels. In a color image, each RGB pixel is an integer
number which contains the mixture of red, green and blue colors.
In this assignment, you will be provided with an image data file, image.dat. The first line in data
file contains the height (rows) and width (columns) of the image. The following lines gives the
red, green and blue color integer values for each pixel.
The following sample.dat is given as an example:
4 2
01110101,01110101,11110101 01110101,01010111,11110101
01110101,11010101,01010101 11010101,01010101,01010101
11000101,00011101,01110101 01100101,11110111,00100101
11110101,01110101,11010101 11110101,01111101,11110001
According to this input file, the image has 4 rows and 2 columns. The pixel at [0][0] has red
value 117, green value 117 and blue value 245.
Write a Java program that reads from the given input file and creates an RGB image object with
the given width and height. Then, it'll read red, green and blue color values for each pixel from
data file, and calculate RGB integer value using only bitwise shifting and masking operations.
This is how you'll mix the colors and express the color pixel with one single integer. After
generating RGB value for each pixel, your program will set the RGB value of each pixel with the
computed RGB value. Your program will generate a .png image file as an output.
Sample output: sample.png](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc6a237ce-c66f-40e5-b375-228a0d988793%2F20ab3396-afe6-4617-a2f0-db27662952b0%2Fdv0farp_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

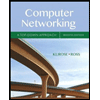
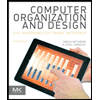
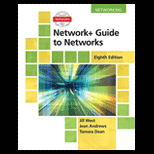
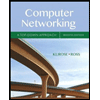
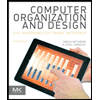
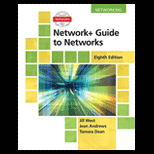
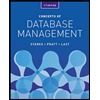
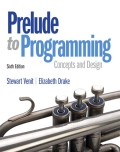
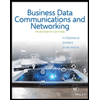