No array lists to be used /** * DO NOT MODIFY * reads the data for a football season from the filename provided. * * @param filename * @throws FileNotFoundException */ public Season(String league, String filename) throws FileNotFoundException { year = Integer.parseInt(filename.substring(0, 2)); File source = new File(league+"/"+filename); Scanner scanner = new Scanner(source); scanner.nextLine(); //ignore header int count = 0; while(scanner.hasNextLine()) { scanner.nextLine(); count++; } scanner.close(); matches = new Match[count]; scanner = new Scanner(source); scanner.nextLine(); //ignore header int i = 0; while(scanner.hasNextLine()) { String line = scanner.nextLine(); String[] tokens = line.split(","); Match m = new Match(tokens[1], tokens[2], Integer.parseInt(tokens[3]), Integer.parseInt(tokens[4])); matches[i++] = m; } scanner.close(); } /** * DO NOT MODIFY * @return */ public int size() { return matches.length; } /** * * @param idx * @return the match at given index (null if index invalid) */ public Match getMatch(int idx) { return new Match("Foo", "Bar", 0, 0); //dummy object returned. to be completed } /** * * @param idx * @return name of the winner of the match at passed index * return "We're all winners!" if the match at passed index ended in a draw. * return null if index is invalid */ public String getWinner(int idx) { return ""; //to be completed } /** * * @param team * @return number of games played at home by the team passed */ public int getHomeGameCount(String team) { return 0; //to be completed }
No array lists to be used
/**
* DO NOT MODIFY
* reads the data for a football season from the filename provided.
*
* @param filename
* @throws FileNotFoundException
*/
public Season(String league, String filename) throws FileNotFoundException {
year = Integer.parseInt(filename.substring(0, 2));
File source = new File(league+"/"+filename);
Scanner scanner = new Scanner(source);
scanner.nextLine(); //ignore header
int count = 0;
while(scanner.hasNextLine()) {
scanner.nextLine();
count++;
}
scanner.close();
matches = new Match[count];
scanner = new Scanner(source);
scanner.nextLine(); //ignore header
int i = 0;
while(scanner.hasNextLine()) {
String line = scanner.nextLine();
String[] tokens = line.split(",");
Match m = new Match(tokens[1], tokens[2], Integer.parseInt(tokens[3]), Integer.parseInt(tokens[4]));
matches[i++] = m;
}
scanner.close();
}
/**
* DO NOT MODIFY
* @return
*/
public int size() {
return matches.length;
}
/**
*
* @param idx
* @return the match at given index (null if index invalid)
*/
public Match getMatch(int idx) {
return new Match("Foo", "Bar", 0, 0); //dummy object returned. to be completed
}
/**
*
* @param idx
* @return name of the winner of the match at passed index
* return "We're all winners!" if the match at passed index ended in a draw.
* return null if index is invalid
*/
public String getWinner(int idx) {
return ""; //to be completed
}
/**
*
* @param team
* @return number of games played at home by the team passed
*/
public int getHomeGameCount(String team) {
return 0; //to be completed
}

Step by step
Solved in 4 steps

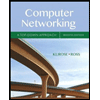
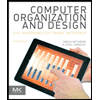
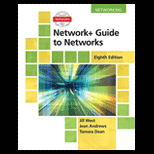
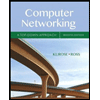
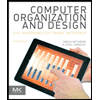
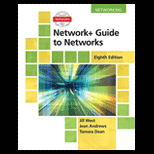
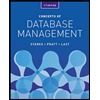
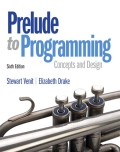
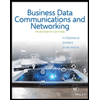