Notes: -The maximum size of any c-string in this lab is 100 characters and it should be dynamically allocated in the constructor and de-allocated in the destructor. -For Visual Studio you need to put the following line as first top line in your source code file: #define _CRT_SECURE_NO_WARNINGS Question 1: The class Person contains the following properties and functions: -Name (e-string), private -Age (int), private -Address (e-string), private -Constructors (default and non-default) -Getters and Setters -Destructor: to free the memory and print the message "Object with name [PERSON_NAME] is dead" where PERSON_NAME is the person name for the object being disposed. -Print function to print the details of the employee. Test your class with the following main: int main() Person per1; Person per2("Ahmad", "AUS Campus", 21); cout << "Person perl :\n"; perl.print(); cout << "\nPerson per2 :\n"; per2.print(); cout<<"\nTesting the setters functions: \nSetting perl name to Johnny English, address to UK and age to 54\n"; per1.setName("Johnny English"); per1.setAddress("UK"); per1.setAge(54); cout<<"Testing the getters :\n";
Notes: -The maximum size of any c-string in this lab is 100 characters and it should be dynamically allocated in the constructor and de-allocated in the destructor. -For Visual Studio you need to put the following line as first top line in your source code file: #define _CRT_SECURE_NO_WARNINGS Question 1: The class Person contains the following properties and functions: -Name (e-string), private -Age (int), private -Address (e-string), private -Constructors (default and non-default) -Getters and Setters -Destructor: to free the memory and print the message "Object with name [PERSON_NAME] is dead" where PERSON_NAME is the person name for the object being disposed. -Print function to print the details of the employee. Test your class with the following main: int main() Person per1; Person per2("Ahmad", "AUS Campus", 21); cout << "Person perl :\n"; perl.print(); cout << "\nPerson per2 :\n"; per2.print(); cout<<"\nTesting the setters functions: \nSetting perl name to Johnny English, address to UK and age to 54\n"; per1.setName("Johnny English"); per1.setAddress("UK"); per1.setAge(54); cout<<"Testing the getters :\n";
Chapter3: Using Methods, Classes, And Objects
Section: Chapter Questions
Problem 1GZ
Related questions
Question
![per1.setAge(54);
cout<<"Testing the getters :\n";
cout<<"perl name is "
<<per1.getName() <<endl;
cout<<"perl address is "
<<per1.getAddress()<<endl;
cout<<"perl age is "<<per1.getAge()
<<endl;
return 0;
}
You should get the following output:
Person peri
Name [None] Age [0] Address [None]
Person per2:
Name [Ahmad] Age [21] Address [AUS Campus]
Testing the setters functions:
Setting peri name to Johnny English, address to UK and age to 54
Testing the getters:
perl name is Johnny English
perl address is UK
peri age is 54
Object is with name [Ahmad] is dead
Object is with name [Johnny English] is dead](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2f9c2004-8dba-4b84-bd4a-da630aeddd4b%2F393fde2a-691f-4298-b95d-ea62cd32d73b%2Fuamilmq_processed.png&w=3840&q=75)
Transcribed Image Text:per1.setAge(54);
cout<<"Testing the getters :\n";
cout<<"perl name is "
<<per1.getName() <<endl;
cout<<"perl address is "
<<per1.getAddress()<<endl;
cout<<"perl age is "<<per1.getAge()
<<endl;
return 0;
}
You should get the following output:
Person peri
Name [None] Age [0] Address [None]
Person per2:
Name [Ahmad] Age [21] Address [AUS Campus]
Testing the setters functions:
Setting peri name to Johnny English, address to UK and age to 54
Testing the getters:
perl name is Johnny English
perl address is UK
peri age is 54
Object is with name [Ahmad] is dead
Object is with name [Johnny English] is dead
![Notes:
The maximum size of any c-string in this lab is 100
characters and it should be dynamically allocated in
the constructor and de-allocated in the destructor.
For Visual Studio you need to put the following line
as first top line in your source code file:
#define _CRT_SECURE_NO_WARNINGS
Question 1:
The class Person contains the following properties and
functions:
-Name (c-string), private.
-Age (int), private
- Address (c-string), private
- Constructors (default and non-default)
-Getters and Setters
-Destructor: to free the memory and print the message
"Object with name [PERSON_NAME] is dead"
where PERSON_NAME is the person name for the
object being disposed.
Print function to print the details of the employee.
Test your class with the following main:
int main()
{
Person per1;
Person per2("Ahmad", "AUS Campus",
21);
cout << "Person per1 :\n";
per1.print();
cout << "\nPerson per2 :\n";
per2.print();
cout<<"\nTesting the setters
functions: \nSetting perl name to
Johnny English, address to UK and age
to 54\n";
per1.setName("Johnny English");
per1.setAddress("UK");
per1.setAge(54);
cout<<"Testing the getters :\n";
cout<<"perl name is
<<per1.getName() <<endl;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2f9c2004-8dba-4b84-bd4a-da630aeddd4b%2F393fde2a-691f-4298-b95d-ea62cd32d73b%2Ffpdyakm_processed.png&w=3840&q=75)
Transcribed Image Text:Notes:
The maximum size of any c-string in this lab is 100
characters and it should be dynamically allocated in
the constructor and de-allocated in the destructor.
For Visual Studio you need to put the following line
as first top line in your source code file:
#define _CRT_SECURE_NO_WARNINGS
Question 1:
The class Person contains the following properties and
functions:
-Name (c-string), private.
-Age (int), private
- Address (c-string), private
- Constructors (default and non-default)
-Getters and Setters
-Destructor: to free the memory and print the message
"Object with name [PERSON_NAME] is dead"
where PERSON_NAME is the person name for the
object being disposed.
Print function to print the details of the employee.
Test your class with the following main:
int main()
{
Person per1;
Person per2("Ahmad", "AUS Campus",
21);
cout << "Person per1 :\n";
per1.print();
cout << "\nPerson per2 :\n";
per2.print();
cout<<"\nTesting the setters
functions: \nSetting perl name to
Johnny English, address to UK and age
to 54\n";
per1.setName("Johnny English");
per1.setAddress("UK");
per1.setAge(54);
cout<<"Testing the getters :\n";
cout<<"perl name is
<<per1.getName() <<endl;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
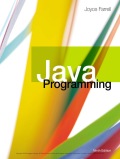
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
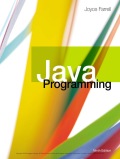
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT