nip> // Needed for setw and others #include // Needed for ifstream #include // Needed for string data type #include // Needed for pow #include "Graph.h" // Used to graph t
Develop a program that takes a set of pairs of data values (e.g. sales and month), fits a straight line to the data, and displays the results in a table and chart. Fitting a line or equation to data is done in many fields including business and scientific research.
__________________________________________________________
#include <iostream> // Needed for cout and cin
#include <iomanip> // Needed for setw and others
#include <fstream> // Needed for ifstream
#include <string> // Needed for string data type
#include <cmath> // Needed for pow
#include "Graph.h" // Used to graph the results
using namespace std; // To use cout instead of std::cout
// Function prototypes
bool readArrays(ifstream& inFile, double x[], double y[], int size);
int main() {
// Do "Press return to continue..." on exit
atexit([] {system("pause"); });
// Prompt for the name of the data file and store it in a string variable named fileName.
// Put your code here
string fileName; // initializing string filename
cout << "Enter data file name: ";
cin >> fileName;
// Define a variable for the data file and open the file.
ifstream inFile(fileName);
// If the open failed, report an error/
if (inFile.fail()) {
cout << "Open of " << fileName << " failed." << endl;
return 1; // 1 means program failed.
}
// Read the title of the data set into a string variable named title.
// Put your code here
// Display the title.
// Put your code here
// Define an unsigned int variable named n. This will hold how many pairs are in the data file.
// Put your code here
// Read n from the file.
// Put your code here
// Allocate dynamic memory arrays of doubles named x and y for the x and y values.
// Put your code here
// Read the x and y values from the file.
if (!readArrays(inFile, x, y, n)) {
cout << "Failed to read data pairs from " << fileName << endl;
return 1;
};
// Close the file.
// Put your code here
// Compute the sums needed for the least squares computation.
double xSum = 0.0, ySum = 0.0, xySum = 0.0, x2Sum = 0.0;
for (unsigned i = 0; i < n; i++) {
// Put your code here
}
// Compute the slope a and y-intercept b of the line that fits the data.
// Put your code here
// Allocate an dynamic memory array of doubles named yFitted for the fitted values.
// Put your code here
// Compute fitted values corresponding to the x values.
// Put your code here
// Display the results in a tabular form.
cout << endl;
cout << setw(8) << "x" << setw(12) << "y(actual)" << setw(12) << "y(fitted)" << endl;
cout << setw(8) << "----" << setw(12) << "---------" << setw(12) << "---------" << endl;
for (unsigned i = 0; i < n; i++) {
// Put your code here
}
// Display the equation for the line.
cout << fixed << setprecision(5);
cout << "\nThe equation for the line is: y = " << m << "x + " << b << endl << endl;
// Display the results in a graph.
Graph graph;
graph.setWindowTitle(title.c_str());
graph.setPlot(Graph::PlotType::POINTS, "Actual", n, x, y);
graph.setPlot(Graph::PlotType::LINES, "Fitted", n, x, yFitted);
graph.mainLoop();
// Deallocate the arrays.
// Put your code here
return 0; // 0 means program worked
}
// Function definitions
// readArrays - Read the (x,y) pairs of data into x and y arrays from inFile.
// The parameter size is the number of pairs to read.
// Return false if unable to read size pairs from the file.
// Otherwise, return true.
bool readArrays(ifstream& inFile, double x[], double y[], int size) {
for (int i = 0; i < size; i++) {
// Read x
inFile >> x[i];
// Return false if that failed
if (!inFile) return false;
// Read y
inFile >> y[i];
// Return false if that failed
if (!inFile) return false;
}
// No failures so return true
return true;
}

Step by step
Solved in 2 steps

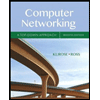
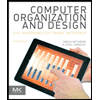
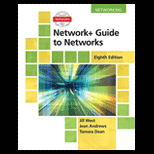
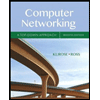
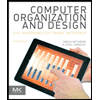
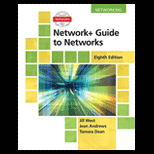
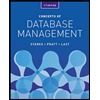
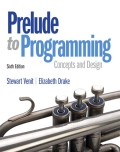
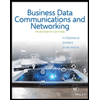